10 Best C++ Projects for Beginners
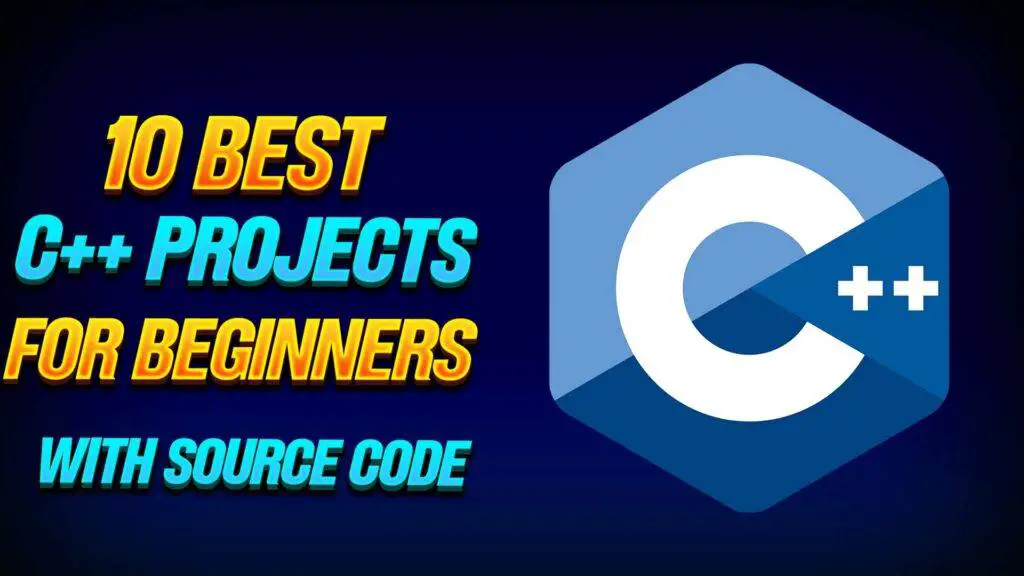
Are you a beginner looking to sharpen your skills in C++? Look no further! In this article, we’ll explore 10 C++ projects for beginners that help you learn the fundamentals of the language and introduce you to the world of software development. The source code is also available to help beginners.
10 Best C++ Projects for Beginners with Source Code
1.Book Shop Management System in C++
You can build a management system for a bookshop. With C++, you can create a complete management system that handles inventory, sales tracking, and customer information tasks. By executing concepts like classes, objects, and file handling, you’ll gain a deeper understanding of C++ fundamentals while developing a practical solution for real-world businesses.
Source Code for Book Shop Management System in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a book
struct Book {
string title;
string author;
int quantity;
double price;
};
// Function to add a book to the inventory
void addBook(vector<Book>& inventory) {
Book newBook;
cout << "Enter book title: ";
getline(cin >> ws, newBook.title); // Using getline to handle spaces in book titles
cout << "Enter author name: ";
getline(cin >> ws, newBook.author);
cout << "Enter quantity: ";
cin >> newBook.quantity;
cout << "Enter price: ";
cin >> newBook.price;
inventory.push_back(newBook);
cout << "Book added successfully!" << endl;
}
// Function to display all books in the inventory
void displayBooks(const vector<Book>& inventory) {
cout << "Inventory:" << endl;
for (const auto& book : inventory) {
cout << "Title: " << book.title << ", Author: " << book.author << ", Quantity: " << book.quantity << ", Price: $" << book.price << endl;
}
}
// Function to search for a book by title
void searchBook(const vector<Book>& inventory, const string& title) {
bool found = false;
for (const auto& book : inventory) {
if (book.title == title) {
cout << "Book found:" << endl;
cout << "Title: " << book.title << ", Author: " << book.author << ", Quantity: " << book.quantity << ", Price: $" << book.price << endl;
found = true;
break;
}
}
if (!found) {
cout << "Book not found." << endl;
}
}
int main() {
vector<Book> inventory;
int choice;
string searchTitle;
do {
cout << "\nBookshop Management System\n";
cout << "1. Add a book\n";
cout << "2. Display all books\n";
cout << "3. Search for a book by title\n";
cout << "4. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1:
cin.ignore(); // Ignore the newline character left by cin
addBook(inventory);
break;
case 2:
displayBooks(inventory);
break;
case 3:
cout << "Enter book title to search: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin >> ws, searchTitle);
searchBook(inventory, searchTitle);
break;
case 4:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 4." << endl;
}
} while (choice != 4);
return 0;
}
2. Banking Management system
The banking management system can be a good C++ project for beginners. This project lets you simulate essential banking operations like account creation, deposits, withdrawals, and balance inquiries. Not only does this project enhance your C++ proficiency, but it also introduces you to the importance of error handling and exception management in software development.
