10 Unique C++ Projects with Source Code
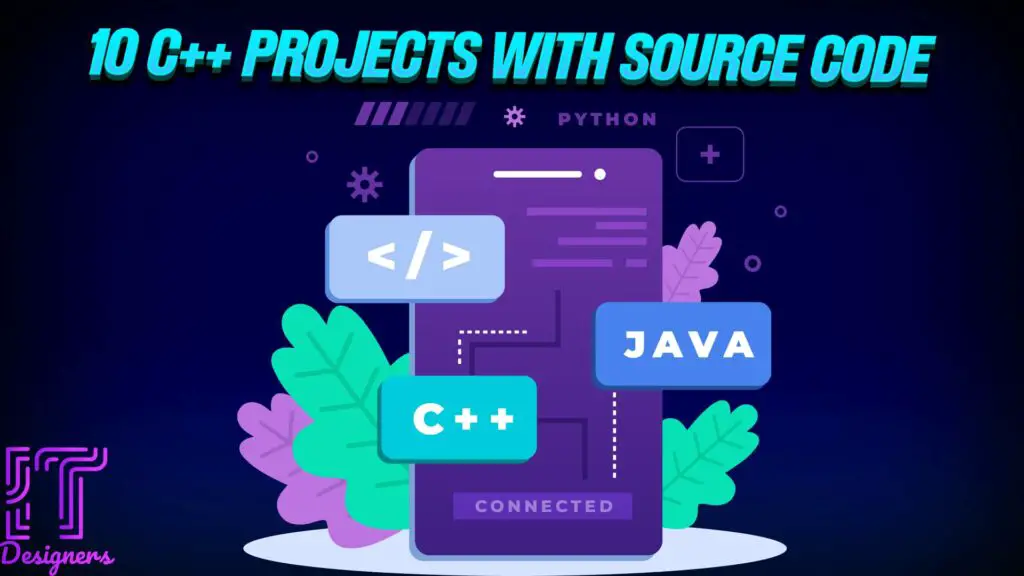
The C++ programming language is widely recognized for offering numerous advantages compared to the C language. The most interesting feature of this language is its support for object-oriented programming, which opens up a completely new realm of possibilities. We are pleased to provide you with over 10 C++ projects with source code for learners.
One of the most popular programming languages that is widely used in many different industries, including games, operating systems, web browsers, and database management systems, is C++.
Because it incorporates object-oriented programming concepts, such as the use of specified classes, into the C programming language, some refer to C++ as “C with classes.” Over time, C++ has continued to be a very useful language for computer programming as well as to teach new programmers how object-oriented programming operates.
C++ Projects with Source Code
1. Bank Management System Project
This project is a simple C++ Project with source code. Bank Management System Project in C++ uses C++ to manage banking operations like account creation, deposits, and withdrawals. Users interact with the system, providing input for tasks such as updating account details. C++ is chosen for its efficiency and versatility, allowing the implementation of features like data processing and user interfaces. The output includes updated account balances and transaction receipts displayed to users.
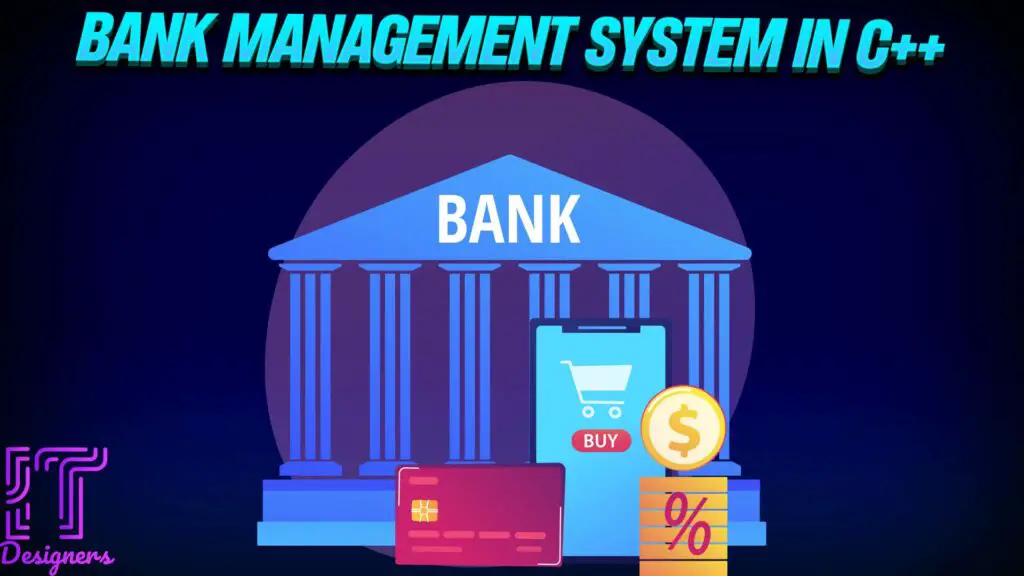
#include <iostream>
#include <map>
#include <string>
using namespace std;
class Bank {
private:
map<string, double> accounts;
public:
void createAccount(const string& name, double initialDeposit) {
if (accounts.find(name) != accounts.end()) {
cout << "Account already exists!" << endl;
} else {
accounts[name] = initialDeposit;
cout << "Account created successfully!" << endl;
}
}
void deposit(const string& name, double amount) {
if (accounts.find(name) != accounts.end()) {
accounts[name] += amount;
cout << "Amount deposited successfully!" << endl;
} else {
cout << "Account does not exist!" << endl;
}
}
void withdraw(const string& name, double amount) {
if (accounts.find(name) != accounts.end()) {
if (accounts[name] >= amount) {
accounts[name] -= amount;
cout << "Amount withdrawn successfully!" << endl;
} else {
cout << "Insufficient balance!" << endl;
}
} else {
cout << "Account does not exist!" << endl;
}
}
void checkBalance(const string& name) {
if (accounts.find(name) != accounts.end()) {
cout << "Balance for account " << name << " is: " << accounts[name] << endl;
} else {
cout << "Account does not exist!" << endl;
}
}
};
int main() {
Bank bank;
// Sample usage
bank.createAccount("Alice", 1000);
bank.deposit("Alice", 500);
bank.checkBalance("Alice");
bank.withdraw("Alice", 200);
bank.checkBalance("Alice");
return 0;
}
2. Train Reservation System Project
The concept of the project is a train reservation system to reserve train tickets for multiple destinations. In this project, C++ is used to implement the logic for seat availability, reservation confirmation, and the generation of booking records. C++ is utilized to handle data processing, implement user interfaces, and manage the overall flow of the reservation application. The language allows for implementing key functionalities required for a reservation system, providing users with a straightforward and reliable platform to manage their train travel plans.
