Best 20 python projects for beginners with source code
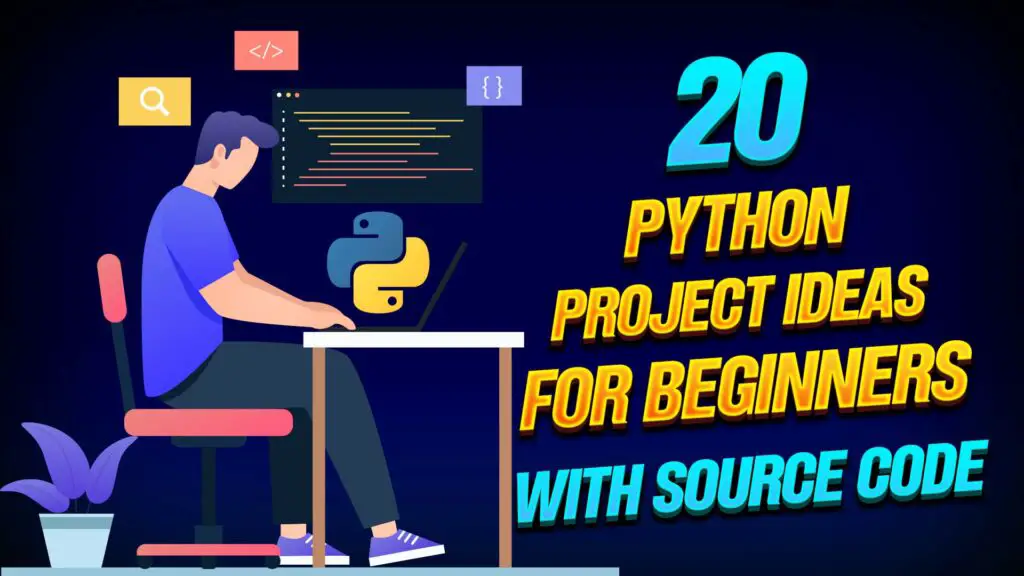
Are you an aspiring Python programmer looking for creative projects to sharpen your skills? Whether you’re a beginner or have some coding experience, practicing on projects is a fantastic way to support your learning and apply your knowledge to real-world scenarios. Below, we have combined 20 Python projects with source code specifically for beginners. Let’s dip in and explore each idea in detail.
1. To-Do List Application
Create a command-line or GUI-based to-do list application using Python. Users should be able to add, delete, update, and view tasks, with options to mark tasks as completed.
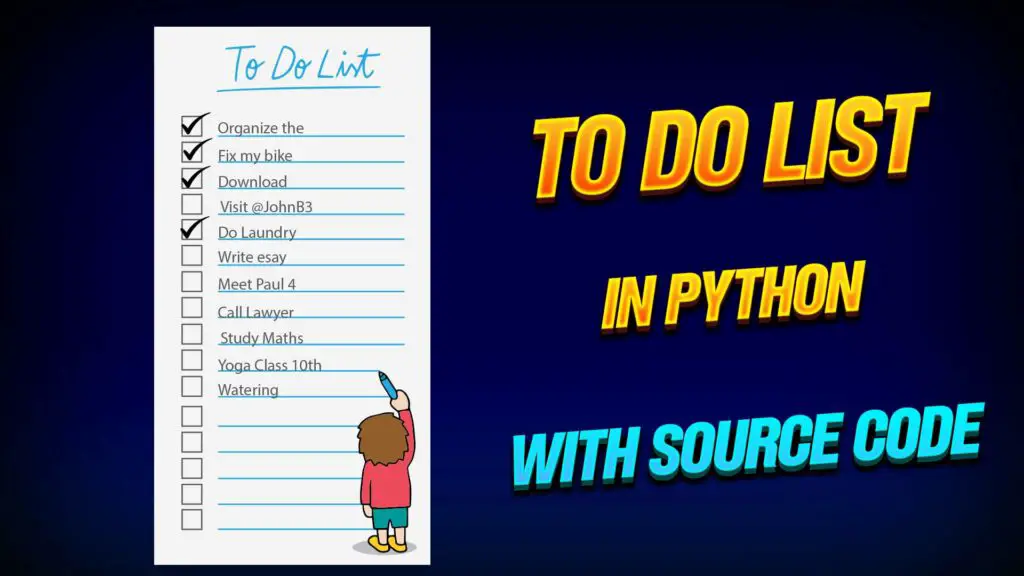
# Define an empty list to store tasks
tasks = []
# Function to add a new task
def add_task():
task = input("Enter the task: ")
tasks.append({"task": task, "completed": False})
print("Task added successfully!")
# Function to view all tasks
def view_tasks():
if not tasks:
print("No tasks found.")
else:
print("Tasks:")
for idx, task in enumerate(tasks, start=1):
status = "Done" if task["completed"] else "Not Done"
print(f"{idx}. {task['task']} - {status}")
# Function to mark a task as completed
def mark_completed():
view_tasks()
idx = int(input("Enter the task number to mark as completed: ")) - 1
if 0 <= idx < len(tasks):
tasks[idx]["completed"] = True
print("Task marked as completed!")
else:
print("Invalid task number.")
# Function to delete a task
def delete_task():
view_tasks()
idx = int(input("Enter the task number to delete: ")) - 1
if 0 <= idx < len(tasks):
del tasks[idx]
print("Task deleted successfully!")
else:
print("Invalid task number.")
# Main function to run the To-Do List Application
def main():
while True:
print("\nTo-Do List Application")
print("1. Add Task")
print("2. View Tasks")
print("3. Mark Task as Completed")
print("4. Delete Task")
print("5. Exit")
choice = input("Enter your choice (1-5): ")
if choice == "1":
add_task()
elif choice == "2":
view_tasks()
elif choice == "3":
mark_completed()
elif choice == "4":
delete_task()
elif choice == "5":
print("Thank you for using the To-Do List Application. Goodbye!")
break
else:
print("Invalid choice. Please enter a number from 1 to 5.")
if __name__ == "__main__":
main()
2. Weather App
Develop a weather application that fetches data from a weather API and displays current weather conditions, forecasts, and temperature trends for a specified location.
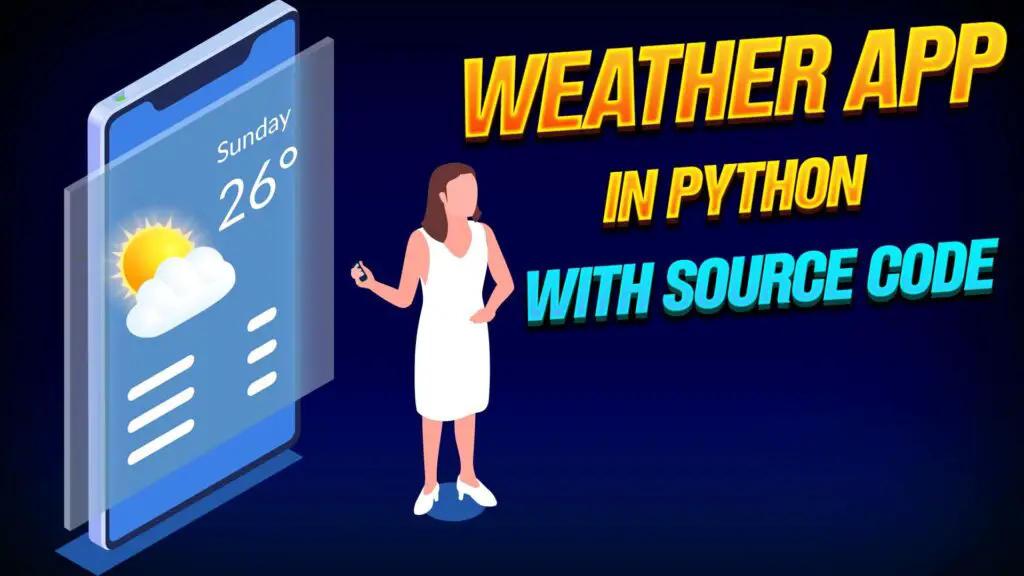
import requests
def get_weather(city):
# Replace 'YOUR_API_KEY' with your actual OpenWeatherMap API key
api_key = 'YOUR_API_KEY'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric'
response = requests.get(url)
data = response.json()
if data['cod'] == 200:
weather = {
'city': data['name'],
'temperature': data['main']['temp'],
'description': data['weather'][0]['description'],
'humidity': data['main']['humidity'],
'wind_speed': data['wind']['speed']
}
return weather
else:
return None
def main():
print("Welcome to the Weather App!")