Source Code for Banking Management system in C++
#include <iostream>
#include <vector>
#include <iomanip>
using namespace std;
// Define a structure to represent an account
struct Account {
int accountNumber;
string accountHolderName;
double balance;
};
// Function to create a new account
void createAccount(vector<Account>& accounts) {
Account newAccount;
cout << "Enter account number: ";
cin >> newAccount.accountNumber;
cout << "Enter account holder name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newAccount.accountHolderName);
cout << "Enter initial balance: ";
cin >> newAccount.balance;
accounts.push_back(newAccount);
cout << "Account created successfully!" << endl;
}
// Function to display all accounts
void displayAccounts(const vector<Account>& accounts) {
cout << "\nBank Accounts:" << endl;
cout << "--------------------------------------------------" << endl;
cout << setw(15) << "Account No." << setw(25) << "Account Holder Name" << setw(15) << "Balance" << endl;
cout << "--------------------------------------------------" << endl;
for (const auto& account : accounts) {
cout << setw(15) << account.accountNumber << setw(25) << account.accountHolderName << setw(15) << fixed << setprecision(2) << account.balance << endl;
}
cout << "--------------------------------------------------" << endl;
}
// Function to deposit money into an account
void deposit(vector<Account>& accounts, int accountNumber, double amount) {
for (auto& account : accounts) {
if (account.accountNumber == accountNumber) {
account.balance += amount;
cout << "Deposit successful. New balance: " << fixed << setprecision(2) << account.balance << endl;
return;
}
}
cout << "Account not found." << endl;
}
// Function to withdraw money from an account
void withdraw(vector<Account>& accounts, int accountNumber, double amount) {
for (auto& account : accounts) {
if (account.accountNumber == accountNumber) {
if (account.balance >= amount) {
account.balance -= amount;
cout << "Withdrawal successful. New balance: " << fixed << setprecision(2) << account.balance << endl;
} else {
cout << "Insufficient funds." << endl;
}
return;
}
}
cout << "Account not found." << endl;
}
int main() {
vector<Account> accounts;
int choice, accountNumber;
double amount;
do {
cout << "\nBanking Management System\n";
cout << "1. Create Account\n";
cout << "2. Display Accounts\n";
cout << "3. Deposit\n";
cout << "4. Withdraw\n";
cout << "5. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
createAccount(accounts);
break;
case 2:
displayAccounts(accounts);
break;
case 3:
cout << "Enter account number: ";
cin >> accountNumber;
cout << "Enter amount to deposit: ";
cin >> amount;
deposit(accounts, accountNumber, amount);
break;
case 4:
cout << "Enter account number: ";
cin >> accountNumber;
cout << "Enter amount to withdraw: ";
cin >> amount;
withdraw(accounts, accountNumber, amount);
break;
case 5:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 5." << endl;
}
} while (choice != 5);
return 0;
}
3. Airline Reservation System in C++
This project allows you to precisely create the booking process, seat allocation, and flight schedules. Implement data structures like arrays and linked lists to manage passenger information efficiently. You’ll learn to build scalable solutions that cater to complex business requirements by incorporating concepts such as dynamic memory allocation and modular programming.