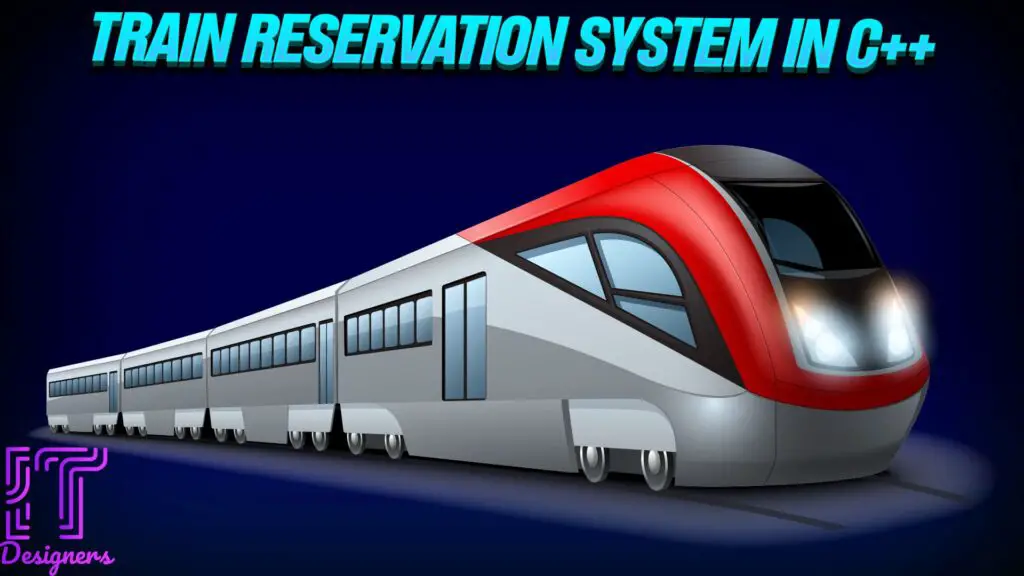
#include <iostream>
#include <map>
#include <vector>
#include <string>
using namespace std;
class TrainReservationSystem {
private:
map<int, int> availableSeats; // Map to store available seats for each train
public:
TrainReservationSystem() {
// Initialize available seats for each train
availableSeats[1] = 50;
availableSeats[2] = 50;
availableSeats[3] = 50;
}
void displayAvailableSeats() {
cout << "Available Seats:" << endl;
for (const auto& pair : availableSeats) {
cout << "Train " << pair.first << ": " << pair.second << " seats" << endl;
}
}
void bookTicket(int trainNumber, int numTickets) {
if (availableSeats.find(trainNumber) != availableSeats.end()) {
if (availableSeats[trainNumber] >= numTickets) {
availableSeats[trainNumber] -= numTickets;
cout << numTickets << " ticket(s) booked successfully for Train " << trainNumber << endl;
} else {
cout << "Insufficient seats available for Train " << trainNumber << endl;
}
} else {
cout << "Invalid Train Number" << endl;
}
}
void cancelTicket(int trainNumber, int numTickets) {
if (availableSeats.find(trainNumber) != availableSeats.end()) {
availableSeats[trainNumber] += numTickets;
cout << numTickets << " ticket(s) canceled successfully for Train " << trainNumber << endl;
} else {
cout << "Invalid Train Number" << endl;
}
}
};
int main() {
TrainReservationSystem trainSystem;
int choice, trainNumber, numTickets;
do {
cout << "\nTrain Reservation System" << endl;
cout << "1. Display Available Seats" << endl;
cout << "2. Book Ticket" << endl;
cout << "3. Cancel Ticket" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1:
trainSystem.displayAvailableSeats();
break;
case 2:
cout << "Enter Train Number: ";
cin >> trainNumber;
cout << "Enter Number of Tickets: ";
cin >> numTickets;
trainSystem.bookTicket(trainNumber, numTickets);
break;
case 3:
cout << "Enter Train Number: ";
cin >> trainNumber;
cout << "Enter Number of Tickets: ";
cin >> numTickets;
trainSystem.cancelTicket(trainNumber, numTickets);
break;
case 4:
cout << "Exiting Program..." << endl;
break;
default:
cout << "Invalid choice! Please try again." << endl;
}
} while (choice != 4);
return 0;
}
3. Transport Management System Project
This project in C++ is based on the project idea of the management system developed for students and others. It aims to enhance efficiency in managing transportation resources and schedules. This Project in C++ involves building a program to manage transportation operations efficiently, and the C++ programming language is employed for its effectiveness in handling such projects. Users interact with the system by providing input such as route details, vehicle information, and scheduling preferences. The user must pass through a login. C++ implements the logic for route optimization, vehicle allocation, and tracking transportation schedules.