city = input("Enter the city name: ")
weather = get_weather(city)
if weather:
print(f"Weather in {weather['city']}:")
print(f"Temperature: {weather['temperature']}°C")
print(f"Description: {weather['description']}")
print(f"Humidity: {weather['humidity']}%")
print(f"Wind Speed: {weather['wind_speed']} m/s")
else:
print("Weather data not available for the entered city.")
if __name__ == "__main__":
main()
3. Recipe Finder
Design a recipe finder application that retrieves recipes based on user preferences or ingredients. Integrate with a recipe API to fetch recipe details and display them to the user.
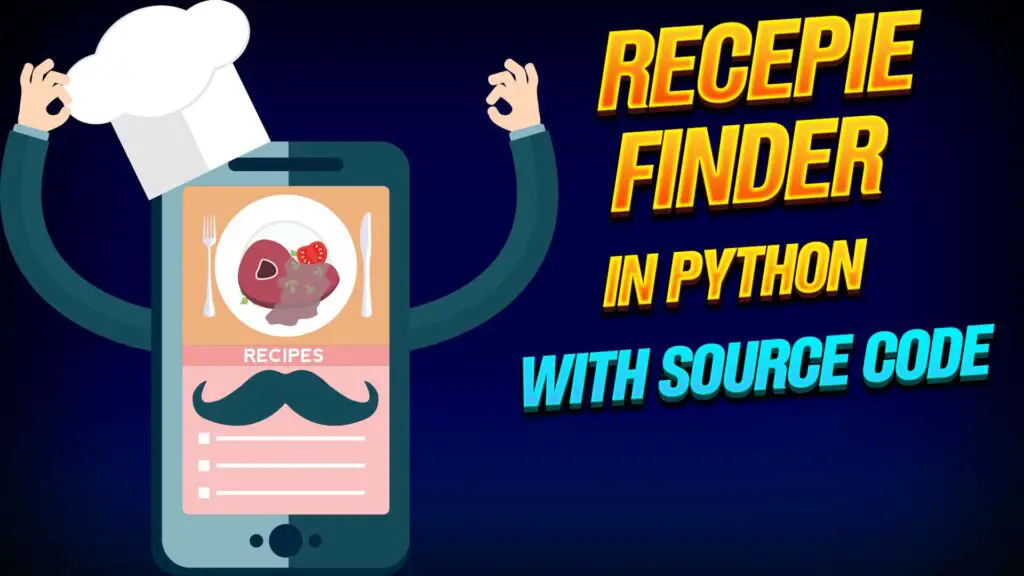
import requests
def find_recipes(query):
# Replace 'YOUR_API_KEY' with your actual Spoonacular API key
api_key = 'YOUR_API_KEY'
url = f'https://api.spoonacular.com/recipes/complexSearch?apiKey={api_key}&query={query}&number=5'
response = requests.get(url)
data = response.json()
if 'results' in data:
recipes = []
for result in data['results']:
recipe = {
'title': result['title'],
'url': result['sourceUrl']
}
recipes.append(recipe)
return recipes
else:
return None
def main():
print("Welcome to the Recipe Finder!")
query = input("Enter an ingredient or dish name: ")
recipes = find_recipes(query)
if recipes:
print(f"Here are some recipes containing '{query}':")
for idx, recipe in enumerate(recipes, start=1):
print(f"{idx}. {recipe['title']} - {recipe['url']}")
else:
print("No recipes found for the entered query.")
if __name__ == "__main__":
main()
4. URL Shortener
Create a URL shortening service similar to Bitly, where users can input long URLs and receive shortened versions that redirect to the original links.
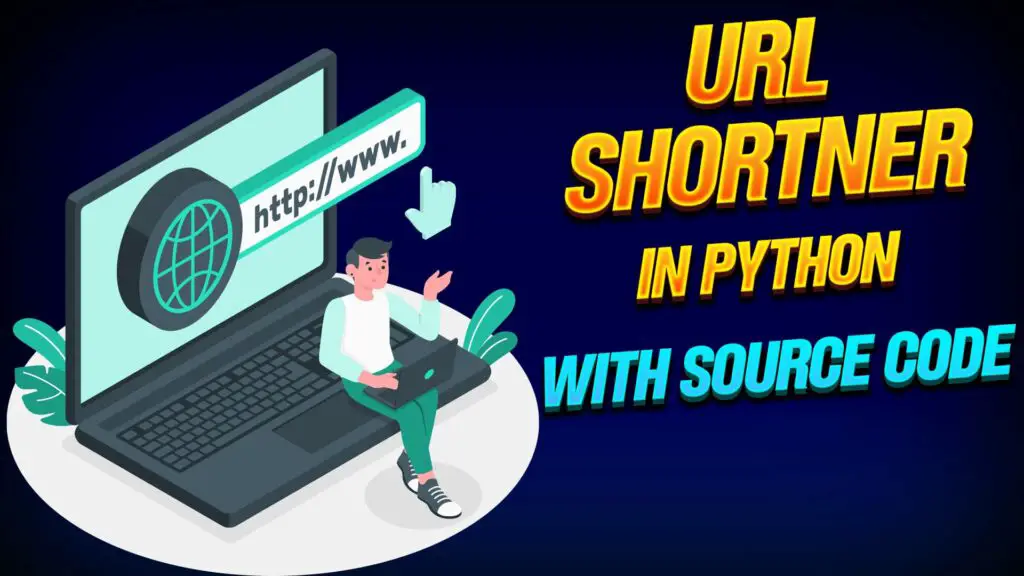
import pyshorteners
def shorten_url(url):
# Initialize a Shortener object
s = pyshorteners.Shortener()
# Shorten the URL
shortened_url = s.tinyurl.short(url)
return shortened_url
def main():
print("Welcome to the URL Shortener!")
url = input("Enter the URL to shorten: ")
shortened_url = shorten_url(url)
print("Shortened URL:", shortened_url)
if __name__ == "__main__":
main()
5. Password Generator
Develop a password generator tool that generates strong, randomized passwords based on user-specified criteria such as length, character types, and complexity.