Source Code for Airline Reservation System in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a flight
struct Flight {
string flightNumber;
string origin;
string destination;
int capacity;
int bookedSeats;
};
// Function to display flight details
void displayFlightDetails(const Flight& flight) {
cout << "Flight Number: " << flight.flightNumber << endl;
cout << "Origin: " << flight.origin << endl;
cout << "Destination: " << flight.destination << endl;
cout << "Capacity: " << flight.capacity << endl;
cout << "Booked Seats: " << flight.bookedSeats << endl;
}
// Function to check seat availability and book a seat
void bookSeat(Flight& flight) {
if (flight.bookedSeats < flight.capacity) {
flight.bookedSeats++;
cout << "Seat booked successfully!" << endl;
} else {
cout << "Sorry, the flight is full. No seats available." << endl;
}
}
int main() {
// Create some sample flights
vector<Flight> flights = {
{"F101", "New York", "Los Angeles", 200, 0},
{"F102", "Los Angeles", "Chicago", 150, 0},
{"F103", "Chicago", "Houston", 180, 0}
};
int choice, flightIndex;
do {
cout << "\nAirline Reservation System\n";
cout << "1. Display Flight Details\n";
cout << "2. Book a Seat\n";
cout << "3. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "\nFlight Details\n";
cout << "-----------------------------------" << endl;
for (size_t i = 0; i < flights.size(); ++i) {
cout << i + 1 << ". ";
displayFlightDetails(flights[i]);
cout << "-----------------------------------" << endl;
}
break;
case 2:
cout << "Enter the index of the flight to book a seat: ";
cin >> flightIndex;
if (flightIndex >= 1 && flightIndex <= flights.size()) {
bookSeat(flights[flightIndex - 1]);
} else {
cout << "Invalid flight index." << endl;
}
break;
case 3:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 3." << endl;
}
} while (choice != 3);
return 0;
}
4. Hospital Management System in C++
In this project, you will create a system for patients, doctors, medicines, and appointment management. Through this project, you not only hone your C++ skills but also contribute to enhancing efficiency in healthcare service delivery.
Source Code for Hospital Management system in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a patient
struct Patient {
string name;
int age;
string gender;
string illness;
};
// Define a structure to represent a doctor
struct Doctor {
string name;
string specialization;
};
// Define a structure to represent a hospital
struct Hospital {
string name;
vector<Doctor> doctors;
vector<Patient> patients;
};
// Function to add a new patient to the hospital
void addPatient(Hospital& hospital) {
Patient newPatient;
cout << "Enter patient name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newPatient.name);
cout << "Enter patient age: ";
cin >> newPatient.age;
cout << "Enter patient gender: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newPatient.gender);
cout << "Enter patient illness: ";
getline(cin, newPatient.illness);
hospital.patients.push_back(newPatient);
cout << "Patient added successfully!" << endl;
}
// Function to add a new doctor to the hospital
void addDoctor(Hospital& hospital) {
Doctor newDoctor;
cout << "Enter doctor name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newDoctor.name);
cout << "Enter doctor's specialization: ";
getline(cin, newDoctor.specialization);
hospital.doctors.push_back(newDoctor);
cout << "Doctor added successfully!" << endl;
}
// Function to display all patients in the hospital
void displayPatients(const Hospital& hospital) {
cout << "\nPatients:" << endl;
cout << "-----------------------------------" << endl;
for (const auto& patient : hospital.patients) {
cout << "Name: " << patient.name << ", Age: " << patient.age << ", Gender: " << patient.gender << ", Illness: " << patient.illness << endl;
}
cout << "-----------------------------------" << endl;
}
// Function to display all doctors in the hospital
void displayDoctors(const Hospital& hospital) {
cout << "\nDoctors:" << endl;
cout << "-----------------------------------" << endl;
for (const auto& doctor : hospital.doctors) {
cout << "Name: " << doctor.name << ", Specialization: " << doctor.specialization << endl;
}
cout << "-----------------------------------" << endl;
}
int main() {
Hospital hospital;
hospital.name = "ABC Hospital";
int choice;
do {
cout << "\nHospital Management System\n";
cout << "1. Add Patient\n";
cout << "2. Add Doctor\n";
cout << "3. Display Patients\n";
cout << "4. Display Doctors\n";
cout << "5. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addPatient(hospital);
break;
case 2:
addDoctor(hospital);
break;
case 3:
displayPatients(hospital);
break;
case 4:
displayDoctors(hospital);
break;
case 5:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 5." << endl;
}
} while (choice != 5);
return 0;
}
5. Library Management System in C++
Library Management system empowers you to automate library operations such as book cataloging, issue tracking, and fine calculation. Including graphical user interfaces (GUIs) using libraries like Qt or wxWidgets will boost the user experience and showcase your creativity as a developer.