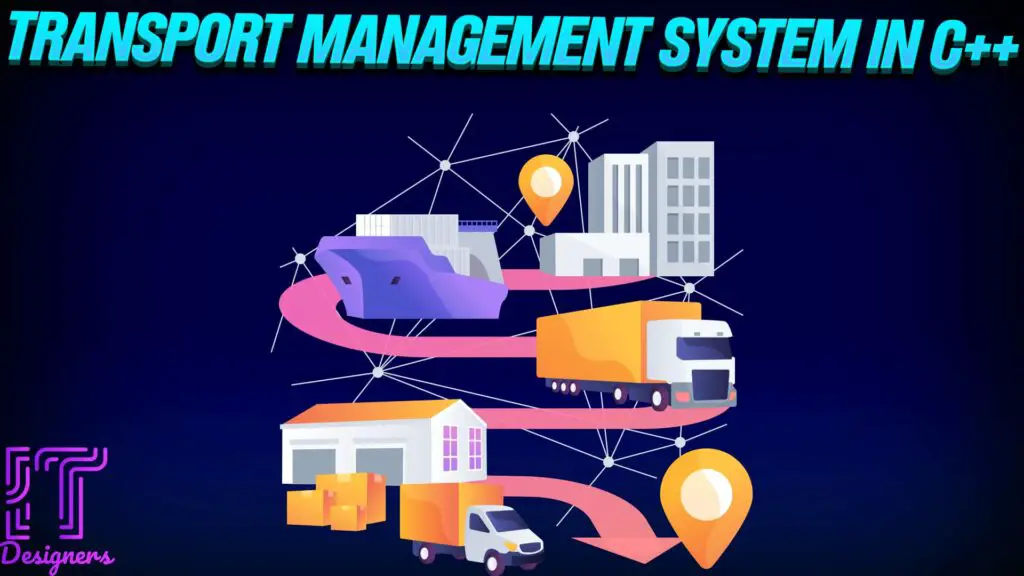
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Vehicle {
private:
string regNumber;
string model;
string type;
int capacity;
string assignedDriver;
public:
Vehicle(const string& reg, const string& mdl, const string& tp, int cap)
: regNumber(reg), model(mdl), type(tp), capacity(cap), assignedDriver("None") {}
const string& getRegNumber() const { return regNumber; }
const string& getModel() const { return model; }
const string& getType() const { return type; }
int getCapacity() const { return capacity; }
const string& getAssignedDriver() const { return assignedDriver; }
void assignDriver(const string& driverName) {
assignedDriver = driverName;
}
};
class Driver {
private:
string name;
string licenseNumber;
public:
Driver(const string& n, const string& lic) : name(n), licenseNumber(lic) {}
const string& getName() const { return name; }
const string& getLicenseNumber() const { return licenseNumber; }
};
class TransportManagementSystem {
private:
vector<Vehicle> vehicles;
vector<Driver> drivers;
public:
void addVehicle(const string& reg, const string& model, const string& type, int capacity) {
vehicles.emplace_back(reg, model, type, capacity);
cout << "Vehicle added successfully!" << endl;
}
void addDriver(const string& name, const string& licenseNumber) {
drivers.emplace_back(name, licenseNumber);
cout << "Driver added successfully!" << endl;
}
void assignDriverToVehicle(const string& regNumber, const string& driverName) {
for (auto& vehicle : vehicles) {
if (vehicle.getRegNumber() == regNumber) {
vehicle.assignDriver(driverName);
cout << "Driver assigned successfully to Vehicle: " << regNumber << endl;
return;
}
}
cout << "Vehicle not found!" << endl;
}
void displayVehicles() {
cout << "Registered Vehicles:" << endl;
for (const auto& vehicle : vehicles) {
cout << "Registration Number: " << vehicle.getRegNumber() << ", Model: " << vehicle.getModel()
<< ", Type: " << vehicle.getType() << ", Capacity: " << vehicle.getCapacity()
<< ", Assigned Driver: " << vehicle.getAssignedDriver() << endl;
}
}
void displayDrivers() {
cout << "Registered Drivers:" << endl;
for (const auto& driver : drivers) {
cout << "Name: " << driver.getName() << ", License Number: " << driver.getLicenseNumber() << endl;
}
}
};
int main() {
TransportManagementSystem tms;
// Adding vehicles
tms.addVehicle("ABC123", "Toyota Camry", "Sedan", 4);
tms.addVehicle("XYZ456", "Ford Transit", "Van", 10);
// Adding drivers
tms.addDriver("John Doe", "DL12345");
tms.addDriver("Jane Smith", "DL67890");
// Assigning driver to vehicle
tms.assignDriverToVehicle("ABC123", "John Doe");
// Displaying vehicles and drivers
tms.displayVehicles();
tms.displayDrivers();
return 0;
}
4. Credit Card Validator
Credit cards are essential in this digital age where e-commerce is king. Before completing the payment, most payment gateways use some validation process for credit card information.
A Credit Card Validator in C++ involves creating a program to check the validity of credit card numbers. Users input credit card numbers into the system, and C++ algorithms check if the supplied numbers follow common validation guidelines.
The output of the Credit Card Validator confirms whether the inputted credit card numbers are valid. C++ processes the input data applies the validation logic and generates clear user output messages.
