Top 10 ethical hacking projects
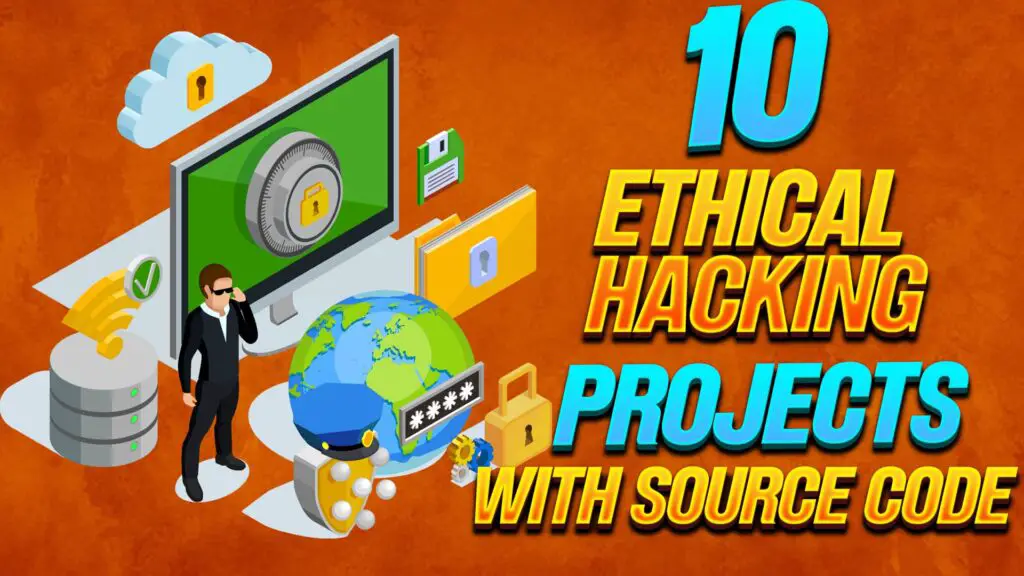
Ethical hacking is a proactive defense strategy where authorized professionals test systems for vulnerabilities before malicious actors misuse them. In this article, we’ll explore ten intriguing ethical hacking projects designed to enhance your skills and contribute positively to the cybersecurity landscape. These projects cover a wide range of ethical hacking activities, from creating viruses for educational purposes to developing phishing website checkers. Let’s dip in and explore each ethical hacking project idea.
1. User Authentication System
User authentication is like a lock that protects sensitive information. It guarantees that only authorized individuals have access to digital spaces. However, the growing complexity of cyber threats makes these systems vulnerable. The User Authentication System ethical hacking project aims to strengthen the security of sensitive information. Developers and security professionals can use ethical hacking principles to build robust authentication systems to withstand cyber threats. This project combines security and ethical practices to provide a safer digital experience for users everywhere.
import sqlite3
import hashlib
import os
def hash_password(password):
salt = os.urandom(32)
key = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return salt + key
def verify_password(username, password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute('SELECT salt, key FROM users WHERE username = ?', (username,))
user = cursor.fetchone()
connection.close()
if user:
salt = user[0]
key = user[1]
hashed_password = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return hashed_password == key
else:
return False
def register(username, password):
try:
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
hashed_password = hash_password(password)
cursor.execute('INSERT INTO users (username, salt, key) VALUES (?, ?, ?)', (username, hashed_password[:32], hashed_password[32:]))
connection.commit()
print(f"User {username} registered successfully!")
except sqlite3.IntegrityError:
print(f"User {username} already exists!")
finally:
connection.close()
def login(username, password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute('SELECT * FROM users WHERE username = ?', (username,))
user = cursor.fetchone()
connection.close()
if user:
if user[2] >= 3:
print("Account locked. Too many failed login attempts.")
else:
salt = user[1]
key = user[2]
hashed_password = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
if hashed_password == key:
print(f"Welcome back, {username}!")
else:
print("Invalid username or password.")
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute('UPDATE users SET attempts = attempts + 1 WHERE username = ?', (username,))
connection.commit()
connection.close()
else:
print("Invalid username or password.")
def change_password(username, old_password, new_password):
if verify_password(username, old_password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
hashed_password = hash_password(new_password)
cursor.execute('UPDATE users SET salt = ?, key = ? WHERE username = ?', (hashed_password[:32], hashed_password[32:], username))
connection.commit()
connection.close()
print(f"Password changed successfully for {username}!")
else:
print("Invalid username or password.")
def reset_password(username, new_password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
hashed_password = hash_password(new_password)
cursor.execute('UPDATE users SET salt = ?, key = ? WHERE username = ?', (hashed_password[:32], hashed_password[32:], username))
connection.commit()
connection.close()
print(f"Password reset successfully for {username}!")