Source Code for Library Management system
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a book
struct Book {
string title;
string author;
int id;
bool isAvailable;
};
// Define a structure to represent a member
struct Member {
string name;
int id;
vector<Book*> borrowedBooks;
};
// Function to add a new book to the library
void addBook(vector<Book>& library) {
Book newBook;
cout << "Enter book title: ";
getline(cin >> ws, newBook.title);
cout << "Enter author name: ";
getline(cin >> ws, newBook.author);
cout << "Enter book ID: ";
cin >> newBook.id;
newBook.isAvailable = true;
library.push_back(newBook);
cout << "Book added successfully!" << endl;
}
// Function to lend a book to a member
void lendBook(vector<Book>& library, vector<Member>& members) {
int memberId, bookId;
cout << "Enter member ID: ";
cin >> memberId;
cout << "Enter book ID: ";
cin >> bookId;
// Search for the member
Member* member = nullptr;
for (auto& m : members) {
if (m.id == memberId) {
member = &m;
break;
}
}
if (!member) {
cout << "Member not found." << endl;
return;
}
// Search for the book
Book* book = nullptr;
for (auto& b : library) {
if (b.id == bookId) {
book = &b;
break;
}
}
if (!book) {
cout << "Book not found." << endl;
return;
}
if (book->isAvailable) {
book->isAvailable = false;
member->borrowedBooks.push_back(book);
cout << "Book successfully lent to " << member->name << "." << endl;
} else {
cout << "Book is already lent out." << endl;
}
}
// Function to display all books in the library
void displayBooks(const vector<Book>& library) {
cout << "Library Books:" << endl;
for (const auto& book : library) {
cout << "Title: " << book.title << ", Author: " << book.author << ", ID: " << book.id;
if (book.isAvailable) {
cout << " (Available)" << endl;
} else {
cout << " (Not Available)" << endl;
}
}
}
int main() {
vector<Book> library;
vector<Member> members;
int choice;
do {
cout << "\nLibrary Management System\n";
cout << "1. Add Book\n";
cout << "2. Lend Book\n";
cout << "3. Display Books\n";
cout << "4. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cin.ignore(); // Ignore the newline character left by cin
addBook(library);
break;
case 2:
lendBook(library, members);
break;
case 3:
displayBooks(library);
break;
case 4:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 4." << endl;
}
} while (choice != 4);
return 0;
}
6. School Management System in C++
School Management System in C++ is also a good idea for beginners. This project involves designing functions for student enrollment, attendance tracking, and grade management. Learn object-oriented programming principles to model real-world entities such as students, teachers, and courses. Through this project, you’ll refine your C++ proficiency and gain an understanding of the complexities of school management.
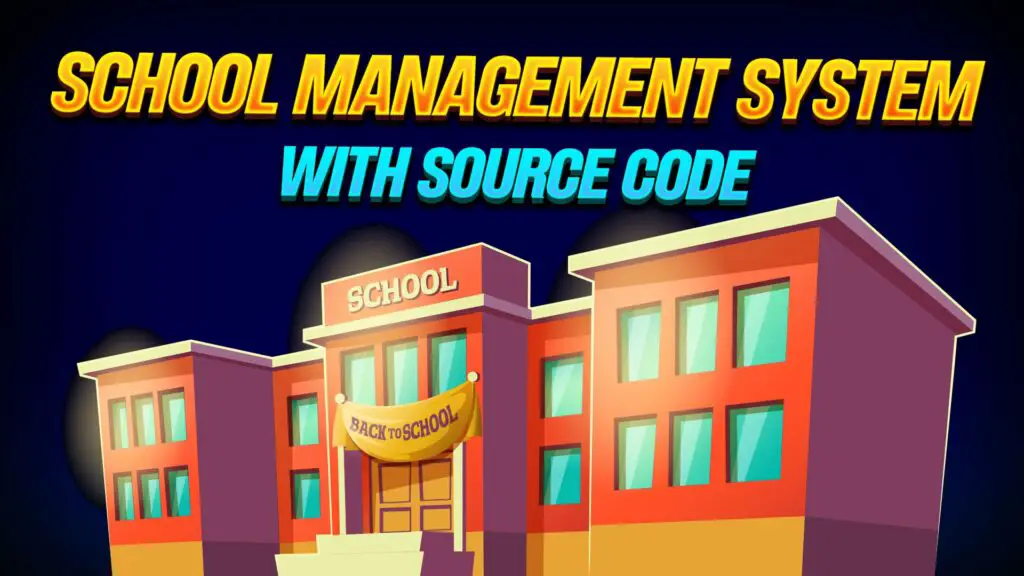
Source Code for School Management system in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a student
struct Student {
string name;
int age;
string grade;
string address;
};
// Define a structure to represent a teacher
struct Teacher {
string name;
string subject;
};
// Define a structure to represent a school