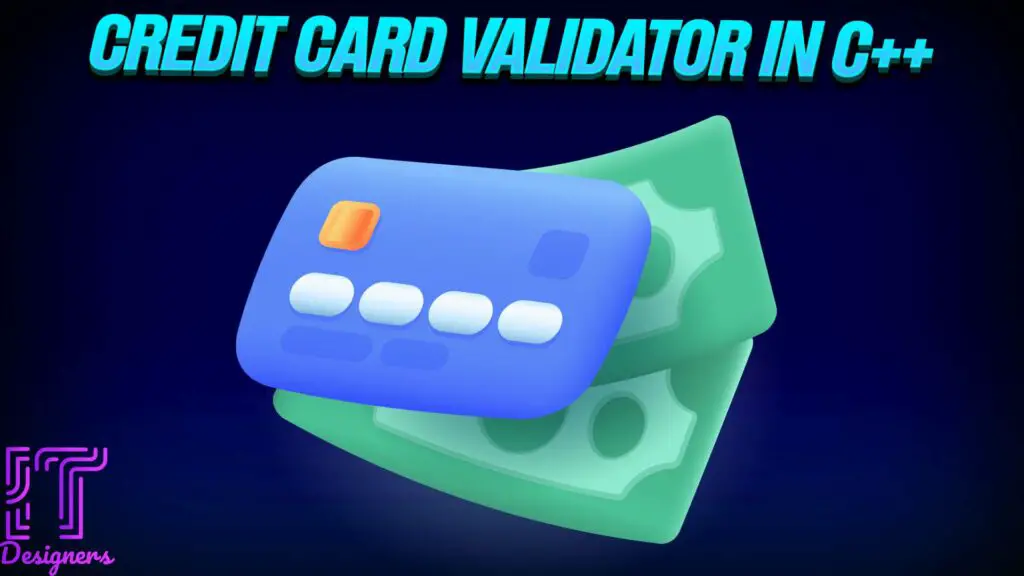
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
bool isValidCreditCard(const string& cardNumber) {
// Remove spaces and dashes from the card number
string cleanNumber = "";
for (char c : cardNumber) {
if (c != ' ' && c != '-') {
cleanNumber += c;
}
}
// Check if the card number contains only digits
if (!all_of(cleanNumber.begin(), cleanNumber.end(), ::isdigit)) {
return false;
}
// Reverse the card number
reverse(cleanNumber.begin(), cleanNumber.end());
int sum = 0;
bool doubleDigit = false;
for (size_t i = 0; i < cleanNumber.length(); ++i) {
int digit = cleanNumber[i] - '0';
// Double every second digit
if (doubleDigit) {
digit *= 2;
if (digit > 9) {
digit -= 9; // Subtract 9 if the result is a two-digit number
}
}
sum += digit;
doubleDigit = !doubleDigit;
}
// The card number is valid if the sum is a multiple of 10
return (sum % 10 == 0);
}
int main() {
string cardNumber;
cout << "Enter credit card number: ";
getline(cin, cardNumber);
if (isValidCreditCard(cardNumber)) {
cout << "Valid credit card number." << endl;
} else {
cout << "Invalid credit card number." << endl;
}
return 0;
}
5. Car Parking Management System
The aim of this project behind the car parking reservation system in C++ is to produce and store parking details, including the total charge. In this Project, C++ is used to implement the logic for allocating parking spaces, processing payment transactions, and generating parking tickets. Users input information by specifying parking preferences, such as duration and vehicle details. C++ plays a crucial role in handling data processing, implementing user interfaces, and managing the overall flow of the parking management application. Then, the output of this project’s parking confirmation, payment receipts, and real-time availability updates are displayed to users. Projects play a crucial role in handling data processing, implementing user interfaces, and managing the overall flow of the parking management application.
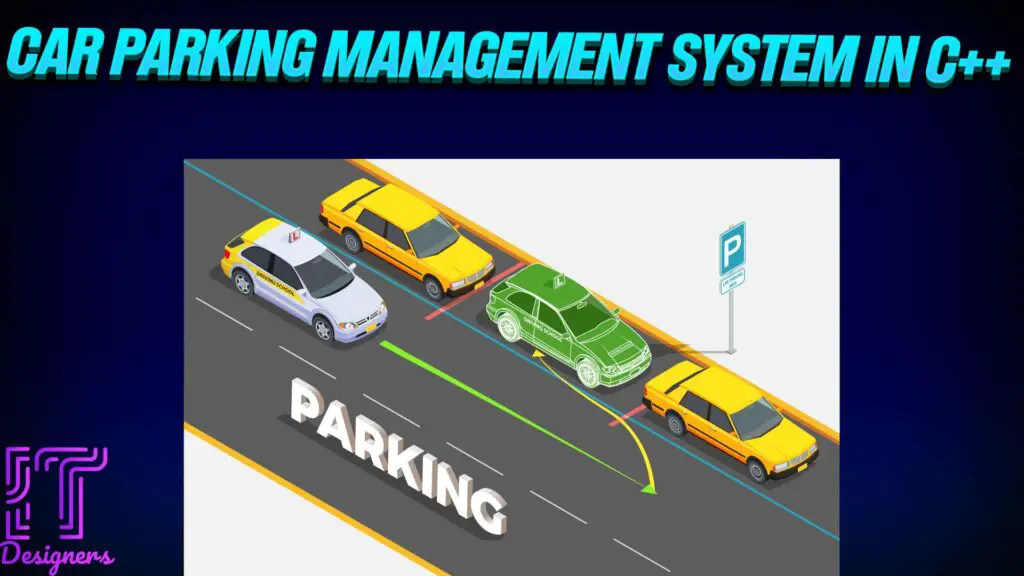
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class ParkingSystem {
private:
int capacity;
int occupied;
public:
ParkingSystem(int cap) : capacity(cap), occupied(0) {}
bool parkCar() {
if (occupied < capacity) {
occupied++;
cout << "Car parked successfully." << endl;
return true;
} else {
cout << "Parking lot is full. Cannot park car." << endl;
return false;
}
}
void removeCar() {
if (occupied > 0) {
occupied--;
cout << "Car removed from parking lot." << endl;
} else {
cout << "Parking lot is already empty." << endl;
}
}
void displayAvailableSpaces() {
int available = capacity - occupied;
cout << "Available parking spaces: " << available << endl;
}
};
int main() {
// Creating a parking lot with capacity of 10 cars
ParkingSystem parkingLot(10);
int choice;
do {
cout << "\nCar Parking System" << endl;
cout << "1. Park Car" << endl;
cout << "2. Remove Car" << endl;
cout << "3. Display Available Spaces" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1:
parkingLot.parkCar();
break;
case 2:
parkingLot.removeCar();
break;
case 3:
parkingLot.displayAvailableSpaces();
break;
case 4:
cout << "Exiting Program..." << endl;
break;
default:
cout << "Invalid choice! Please try again." << endl;
}
} while (choice != 4);
return 0;
}
6. Simple ATM System
It aims to offer a secure and accessible solution for basic banking operations. A simple ATM program is a completely functional project that uses the C++ programming language in a file management database to create an ATM program system in C++. This project designed a program to manage basic ATM operations. C++ is used to implement the logic for account verification, transaction processing, and updating account balances. C++ in handling data processing, implementing user interfaces, and managing the overall flow of the ATM application.