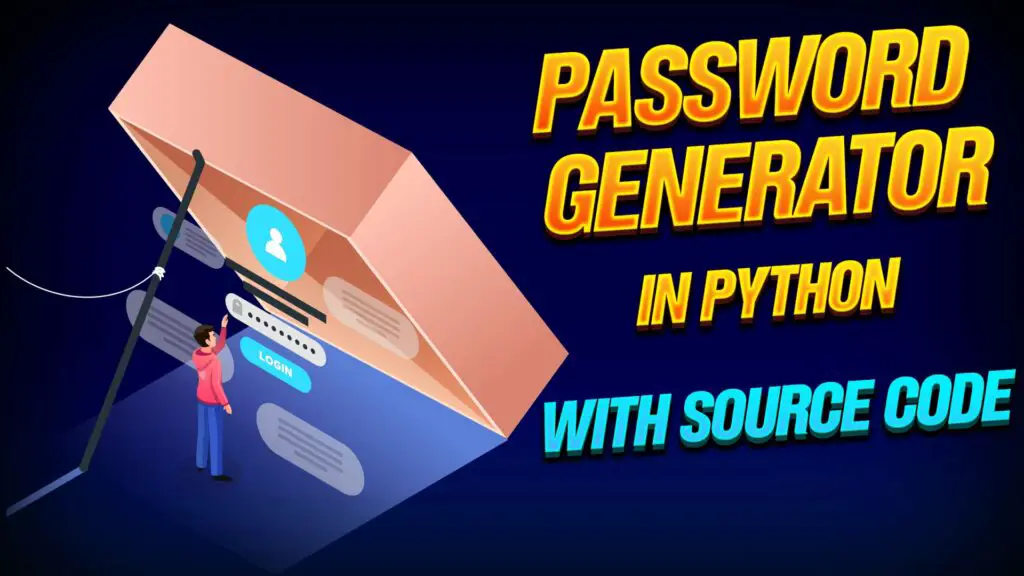
import random
import string
def generate_password(length=12):
# Define character sets for generating the password
characters = string.ascii_letters + string.digits + string.punctuation
# Generate a random password
password = ''.join(random.choice(characters) for _ in range(length))
return password
def main():
print("Welcome to the Password Generator!")
length = int(input("Enter the length of the password (default is 12): "))
password = generate_password(length)
print("Generated Password:", password)
if __name__ == "__main__":
main()
6. Calculator
Develop a Calculator. This project will help you in boosting your logic building.
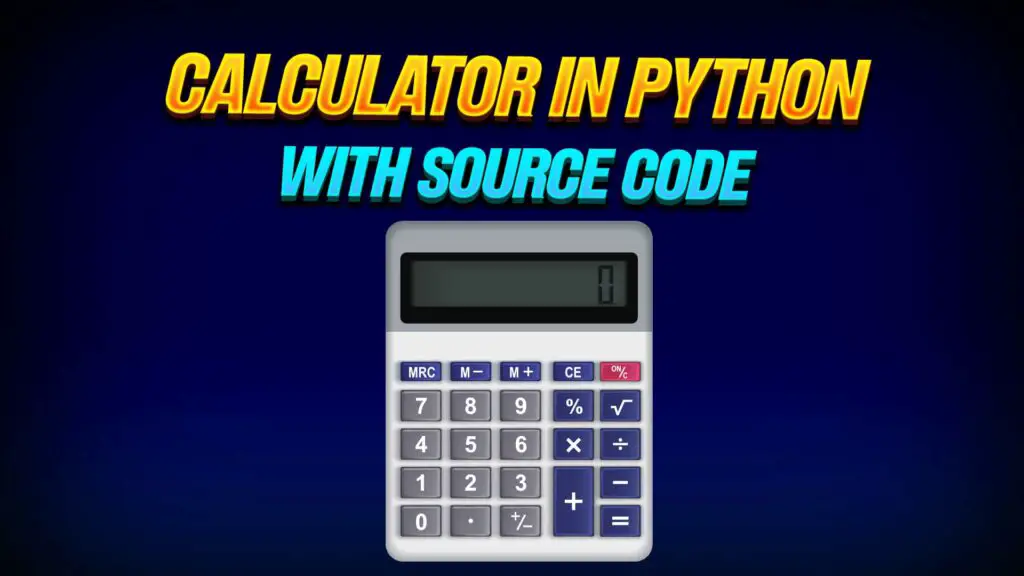
import random
import string
def generate_password(length=12):
# Define character sets for generating the password
characters = string.ascii_letters + string.digits + string.punctuation
# Generate a random password
password = ''.join(random.choice(characters) for _ in range(length))
return password
def main():
print("Welcome to the Password Generator!")
length = int(input("Enter the length of the password (default is 12): "))
password = generate_password(length)
print("Generated Password:", password)
if __name__ == "__main__":
main()
7. Quiz Game
Build a quiz game application that presents users with multiple-choice questions on various topics. Track user scores and provide feedback on correct and incorrect answers.
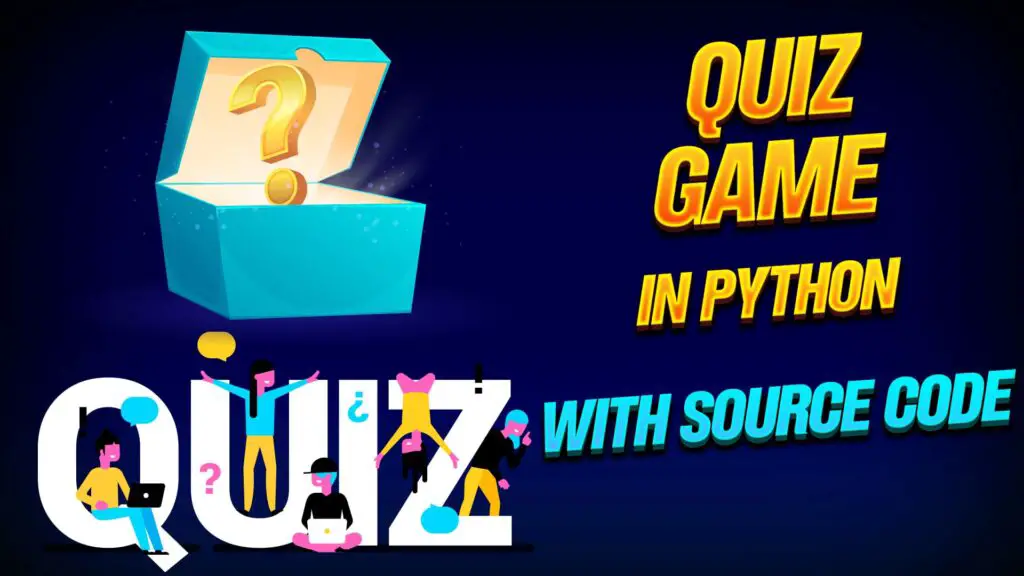
class Question:
def __init__(self, text, choices, answer):
self.text = text
self.choices = choices
self.answer = answer
def check_answer(self, user_answer):
return user_answer.lower() == self.answer.lower()
class Quiz:
def __init__(self, questions):
self.questions = questions
self.score = 0
self.question_index = 0
def get_next_question(self):
current_question = self.questions[self.question_index]
self.question_index += 1
return current_question
def display_question(self, question):
print(question.text)
for idx, choice in enumerate(question.choices, start=1):
print(f"{idx}. {choice}")
def display_score(self):
print(f"Your score: {self.score}/{len(self.questions)}")
def play(self):
for question in self.questions:
self.display_question(question)
user_answer = input("Enter your choice (1, 2, 3, or 4): ")
if question.check_answer(user_answer):
print("Correct!")
self.score += 1
else:
print("Incorrect.")
print()
self.display_score()
# Sample questions
q1 = Question("What is the capital of France?", ["London", "Paris", "Berlin", "Madrid"], "Paris")
q2 = Question("Who wrote 'Romeo and Juliet'?", ["William Shakespeare", "Charles Dickens", "Jane Austen", "Mark Twain"], "William Shakespeare")
q3 = Question("What is the largest planet in our solar system?", ["Earth", "Jupiter", "Mars", "Saturn"], "Jupiter")
questions = [q1, q2, q3]
quiz = Quiz(questions)
quiz.play()
8. Text-based Adventure Game
Design a text-based adventure game where players navigate through a series of rooms, solve puzzles, and make decisions that affect the outcome of the game.