struct School {
string name;
vector<Student> students;
vector<Teacher> teachers;
};
// Function to add a new student to the school
void addStudent(School& school) {
Student newStudent;
cout << "Enter student name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newStudent.name);
cout << "Enter student age: ";
cin >> newStudent.age;
cout << "Enter student grade: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newStudent.grade);
cout << "Enter student address: ";
getline(cin, newStudent.address);
school.students.push_back(newStudent);
cout << "Student added successfully!" << endl;
}
// Function to add a new teacher to the school
void addTeacher(School& school) {
Teacher newTeacher;
cout << "Enter teacher name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newTeacher.name);
cout << "Enter teacher subject: ";
getline(cin, newTeacher.subject);
school.teachers.push_back(newTeacher);
cout << "Teacher added successfully!" << endl;
}
// Function to display all students in the school
void displayStudents(const School& school) {
cout << "\nStudents:" << endl;
cout << "-----------------------------------" << endl;
for (const auto& student : school.students) {
cout << "Name: " << student.name << ", Age: " << student.age << ", Grade: " << student.grade << ", Address: " << student.address << endl;
}
cout << "-----------------------------------" << endl;
}
// Function to display all teachers in the school
void displayTeachers(const School& school) {
cout << "\nTeachers:" << endl;
cout << "-----------------------------------" << endl;
for (const auto& teacher : school.teachers) {
cout << "Name: " << teacher.name << ", Subject: " << teacher.subject << endl;
}
cout << "-----------------------------------" << endl;
}
int main() {
School school;
school.name = "XYZ School";
int choice;
do {
cout << "\nSchool Management System\n";
cout << "1. Add Student\n";
cout << "2. Add Teacher\n";
cout << "3. Display Students\n";
cout << "4. Display Teachers\n";
cout << "5. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addStudent(school);
break;
case 2:
addTeacher(school);
break;
case 3:
displayStudents(school);
break;
case 4:
displayTeachers(school);
break;
case 5:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 5." << endl;
}
} while (choice != 5);
return 0;
}
7. Employee Management System
This one is the most in-demand project for the business. This project enables you to simplify HR processes such as employee hiring, payroll management, and performance evaluation. You’ll learn to integrate C++ applications with backend systems by including database connectivity using frameworks like SQLite or MySQL.
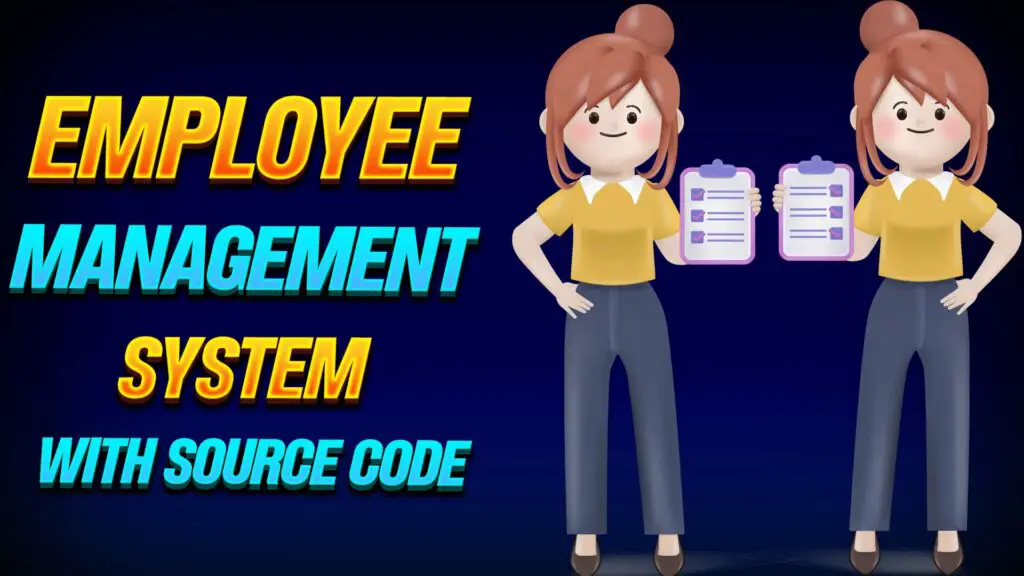
Source Code For employee management system in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent an employee
struct Employee {
string name;
int age;
string department;
double salary;
};
// Function to add a new employee to the system
void addEmployee(vector<Employee>& employees) {
Employee newEmployee;
cout << "Enter employee name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newEmployee.name);
cout << "Enter employee age: ";
cin >> newEmployee.age;