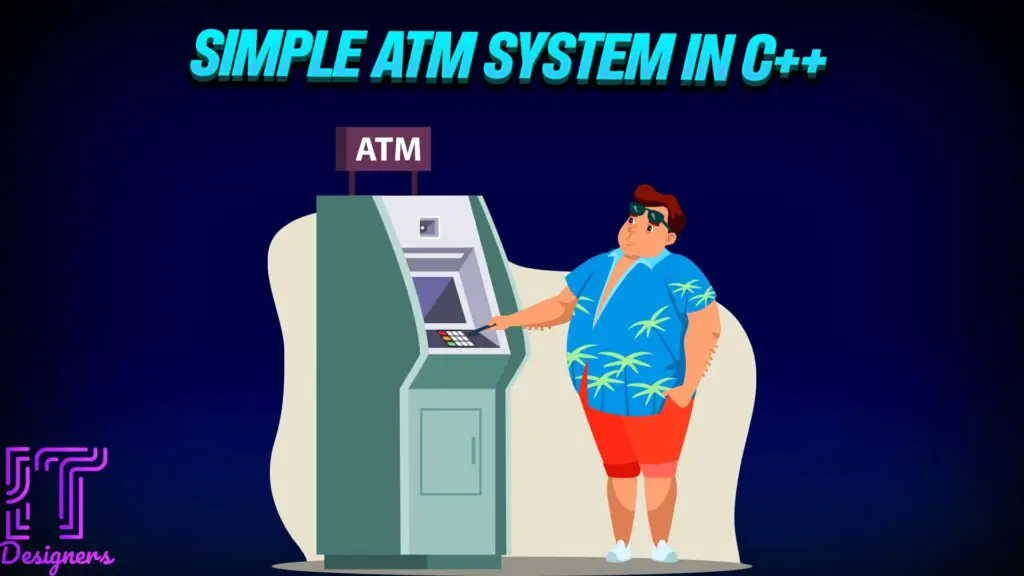
#include <iostream>
using namespace std;
class ATM {
private:
double balance;
public:
ATM(double initialBalance) : balance(initialBalance) {}
void checkBalance() {
cout << "Your balance is: $" << balance << endl;
}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
cout << "Deposit successful. Current balance: $" << balance << endl;
} else {
cout << "Invalid amount for deposit." << endl;
}
}
void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
cout << "Withdrawal successful. Current balance: $" << balance << endl;
} else {
cout << "Invalid amount for withdrawal or insufficient funds." << endl;
}
}
};
int main() {
double initialBalance;
cout << "Enter initial balance: ";
cin >> initialBalance;
ATM atm(initialBalance);
int choice;
do {
cout << "\nATM Menu" << endl;
cout << "1. Check Balance" << endl;
cout << "2. Deposit" << endl;
cout << "3. Withdraw" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1: {
atm.checkBalance();
break;
}
case 2: {
double depositAmount;
cout << "Enter deposit amount: $";
cin >> depositAmount;
atm.deposit(depositAmount);
break;
}
case 3: {
double withdrawAmount;
cout << "Enter withdrawal amount: $";
cin >> withdrawAmount;
atm.withdraw(withdrawAmount);
break;
}
case 4: {
cout << "Exiting ATM system..." << endl;
break;
}
default: {
cout << "Invalid choice! Please try again." << endl;
break;
}
}
} while (choice != 4);
return 0;
}
7. Inventory Management System
This project is good for the final year. And if you don’t know the coding so, don’t worry. We provide this C++ project with source code that is easy to understand. This project aims to create a program to track and manage inventory items. The inventory management system project is a fully functional inventory management system in C++ that uses a file management database with the C++ programming language and a file management database. This project, C++ is used to implement the logic for inventory manipulation, order processing, and report generation.