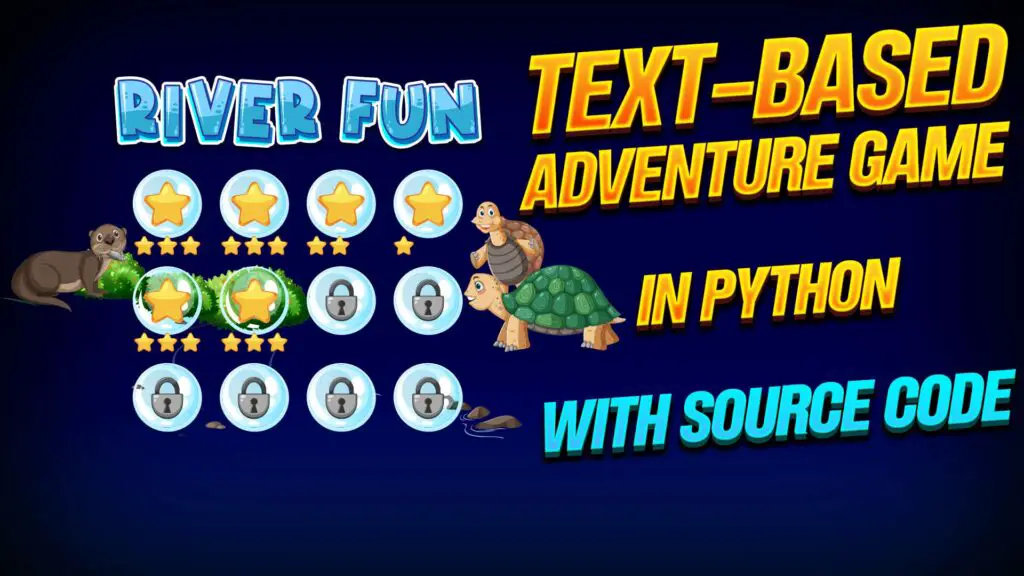
class Room:
def __init__(self, name, description, exits):
self.name = name
self.description = description
self.exits = exits
def display_room(self):
print(f"\n{self.name}")
print(self.description)
print("Exits:", ", ".join(self.exits))
class Player:
def __init__(self, current_room):
self.current_room = current_room
def move(self, direction):
if direction in self.current_room.exits:
self.current_room = rooms[self.current_room.exits[direction]]
print("You move to", self.current_room.name)
else:
print("You cannot go that way.")
# Define rooms
room1 = Room("Room 1", "You are in a small room with a door to the north.", {"north": 1})
room2 = Room("Room 2", "You are in a large hall with exits to the north and east.", {"north": 3, "east": 4})
room3 = Room("Room 3", "You are in a dimly lit corridor. There is an exit to the south.", {"south": 2})
room4 = Room("Room 4", "You are in a dusty library with shelves of old books. There is an exit to the west.", {"west": 2})
# Create dictionary to map room numbers to Room objects
rooms = {
1: room1,
2: room2,
3: room3,
4: room4
}
# Create player object
player = Player(room1)
# Main game loop
while True:
player.current_room.display_room()
command = input("What do you want to do? (Type 'north', 'east', 'south', or 'west' to move, or 'quit' to exit): ").lower()
if command == "quit":
print("Thanks for playing!")
break
elif command in ["north", "east", "south", "west"]:
player.move(command)
else:
print("Invalid command. Please try again.")
9. Chatbot
Create a simple chatbot using natural language processing libraries like NLTK or spaCy. The chatbot should be able to respond to user queries, provide information, and engage in basic conversations.
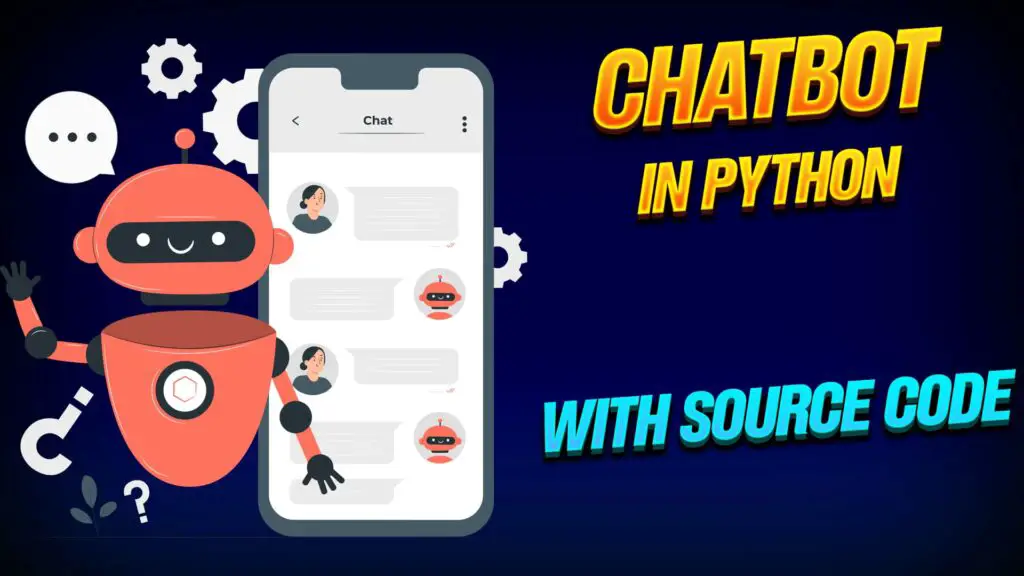
import random
# Define chatbot responses based on patterns
responses = {
"hi": ["Hello!", "Hi there!", "Hey!"],
"how are you": ["I'm good, thank you!", "I'm doing well, thanks for asking!", "All good!"],
"bye": ["Goodbye!", "See you later!", "Bye!"],
"default": ["Sorry, I don't understand.", "Could you please rephrase that?", "I'm not sure I follow."]
}
# Function to generate a response based on user input
def get_response(user_input):
for pattern, responses_list in responses.items():
if pattern in user_input.lower():
return random.choice(responses_list)
return random.choice(responses["default"])
# Main function to run the chatbot
def main():
print("Chatbot: Hi! How can I help you today?")
while True:
user_input = input("You: ")
if user_input.lower() == "exit":
print("Chatbot: Goodbye!")
break
response = get_response(user_input)
print("Chatbot:", response)
if __name__ == "__main__":
main()
10. Music Player
Develop a basic music player application that allows users to play, pause, skip tracks, and create playlists. You can use libraries like Pygame or tkinter for building the user interface.
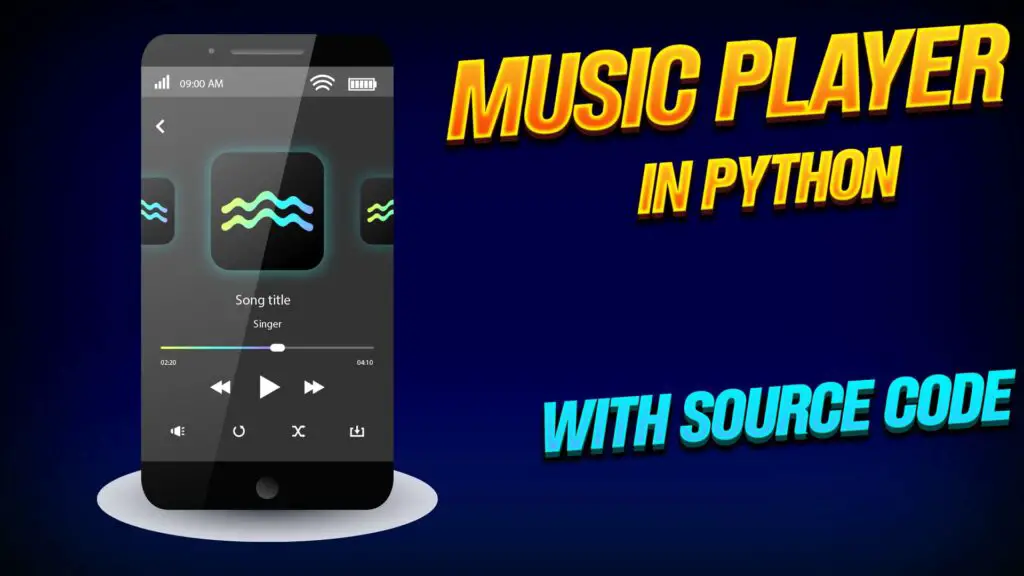
import pygame
import os
def play_music(file_path):
pygame.mixer.init()
pygame.mixer.music.load(file_path)
pygame.mixer.music.play()
def pause_music():
pygame.mixer.music.pause()
def unpause_music():
pygame.mixer.music.unpause()
def stop_music():
pygame.mixer.music.stop()
def main():
print("Welcome to the Python Music Player!")