cout << "Enter employee department: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newEmployee.department);
cout << "Enter employee salary: ";
cin >> newEmployee.salary;
employees.push_back(newEmployee);
cout << "Employee added successfully!" << endl;
}
// Function to display all employees in the system
void displayEmployees(const vector<Employee>& employees) {
cout << "\nEmployees:" << endl;
cout << "-----------------------------------" << endl;
for (const auto& employee : employees) {
cout << "Name: " << employee.name << ", Age: " << employee.age << ", Department: " << employee.department << ", Salary: $" << employee.salary << endl;
}
cout << "-----------------------------------" << endl;
}
int main() {
vector<Employee> employees;
int choice;
do {
cout << "\nEmployee Management System\n";
cout << "1. Add Employee\n";
cout << "2. Display Employees\n";
cout << "3. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addEmployee(employees);
break;
case 2:
displayEmployees(employees);
break;
case 3:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 3." << endl;
}
} while (choice != 3);
return 0;
}
8. Inventory Management System in C++
Learn the art of supply chain management with a C++ inventory management system. This project includes building modules for stock tracking, order processing, and vendor management.
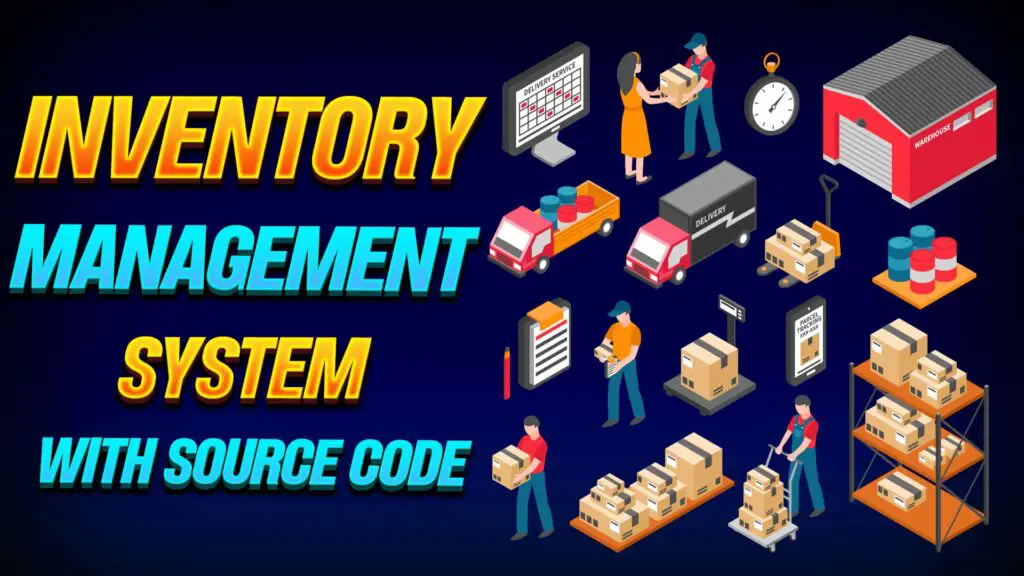
Source Code for Inventory Management System in C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a structure to represent a product
struct Product {
string name;
double price;
int quantity;
};
// Function to add a new product to the inventory
void addProduct(vector<Product>& inventory) {
Product newProduct;
cout << "Enter product name: ";
cin.ignore(); // Ignore the newline character left by cin
getline(cin, newProduct.name);
cout << "Enter product price: ";
cin >> newProduct.price;
cout << "Enter product quantity: ";
cin >> newProduct.quantity;
inventory.push_back(newProduct);
cout << "Product added successfully!" << endl;
}
// Function to display all products in the inventory
void displayInventory(const vector<Product>& inventory) {
cout << "\nInventory:" << endl;
cout << "-----------------------------------" << endl;
cout << "Name\t\tPrice\t\tQuantity" << endl;
for (const auto& product : inventory) {
cout << product.name << "\t\t$" << product.price << "\t\t" << product.quantity << endl;
}
cout << "-----------------------------------" << endl;
}
int main() {
vector<Product> inventory;
int choice;
do {
cout << "\nInventory Management System\n";
cout << "1. Add Product\n";
cout << "2. Display Inventory\n";
cout << "3. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addProduct(inventory);
break;
case 2:
displayInventory(inventory);
break;
case 3:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 3." << endl;
}
} while (choice != 3);
return 0;
}
9. Task Management System in C++
Boost your productivity by creating a task management system using C++. This project includes organizing tasks, setting deadlines, and tracking progress effectively. Combining user-friendly interfaces and notifications will create a seamless user experience that enhances workflow efficiency.