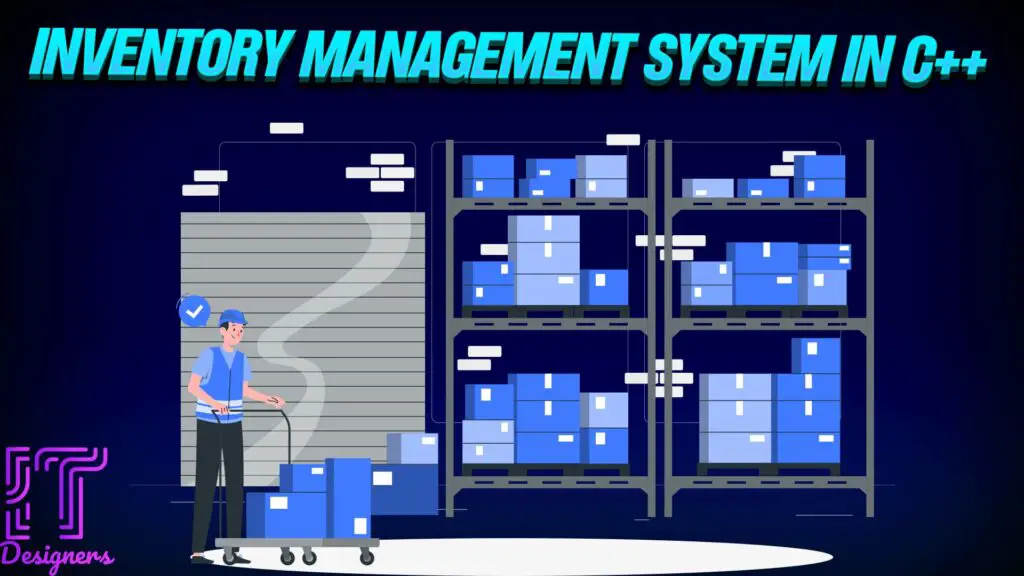
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Product structure
struct Product {
string name;
int quantity;
double price;
Product(const string& n, int q, double p) : name(n), quantity(q), price(p) {}
};
class InventoryManagementSystem {
private:
vector<Product> products;
public:
void addProduct(const string& name, int quantity, double price) {
products.push_back(Product(name, quantity, price));
cout << "Product added successfully." << endl;
}
void removeProduct(const string& name) {
for (auto it = products.begin(); it != products.end(); ++it) {
if (it->name == name) {
products.erase(it);
cout << "Product removed successfully." << endl;
return;
}
}
cout << "Product not found." << endl;
}
void displayAllProducts() {
cout << "All Products:" << endl;
for (const auto& product : products) {
cout << "Name: " << product.name << ", Quantity: " << product.quantity
<< ", Price: $" << product.price << endl;
}
}
void searchProduct(const string& name) {
cout << "Search Results:" << endl;
bool found = false;
for (const auto& product : products) {
if (product.name == name) {
cout << "Name: " << product.name << ", Quantity: " << product.quantity
<< ", Price: $" << product.price << endl;
found = true;
}
}
if (!found) {
cout << "Product not found." << endl;
}
}
};
int main() {
InventoryManagementSystem ims;
int choice;
do {
cout << "\nInventory Management System" << endl;
cout << "1. Add Product" << endl;
cout << "2. Remove Product" << endl;
cout << "3. Display All Products" << endl;
cout << "4. Search Product" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1: {
string name;
int quantity;
double price;
cout << "Enter product name: ";
cin.ignore();
getline(cin, name);
cout << "Enter quantity: ";
cin >> quantity;
cout << "Enter price: $";
cin >> price;
ims.addProduct(name, quantity, price);
break;
}
case 2: {
string name;
cout << "Enter product name to remove: ";
cin.ignore();
getline(cin, name);
ims.removeProduct(name);
break;
}
case 3: {
ims.displayAllProducts();
break;
}
case 4: {
string name;
cout << "Enter product name to search: ";
cin.ignore();
getline(cin, name);
ims.searchProduct(name);
break;
}
case 5: {
cout << "Exiting Inventory Management System..." << endl;
break;
}
default: {
cout << "Invalid choice! Please try again." << endl;
break;
}
}
} while (choice != 5);
return 0;
}
8. Student Attendance System
Design a text-based adventure game where players navigate through a series of rooms, solve puzzles, and make decisions that affect the outcome of the game.
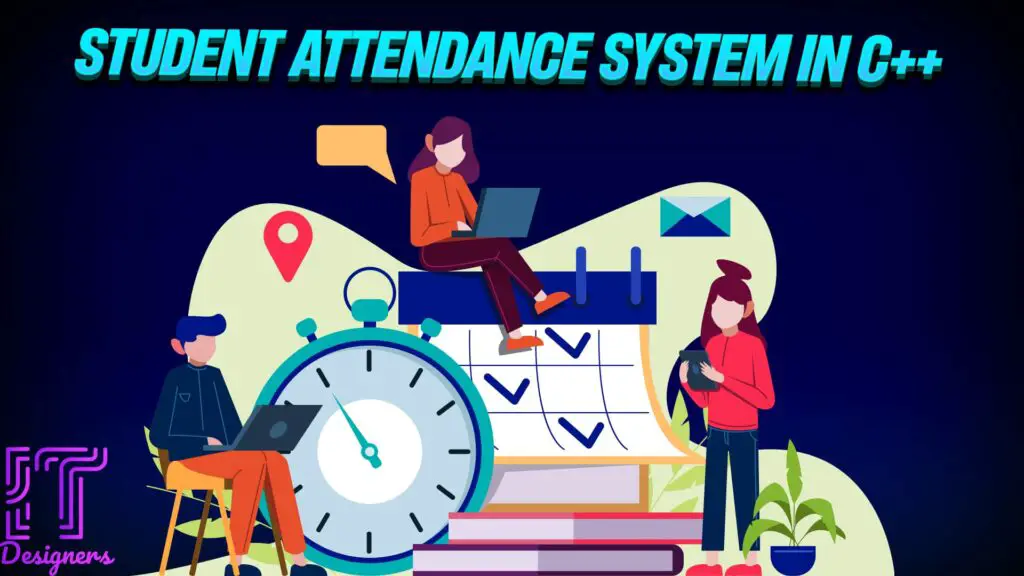
#include <iostream>
#include <unordered_map>
#include <vector>
#include <string>
using namespace std;
class StudentAttendanceSystem {
private:
unordered_map<string, vector<bool>> attendanceRecords;
public:
void markAttendance(const string& studentName, bool present) {
attendanceRecords[studentName].push_back(present);
cout << "Attendance marked for student: " << studentName << endl;
}
void displayAttendance(const string& studentName) {
if (attendanceRecords.find(studentName) != attendanceRecords.end()) {
int totalClasses = attendanceRecords[studentName].size();
int presentCount = 0;
for (bool present : attendanceRecords[studentName]) {
if (present) {
presentCount++;
}
}
double attendancePercentage = (static_cast<double>(presentCount) / totalClasses) * 100;
cout << "Attendance for student " << studentName << ":" << endl;
cout << "Total classes: " << totalClasses << endl;
cout << "Classes attended: " << presentCount << endl;
cout << "Attendance Percentage: " << attendancePercentage << "%" << endl;
} else {
cout << "No attendance record found for student: " << studentName << endl;
}
}
};
int main() {
StudentAttendanceSystem attendanceSystem;
int choice;
do {
cout << "\nStudent Attendance System" << endl;
cout << "1. Mark Attendance" << endl;
cout << "2. View Attendance" << endl;
cout << "3. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1: {
string name;
bool present;
cout << "Enter student name: ";
cin.ignore();
getline(cin, name);
cout << "Is the student present? (1 for Yes / 0 for No): ";
cin >> present;
attendanceSystem.markAttendance(name, present);
break;
}
case 2: {
string name;
cout << "Enter student name to view attendance: ";
cin.ignore();
getline(cin, name);
attendanceSystem.displayAttendance(name);
break;
}
case 3: {
cout << "Exiting Student Attendance System..." << endl;
break;
}
default: {
cout << "Invalid choice! Please try again." << endl;
break;
}
}
} while (choice != 3);
return 0;
}
9. Automated Stock Trading System
Use C++ to implement an automated stock trading system. Make trading decisions in real-time by utilizing market data and financial algorithms. Provide tools for portfolio analysis, risk management, and user customization. This project uses C++ to make trading decisions based on predefined algorithms and market data. Users provide input by setting trading parameters, and C++ is used to implement the logic for analyzing market trends, executing trades, and managing portfolio positions.