file_path = input("Enter the path to the music file: ")
# Check if the file exists
if not os.path.exists(file_path):
print("File not found.")
return
play_music(file_path)
while True:
print("\nOptions:")
print("1. Pause")
print("2. Unpause")
print("3. Stop")
print("4. Exit")
choice = input("Enter your choice (1-4): ")
if choice == "1":
pause_music()
elif choice == "2":
unpause_music()
elif choice == "3":
stop_music()
elif choice == "4":
print("Exiting the Python Music Player. Goodbye!")
break
else:
print("Invalid choice. Please enter a number from 1 to 4.")
if __name__ == "__main__":
main()
11. Currency Converter
Build a currency converter tool that converts between different currencies based on real-time exchange rates fetched from a currency API.
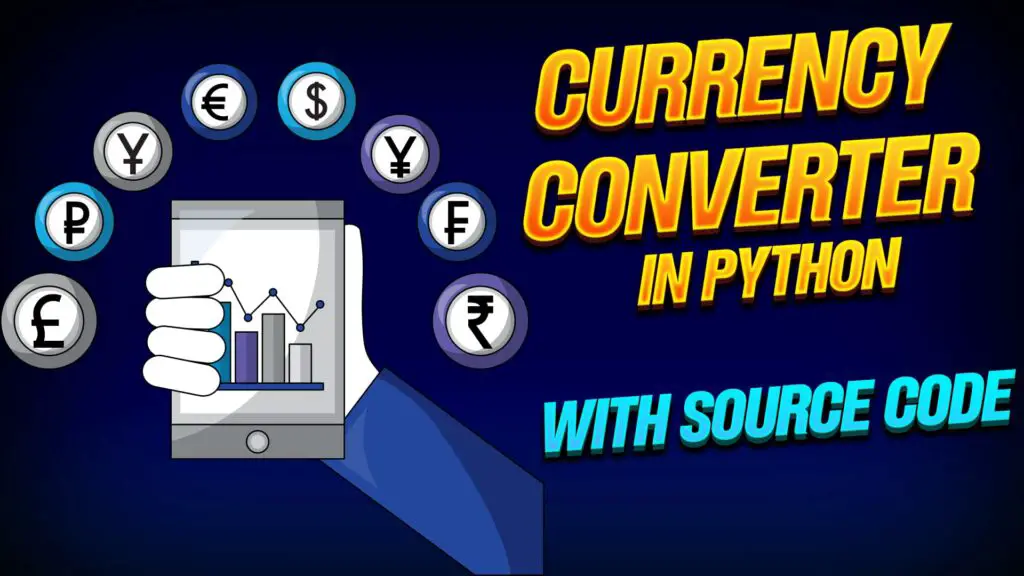
from forex_python.converter import CurrencyRates
def convert_currency(amount, from_currency, to_currency):
c = CurrencyRates()
converted_amount = c.convert(from_currency, to_currency, amount)
return converted_amount
def main():
print("Welcome to the Currency Converter!")
amount = float(input("Enter the amount to convert: "))
from_currency = input("Enter the currency to convert from (e.g., USD): ").upper()
to_currency = input("Enter the currency to convert to (e.g., EUR): ").upper()
converted_amount = convert_currency(amount, from_currency, to_currency)
print(f"{amount} {from_currency} is equal to {converted_amount} {to_currency}")
if __name__ == "__main__":
main()
12. Tic-Tac-Toe Game
Implement the classic Tic-Tac-Toe game where two players take turns marking spaces on a 3×3 grid, with the objective of getting three symbols in a row.
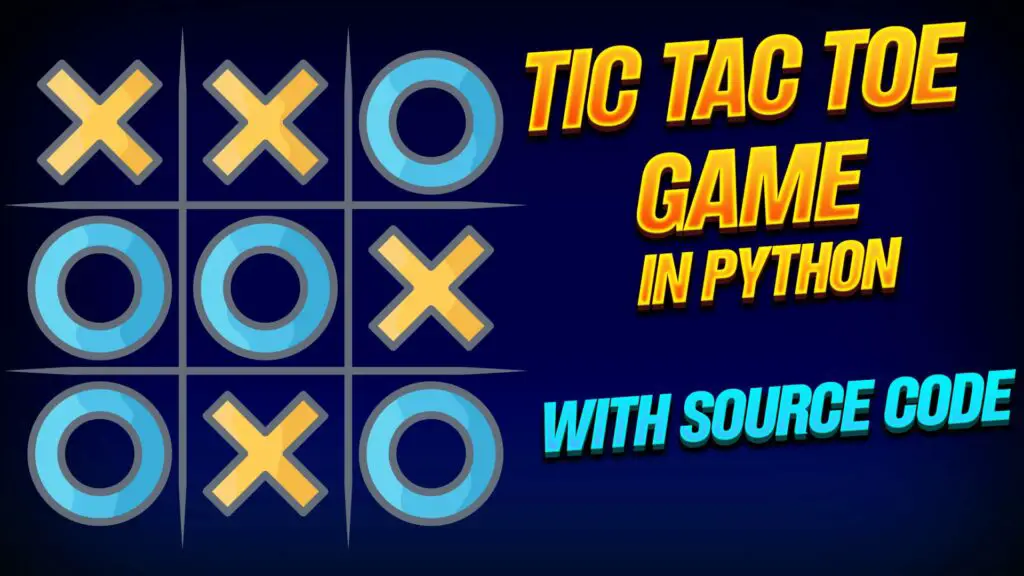
def print_board(board):
for row in board:
print(" | ".join(row))
print("-" * 9)
def check_winner(board):
# Check rows
for row in board:
if row[0] == row[1] == row[2] != " ":
return True, row[0]
# Check columns
for col in range(3):
if board[0][col] == board[1][col] == board[2][col] != " ":
return True, board[0][col]
# Check diagonals
if board[0][0] == board[1][1] == board[2][2] != " ":
return True, board[0][0]
if board[0][2] == board[1][1] == board[2][0] != " ":
return True, board[0][2]
return False, None
def is_board_full(board):
for row in board:
for cell in row:
if cell == " ":
return False
return True
def main():
board = [[" " for _ in range(3)] for _ in range(3)]
player = "X"
print("Welcome to Tic-Tac-Toe!")
print_board(board)
while True:
row = int(input(f"Player {player}, enter row (0, 1, or 2): "))
col = int(input(f"Player {player}, enter column (0, 1, or 2): "))
if board[row][col] != " ":
print("That cell is already taken. Try again.")
continue
board[row][col] = player
print_board(board)
game_over, winner = check_winner(board)
if game_over:
print(f"Player {winner} wins!")
break
elif is_board_full(board):
print("It's a tie!")
break
player = "O" if player == "X" else "X"
if __name__ == "__main__":
main()
13. Hangman Game
Create a Hangman game where players guess letters to uncover a hidden word. Include features like displaying the number of attempts remaining and keeping track of guessed letters.