Source Code for Task Management system in C++
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
// Define a structure to represent a task
struct Task {
string name;
string description;
bool completed;
};
// Function to add a new task to the task list
void addTask(vector<Task>& tasks) {
Task newTask;
cout << "Enter task name: ";
getline(cin >> ws, newTask.name);
cout << "Enter task description: ";
getline(cin >> ws, newTask.description);
newTask.completed = false;
tasks.push_back(newTask);
cout << "Task added successfully!" << endl;
}
// Function to display all tasks in the task list
void displayTasks(const vector<Task>& tasks) {
if (tasks.empty()) {
cout << "Task list is empty." << endl;
return;
}
cout << "\nTasks:" << endl;
cout << "---------------------------------------------------------" << endl;
for (size_t i = 0; i < tasks.size(); ++i) {
cout << i + 1 << ". ";
cout << "Name: " << tasks[i].name << ", Description: " << tasks[i].description;
if (tasks[i].completed) {
cout << " [Completed]";
} else {
cout << " [Pending]";
}
cout << endl;
}
cout << "---------------------------------------------------------" << endl;
}
// Function to mark a task as completed
void markTaskCompleted(vector<Task>& tasks, int index) {
if (index >= 1 && index <= static_cast<int>(tasks.size())) {
tasks[index - 1].completed = true;
cout << "Task marked as completed." << endl;
} else {
cout << "Invalid task index." << endl;
}
}
int main() {
vector<Task> tasks;
int choice;
int taskIndex;
do {
cout << "\nTask Management System\n";
cout << "1. Add Task\n";
cout << "2. Display Tasks\n";
cout << "3. Mark Task as Completed\n";
cout << "4. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cin.ignore(); // Ignore the newline character left by cin
addTask(tasks);
break;
case 2:
displayTasks(tasks);
break;
case 3:
cout << "Enter the index of the task to mark as completed: ";
cin >> taskIndex;
markTaskCompleted(tasks, taskIndex);
break;
case 4:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 4." << endl;
}
} while (choice != 4);
return 0;
}
10. Project Management System in C++
This ambitious project involves producing tasks, allocating resources, and monitoring project progress in real-time. Integrating features like Gantt charts and burndown charts will give stakeholders valuable insights into project dynamics and timelines.