Technologies Necessary:
- The programming language
- C++ Financial algorithms
- Current market information
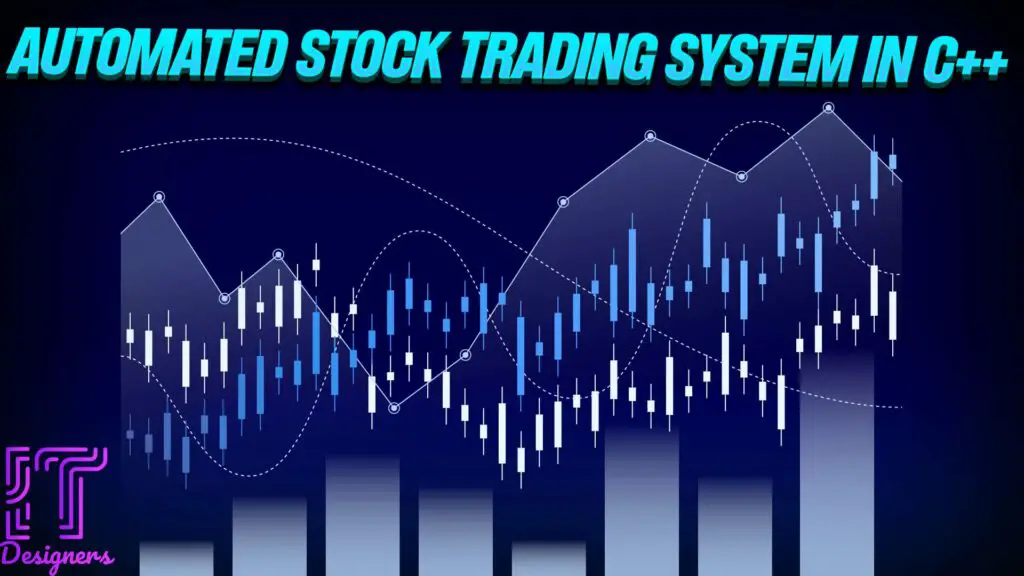
#include <iostream>
#include <vector>
#include <string>
#include <cstdlib> // For rand() function (used for simulation)
using namespace std;
// Define a simple structure to represent stock data
struct Stock {
string symbol;
double price;
};
// Class representing the automated stock trading system
class AutomatedStockTradingSystem {
private:
vector<Stock> stocks;
public:
// Fetch stock data (simulated in this example)
void fetchStockData() {
// Simulate fetching stock data
stocks = { {"AAPL", 150.0}, {"GOOGL", 2500.0}, {"MSFT", 300.0}, {"AMZN", 3500.0} };
}
// Simulate making trading decisions based on stock data
void makeTradingDecisions() {
// Simulate trading decisions
cout << "Making trading decisions..." << endl;
for (const auto& stock : stocks) {
// Simulate a simple trading algorithm (buy if price < 100, sell if price > 200)
if (stock.price < 100.0) {
cout << "Buy " << stock.symbol << " at $" << stock.price << endl;
} else if (stock.price > 200.0) {
cout << "Sell " << stock.symbol << " at $" << stock.price << endl;
}
}
}
// Simulate executing trades
void executeTrades() {
// Simulate executing trades
cout << "Executing trades..." << endl;
// In a real system, this would involve interfacing with a broker API
cout << "Trades executed successfully." << endl;
}
};
int main() {
AutomatedStockTradingSystem tradingSystem;
// Fetch stock data
tradingSystem.fetchStockData();
// Make trading decisions based on stock data
tradingSystem.makeTradingDecisions();
// Execute trades
tradingSystem.executeTrades();
return 0;
}
10. Car Rental System
This project aims to enhance renting vehicles’ overall efficiency and user experience. Customers can reserve and book rental cars using an online or offline software application called a car rental reservation system (CRSS).
These systems frequently have features such as:
- Search:Customers can search for cars available for hire at a particular location and time.
- Pricing:Rates for various car kinds and rental durations are visible to customers.
- Booking:Customers can make a car reservation by supplying their payment information and contact data.
- Confirmation: Upon booking, customers receive an email containing reservation details.
Users input information by specifying rental details, such as pickup dates, return dates, and vehicle preferences. C++ is used to implement the logic for checking vehicle availability, processing rental transactions, and generating rental agreements.