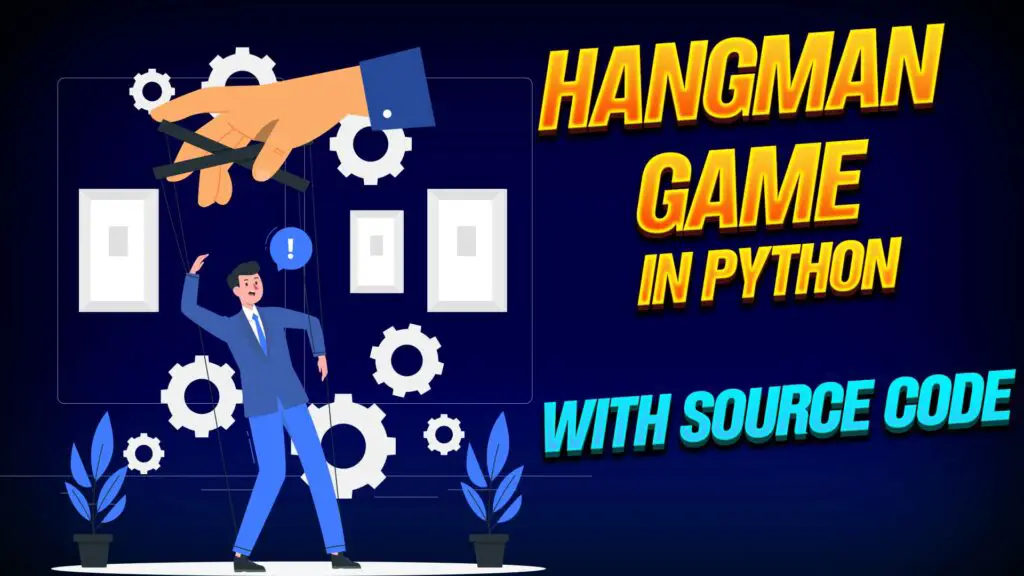
import random
def choose_word():
words = ["python", "java", "ruby", "javascript", "html", "css", "php", "csharp"]
return random.choice(words)
def display_word(word, guessed_letters):
displayed_word = ""
for letter in word:
if letter in guessed_letters:
displayed_word += letter
else:
displayed_word += "_"
return displayed_word
def main():
print("Welcome to Hangman!")
word = choose_word()
guessed_letters = []
attempts = 6
while True:
print("\n" + display_word(word, guessed_letters))
print("Attempts left:", attempts)
guess = input("Enter a letter: ").lower()
if guess in guessed_letters:
print("You've already guessed that letter. Try again.")
continue
guessed_letters.append(guess)
if guess not in word:
attempts -= 1
print("Incorrect guess!")
if attempts == 0:
print("You've run out of attempts! The word was:", word)
break
else:
print("Correct guess!")
if "_" not in display_word(word, guessed_letters):
print("Congratulations! You've guessed the word:", word)
break
if __name__ == "__main__":
main()
14. Contact Book
Design a contact book application that allows users to store and manage contact information, including names, phone numbers, email addresses, and additional notes.
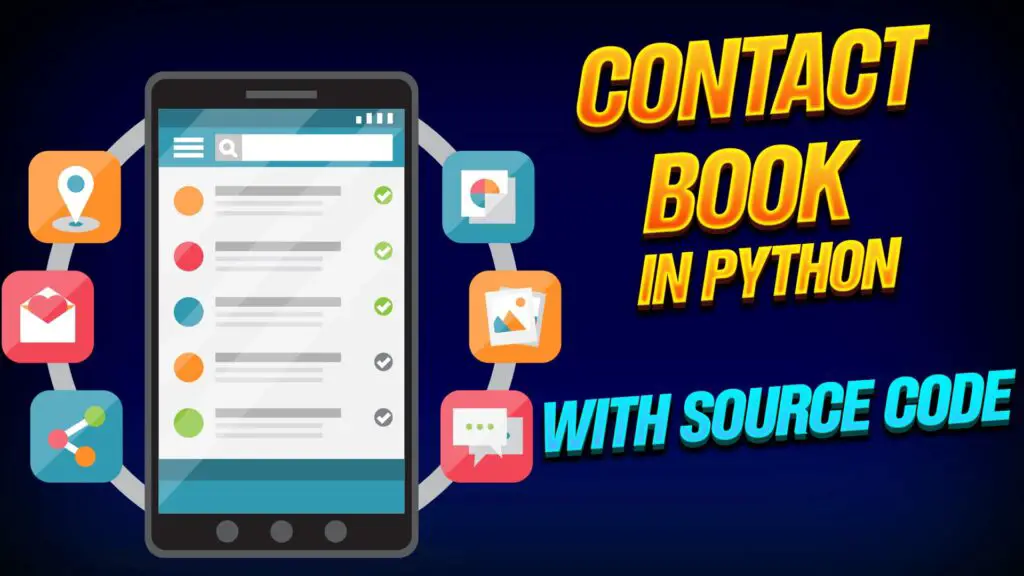
class ContactBook:
def __init__(self):
self.contacts = {}
def add_contact(self, name, phone_number):
self.contacts[name] = phone_number
def remove_contact(self, name):
if name in self.contacts:
del self.contacts[name]
print(f"Contact '{name}' removed successfully.")
else:
print(f"Contact '{name}' not found in the contact book.")
def display_contacts(self):
if self.contacts:
print("Contacts:")
for name, phone_number in self.contacts.items():
print(f"{name}: {phone_number}")
else:
print("Contact book is empty.")
def main():
contact_book = ContactBook()
while True:
print("\nOptions:")
print("1. Add Contact")
print("2. Remove Contact")
print("3. Display Contacts")
print("4. Exit")
choice = input("Enter your choice (1-4): ")
if choice == "1":
name = input("Enter contact name: ")
phone_number = input("Enter contact phone number: ")
contact_book.add_contact(name, phone_number)
elif choice == "2":
name = input("Enter contact name to remove: ")
contact_book.remove_contact(name)
elif choice == "3":
contact_book.display_contacts()
elif choice == "4":
print("Exiting the contact book. Goodbye!")
break
else:
print("Invalid choice. Please enter a number from 1 to 4.")
if __name__ == "__main__":
main()
15. File Encryption/Decryption Tool
Develop a file encryption and decryption tool using cryptographic algorithms like AES or RSA. Users should be able to encrypt sensitive files for secure storage and decrypt them when needed.