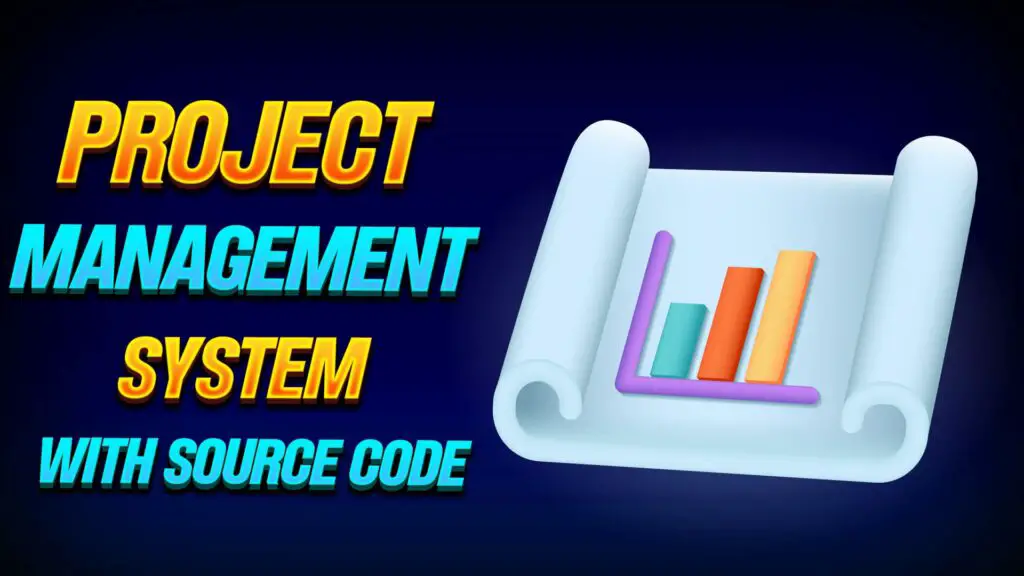
Source Code for Project Management system in C++
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
// Define a structure to represent a task
struct Task {
string name;
string description;
bool completed;
};
// Function to add a new task to the task list
void addTask(vector<Task>& tasks) {
Task newTask;
cout << "Enter task name: ";
getline(cin >> ws, newTask.name);
cout << "Enter task description: ";
getline(cin >> ws, newTask.description);
newTask.completed = false;
tasks.push_back(newTask);
cout << "Task added successfully!" << endl;
}
// Function to display all tasks in the task list
void displayTasks(const vector<Task>& tasks) {
if (tasks.empty()) {
cout << "Task list is empty." << endl;
return;
}
cout << "\nTasks:" << endl;
cout << "---------------------------------------------------------" << endl;
for (size_t i = 0; i < tasks.size(); ++i) {
cout << i + 1 << ". ";
cout << "Name: " << tasks[i].name << ", Description: " << tasks[i].description;
if (tasks[i].completed) {
cout << " [Completed]";
} else {
cout << " [Pending]";
}
cout << endl;
}
cout << "---------------------------------------------------------" << endl;
}
// Function to mark a task as completed
void markTaskCompleted(vector<Task>& tasks, int index) {
if (index >= 1 && index <= static_cast<int>(tasks.size())) {
tasks[index - 1].completed = true;
cout << "Task marked as completed." << endl;
} else {
cout << "Invalid task index." << endl;
}
}
int main() {
vector<Task> tasks;
int choice;
int taskIndex;
do {
cout << "\nTask Management System\n";
cout << "1. Add Task\n";
cout << "2. Display Tasks\n";
cout << "3. Mark Task as Completed\n";
cout << "4. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cin.ignore(); // Ignore the newline character left by cin
addTask(tasks);
break;
case 2:
displayTasks(tasks);
break;
case 3:
cout << "Enter the index of the task to mark as completed: ";
cin >> taskIndex;
markTaskCompleted(tasks, taskIndex);
break;
case 4:
cout << "Exiting...";
break;
default:
cout << "Invalid choice. Please enter a number from 1 to 4." << endl;
}
} while (choice != 4);
return 0;
}
To Learn More:
Basic understanding of C++ syntax and concepts like variables, loops, and functions is recommended. Additionally, familiarity with object-oriented programming principles will be beneficial.
While some projects may require prior programming experience, many of them are beginner-friendly and designed to help newcomers grasp fundamental concepts while building practical applications.
Absolutely! These projects serve as templates that you can adapt and expand according to your needs. Feel free to add new features, modify existing functionalities, or tailor the projects to suit specific industries or use cases.
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
Realtime Object Detection
-
10 Advance Final Year Project Ideas with Source Code
-
E Commerce sales forecasting using machine learning
-
AI Music Composer project with source code
-
Stock market Price Prediction using machine learning
-
c++ Projects for beginners
-
30 Final Year Project Ideas for IT Students
-
Python Projects For Final Year Students With Source Code
-
Top 10 Best JAVA Final Year Projects
-
C++ Projects with Source Code
-
How to Host HTML website for free?
-
20 Exiciting Cyber Security Final Year Projects
-
How to Download image in HTML
-
Artificial Intelligence Projects For Final Year
-
Hate Speech Detection Using Machine Learning
-
10 Web Development Projects for beginners
-
Fake news detection using machine learning source code
-
Credit Card Fraud detection using machine learning