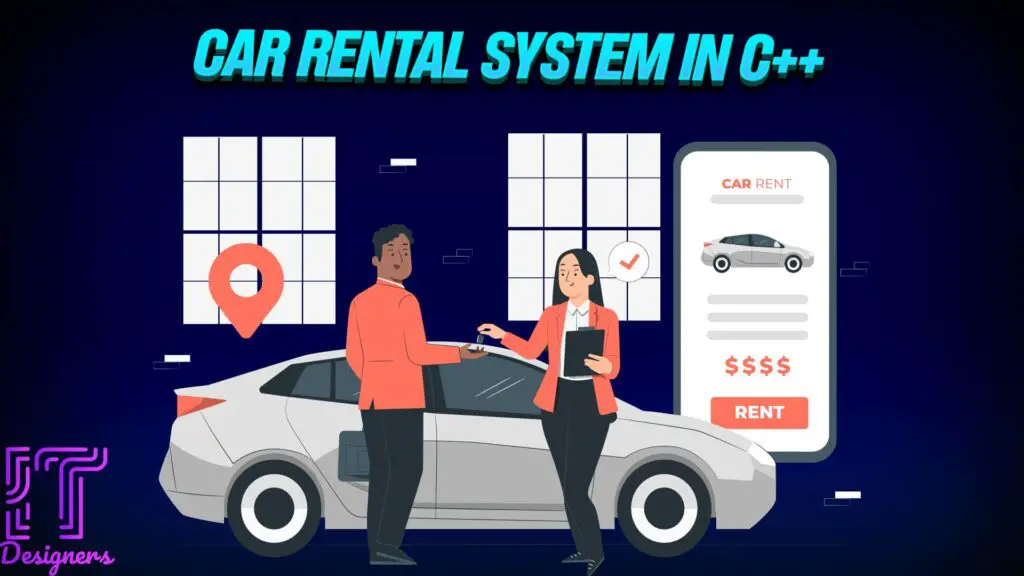
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Car class representing a car object
class Car {
private:
string model;
string licensePlate;
bool available;
public:
Car(const string& mdl, const string& lic) : model(mdl), licensePlate(lic), available(true) {}
const string& getModel() const { return model; }
const string& getLicensePlate() const { return licensePlate; }
bool isAvailable() const { return available; }
void rentCar() {
if (available) {
available = false;
cout << "Car rented successfully." << endl;
} else {
cout << "Car is not available for rent." << endl;
}
}
void returnCar() {
if (!available) {
available = true;
cout << "Car returned successfully." << endl;
} else {
cout << "Car is already available." << endl;
}
}
};
// CarRentalSystem class representing the car rental system
class CarRentalSystem {
private:
vector<Car> cars;
public:
void addCar(const string& model, const string& licensePlate) {
cars.emplace_back(model, licensePlate);
cout << "Car added successfully." << endl;
}
void rentCar(const string& licensePlate) {
for (auto& car : cars) {
if (car.getLicensePlate() == licensePlate) {
car.rentCar();
return;
}
}
cout << "Car not found." << endl;
}
void returnCar(const string& licensePlate) {
for (auto& car : cars) {
if (car.getLicensePlate() == licensePlate) {
car.returnCar();
return;
}
}
cout << "Car not found." << endl;
}
void displayAvailableCars() {
cout << "Available Cars:" << endl;
for (const auto& car : cars) {
if (car.isAvailable()) {
cout << "Model: " << car.getModel() << ", License Plate: " << car.getLicensePlate() << endl;
}
}
}
};
int main() {
CarRentalSystem rentalSystem;
int choice;
do {
cout << "\nCar Rental System" << endl;
cout << "1. Add Car" << endl;
cout << "2. Rent Car" << endl;
cout << "3. Return Car" << endl;
cout << "4. Display Available Cars" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1: {
string model, licensePlate;
cout << "Enter car model: ";
cin.ignore();
getline(cin, model);
cout << "Enter license plate: ";
getline(cin, licensePlate);
rentalSystem.addCar(model, licensePlate);
break;
}
case 2: {
string licensePlate;
cout << "Enter license plate of the car to rent: ";
cin.ignore();
getline(cin, licensePlate);
rentalSystem.rentCar(licensePlate);
break;
}
case 3: {
string licensePlate;
cout << "Enter license plate of the car to return: ";
cin.ignore();
getline(cin, licensePlate);
rentalSystem.returnCar(licensePlate);
break;
}
case 4: {
rentalSystem.displayAvailableCars();
break;
}
case 5: {
cout << "Exiting Car Rental System..." << endl;
break;
}
default: {
cout << "Invalid choice! Please try again." << endl;
break;
}
}
} while (choice != 5);
return 0;
}
C++ offers a powerful combination of low-level and high-level programming capabilities, making it suitable for various projects. Its versatility and performance make it ideal for showcasing your programming skills.
Focus on writing clean, efficient code, incorporating modern technologies, and compelling demos and documentation. Building an online portfolio on platforms like GitHub also adds credibility to your work.
Yes, a user-friendly interface enhances the overall user experience and makes your project more accessible. Spend time designing an intuitive interface that complements the functionality of your project.
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
10 Advance Final Year Project Ideas with Source Code
-
Realtime Object Detection
-
E Commerce sales forecasting using machine learning
-
AI Music Composer project with source code
-
Stock market Price Prediction using machine learning
-
30 Final Year Project Ideas for IT Students
-
c++ Projects for beginners
-
Python Projects For Final Year Students With Source Code
-
Top 10 Best JAVA Final Year Projects
-
20 Exiciting Cyber Security Final Year Projects
-
C++ Projects with Source Code
-
Artificial Intelligence Projects For Final Year
-
How to Host HTML website for free?
-
How to Download image in HTML
-
Hate Speech Detection Using Machine Learning
-
10 Web Development Projects for beginners
-
Fake news detection using machine learning source code
-
Credit Card Fraud detection using machine learning