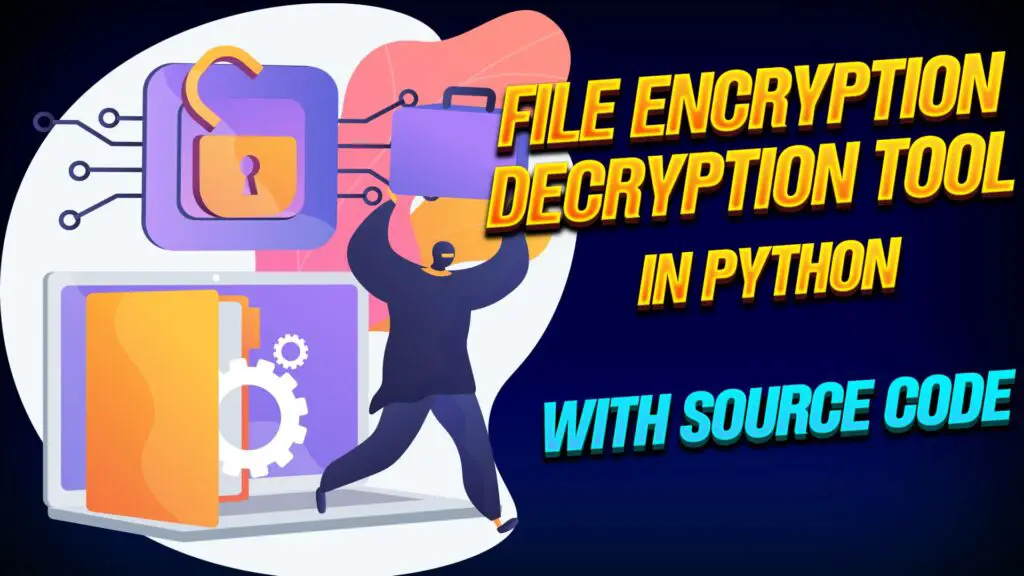
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def write_key_to_file(key, filename="key.key"):
with open(filename, "wb") as key_file:
key_file.write(key)
def load_key_from_file(filename="key.key"):
with open(filename, "rb") as key_file:
return key_file.read()
def encrypt_file(key, input_filename, output_filename=None):
if not output_filename:
output_filename = input_filename + ".enc"
cipher = Fernet(key)
with open(input_filename, "rb") as input_file:
file_data = input_file.read()
encrypted_data = cipher.encrypt(file_data)
with open(output_filename, "wb") as output_file:
output_file.write(encrypted_data)
print(f"File '{input_filename}' encrypted and saved as '{output_filename}'.")
def decrypt_file(key, input_filename, output_filename=None):
if not output_filename:
output_filename = input_filename + ".dec"
cipher = Fernet(key)
with open(input_filename, "rb") as input_file:
encrypted_data = input_file.read()
decrypted_data = cipher.decrypt(encrypted_data)
with open(output_filename, "wb") as output_file:
output_file.write(decrypted_data)
print(f"File '{input_filename}' decrypted and saved as '{output_filename}'.")
def main():
print("Welcome to File Encryption/Decryption Tool!")
key = load_key_from_file()
while True:
print("\nOptions:")
print("1. Encrypt File")
print("2. Decrypt File")
print("3. Exit")
choice = input("Enter your choice (1-3): ")
if choice == "1":
input_filename = input("Enter the name of the file to encrypt: ")
encrypt_file(key, input_filename)
elif choice == "2":
input_filename = input("Enter the name of the file to decrypt: ")
decrypt_file(key, input_filename)
elif choice == "3":
print("Exiting the File Encryption/Decryption Tool. Goodbye!")
break
else:
print("Invalid choice. Please enter a number from 1 to 3.")
if __name__ == "__main__":
main()
16. BMI Calculator
Build a body mass index (BMI) calculator that calculates a person’s BMI based on their height and weight inputs and provides an interpretation of the results (e.g., underweight, normal weight, overweight).
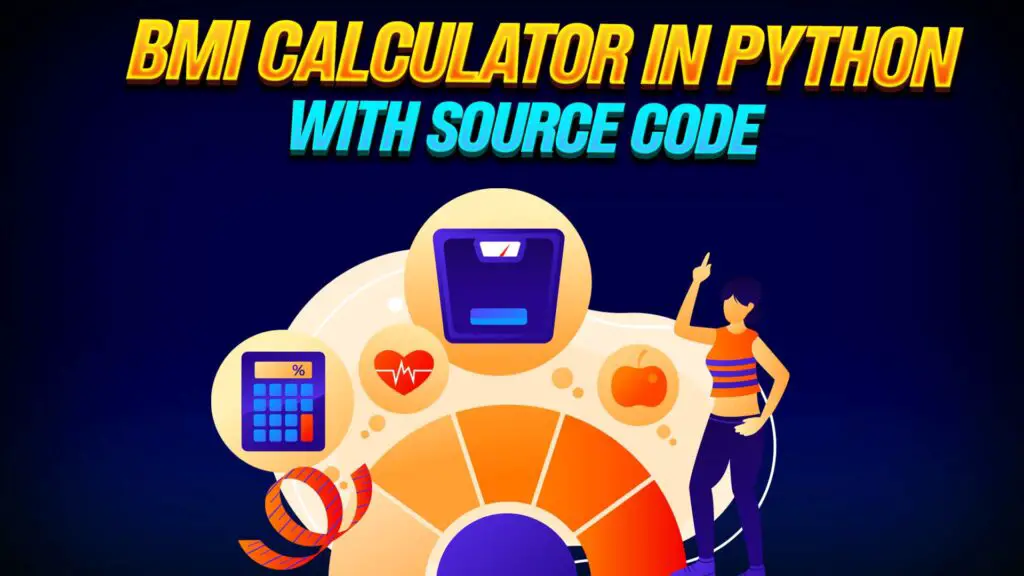
def calculate_bmi(weight, height):
return weight / (height ** 2)
def interpret_bmi(bmi):
if bmi < 18.5:
return "Underweight"
elif 18.5 <= bmi < 25:
return "Normal weight"
elif 25 <= bmi < 30:
return "Overweight"
else:
return "Obese"
def main():
print("Welcome to the BMI Calculator!")
weight = float(input("Enter your weight in kilograms: "))
height = float(input("Enter your height in meters: "))
bmi = calculate_bmi(weight, height)
interpretation = interpret_bmi(bmi)
print(f"Your BMI is {bmi:.2f}, which means you are {interpretation}.")
if __name__ == "__main__":
main()
17. Palindrome Checker
Create a program that checks whether a given word or phrase is a palindrome (reads the same forwards and backward) and provides feedback to the user.
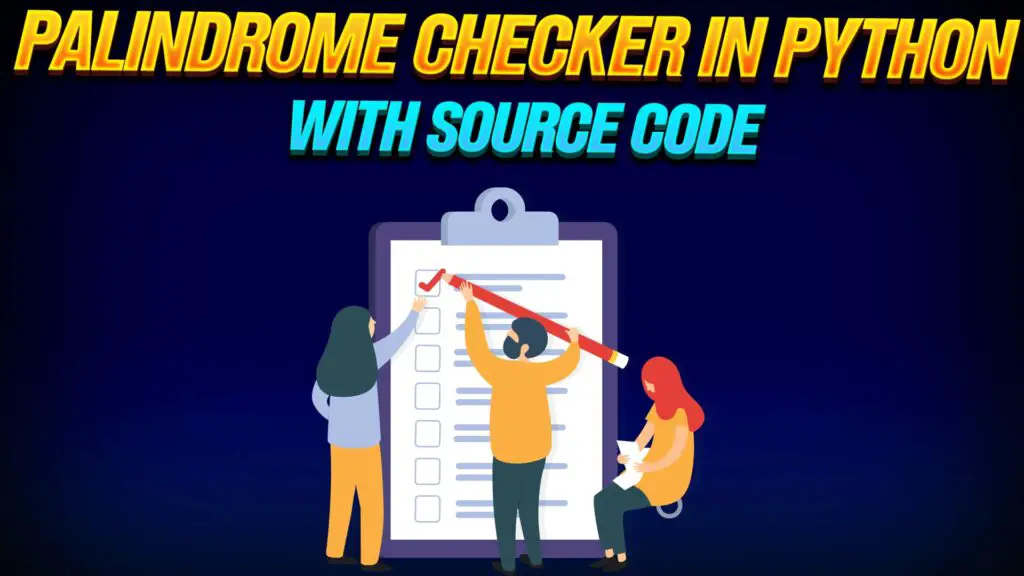
def is_palindrome(s):
s = s.lower() # Convert to lowercase for case-insensitive comparison
s = ''.join(c for c in s if c.isalnum()) # Remove non-alphanumeric characters
return s == s[::-1] # Check if the string is equal to its reverse
def main():
print("Welcome to the Palindrome Checker!")
word = input("Enter a word or phrase: ")
if is_palindrome(word):
print(f"'{word}' is a palindrome.")
else:
print(f"'{word}' is not a palindrome.")
if __name__ == "__main__":
main()
19. Calendar
Design a calendar or planner application that allows users to schedule events, set reminders, and organize their tasks and appointments efficiently.
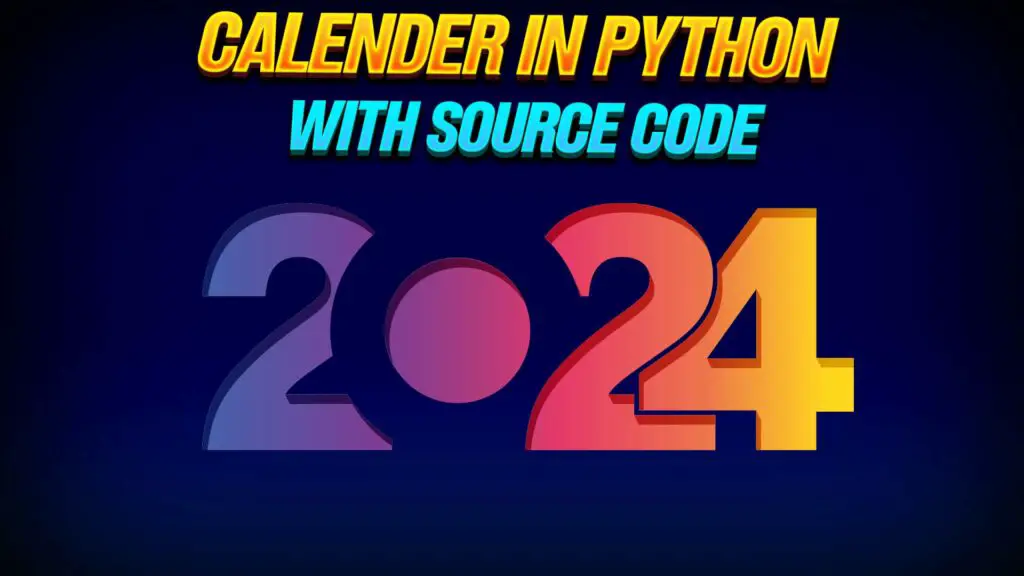
import calendar
def display_calendar(year, month):
cal = calendar.month(year, month)
print(cal)
def main():
print("Welcome to the Calendar!")
year = int(input("Enter the year: "))
month = int(input("Enter the month (1-12): "))
if 1 <= month <= 12:
display_calendar(year, month)
else:
print("Invalid month. Please enter a number from 1 to 12.")
if __name__ == "__main__":
main()
20. Countdown Timer
A countdown timer is a type of timer that counts down from a specified time interval to zero. It is commonly used to track the remaining time until a certain event or deadline.
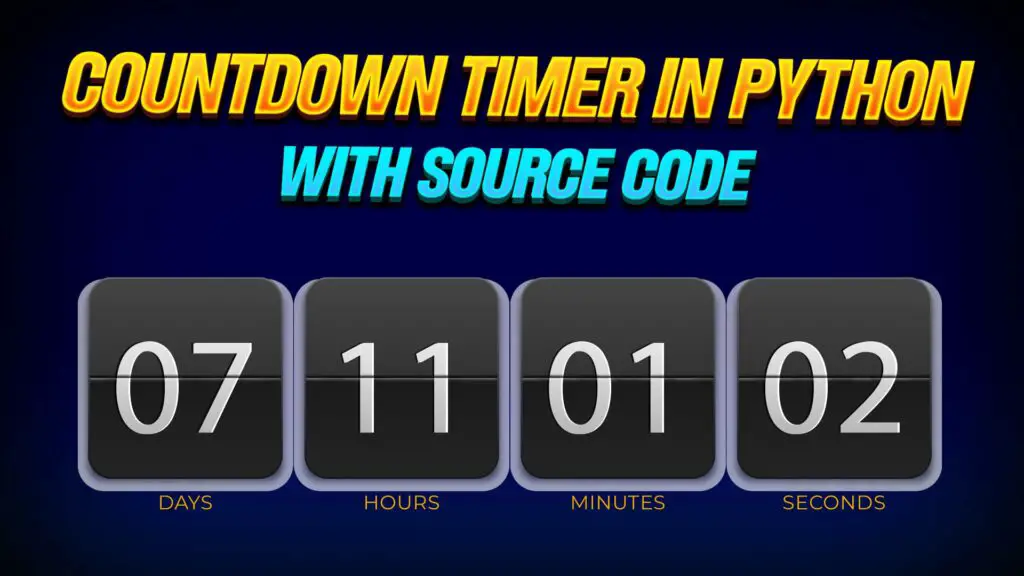
import time
def countdown_timer(seconds):
while seconds > 0:
print(f"Time left: {seconds} seconds", end="\r")
time.sleep(1)
seconds -= 1
print("Time's up!")
def main():
print("Welcome to the Countdown Timer!")
try:
seconds = int(input("Enter the number of seconds to countdown: "))
if seconds < 0:
raise ValueError("Please enter a positive integer.")
countdown_timer(seconds)
except ValueError as e:
print(f"Error: {e}. Please enter a valid positive integer.")
if __name__ == "__main__":
main()
These Python project ideas offer a perfect starting point for beginners to gain hands-on experience, explore different aspects of programming, and unleash their creativity. Choose a project that aligns with your interests and goals, and don’t be afraid to experiment, iterate, and learn along the way. Happy coding!
To Learn More:
Choosing the right Python project depends on your interests, goals, and current skill level. Consider projects that align with your interests or hobbies, as they can be more engaging and motivating. Additionally, start with projects that match your current skill level, but also challenge you to learn new concepts and techniques. Websites like GitHub and Reddit’s r/learn python community are great resources for finding project ideas and getting feedback from other developers.
As a beginner, it’s essential to familiarize yourself with foundational Python libraries and frameworks that are widely used in various domains. Some essential libraries and frameworks for beginners include:
- NumPy: For numerical computing and data manipulation.
- Pandas: For data analysis and manipulation.
- Matplotlib and Seaborn: For data visualization.
- Flask and Django: For web development.
- Beautiful Soup and Scrapy: For web scraping.
- Pygame: For game development. Start with one or two libraries/frameworks that align with your interests and gradually explore others as you gain more experience.
Staying motivated and overcoming challenges is a common concern for beginners. Here are some tips to help you stay on track:
- Break down projects into smaller, manageable tasks.
- Set achievable goals and deadlines for each task.
- Take breaks when you feel stuck or overwhelmed.
- Seek help from online resources, tutorials, documentation, and developer communities.
- Celebrate your progress and accomplishments, no matter how small.
- Remember that getting stuck is part of the learning process, and overcoming challenges will make you a better programmer in the long run.
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
10 Advance Final Year Project Ideas with Source Code
-
Realtime Object Detection
-
E Commerce sales forecasting using machine learning
-
AI Music Composer project with source code
-
Stock market Price Prediction using machine learning
-
30 Final Year Project Ideas for IT Students
-
c++ Projects for beginners
-
Python Projects For Final Year Students With Source Code
-
20 Exiciting Cyber Security Final Year Projects
-
Top 10 Best JAVA Final Year Projects
-
C++ Projects with Source Code
-
Artificial Intelligence Projects For Final Year
-
How to Download image in HTML
-
How to Host HTML website for free?
-
10 Web Development Projects for beginners
-
Hate Speech Detection Using Machine Learning
-
Fake news detection using machine learning source code
-
Credit Card Fraud detection using machine learning