def main():
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
# Create a table to store user credentials
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE NOT NULL,
salt TEXT NOT NULL,
key TEXT NOT NULL,
attempts INTEGER DEFAULT 0
);
''')
connection.commit()
connection.close()
# Register a user
register('alice', 'password123')
# Login with the registered user
login('alice', 'password123')
# Try to register the same user again
register('alice', 'password123')
# Try to login with incorrect credentials
login('alice', 'wrongpassword')
# Change password
change_password('alice', 'password123', 'newpassword456')
# Login with the new password
login('alice', 'newpassword456')
# Reset password
reset_password('alice', 'resetpassword789')
# Login with the reset password
login('alice', 'resetpassword789')
if __name__ == "__main__":
main()
2. Phishing Simulation
Phishing simulation is a way to test and train people to recognize and stop phishing attacks. These attacks trick people into sharing sensitive information by pretending to be trustworthy sources. The project aims to help individuals and organizations understand these tactics and protect against them. The phishing simulation system creates fake phishing emails or messages to imitate real-world scenarios. It includes fake links, deceptive content, and requests for sensitive information. The system tracks user responses to evaluate the effectiveness of training.
import re
import requests
from bs4 import BeautifulSoup
def is_phishing(url):
# Fetch the webpage content
try:
response = requests.get(url)
except Exception as e:
print(f"Error fetching {url}: {e}")
return False
# Check if the URL is using HTTPS
if not url.startswith('https://'):
print("The URL is not using HTTPS.")
return True
# Check if the URL contains an IP address
if re.match(r'^https?://(?:[0-9]{1,3}\.){3}[0-9]{1,3}', url):
print("The URL contains an IP address.")
return True
# Check if the URL is using a free domain
if re.match(r'^https?://(?:www\.)?(?:[a-zA-Z0-9-]+\.)+(?:[a-zA-Z]{2,})', url):
print("The URL is using a free domain.")
return True
# Check if the webpage contains suspicious keywords
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text().lower()
suspicious_keywords = ['login', 'password', 'account', 'credit card', 'social security number']
for keyword in suspicious_keywords:
if keyword in text:
print(f"The webpage contains the keyword '{keyword}'.")
return True
# Check if the webpage contains a form with a POST method
forms = soup.find_all('form')
for form in forms:
if form.get('method', '').lower() == 'post':
print("The webpage contains a form with a POST method.")
return True
# Check if the webpage contains a script tag with an external source
scripts = soup.find_all('script', src=True)
if scripts:
print("The webpage contains a script tag with an external source.")
return True
# Check if the webpage contains an iframe tag with an external source
iframes = soup.find_all('iframe', src=True)
if iframes:
print("The webpage contains an iframe tag with an external source.")
return True
# Check if the webpage contains a link to an external domain
links = soup.find_all('a', href=True)
for link in links:
if not link['href'].startswith('#') and not link['href'].startswith('/'):
print(f"The webpage contains a link to an external domain: {link['href']}")
return True
# If none of the checks return True, the webpage is likely not phishing
print("The webpage is likely not phishing.")
return False
# Example usage
url = 'https://www.google.com'
is_phishing(url)
3. Social Engineering Toolkit (SET)
The Social Engineering Toolkit (SET) is a powerful and dynamic tool for ethical hackers and cybersecurity professionals. SET focuses on social engineering, which manipulates people to share confidential information instead of taking advantage of technological weaknesses like traditional hacking methods. SET helps organizations defend against cybersecurity threats by training ethical hackers to simulate social engineering attacks.
4. Building a Secure File Encryption Tool
In the digital world, it is crucial to protect sensitive information. One way to do this is by using a secure file encryption tool. This tool transforms data into an unreadable format that can only be understood with the correct key. It acts like a digital vault, ensuring only authorized individuals can unlock and access the content. This tool uses robust encryption algorithms to keep the encrypted files secure. Even if someone intercepts the files, they can only crack them with the correct key.
from cryptography.fernet import Fernet
import os
def generate_key():
# Generate a secure encryption key
key = Fernet.generate_key()
with open('encryption_key.key', 'wb') as key_file:
key_file.write(key)
def load_key():
# Load the encryption key from a file
with open('encryption_key.key', 'rb') as key_file:
key = key_file.read()
return key
def encrypt_file(filename):
# Load the encryption key
key = load_key()
fernet = Fernet(key)
# Read the file content
with open(filename, 'rb') as file:
file_data = file.read()
# Encrypt the file content
encrypted_data = fernet.encrypt(file_data)
# Write the encrypted data back to the file
with open(filename, 'wb') as file:
file.write(encrypted_data)
def decrypt_file(filename):
# Load the encryption key
key = load_key()
fernet = Fernet(key)
# Read the encrypted file content
with open(filename, 'rb') as file:
encrypted_data = file.read()
# Decrypt the file content
decrypted_data = fernet.decrypt(encrypted_data)
# Write the decrypted data back to the file
with open(filename, 'wb') as file:
file.write(decrypted_data)
# Example usage
generate_key()
encrypt_file('example.txt')
decrypt_file('example.txt')
5. Image Steganography Program
Image steganography is like a secret agent in the world of digital communication. It involves hiding messages within images, a technique that dates back centuries but has found new relevance in the digital age. Like secret codes in spy movies, steganography lets you send messages without anyone realizing they’re there. The Image Steganography Program takes this concept to the digital realm, offering a creative and covert way to exchange information securely. The Image Steganography Program lets you hide messages inside images without changing the image’s appearance. It uses algorithms to change the pixels in the image to hide the message. You can easily use the program by choosing the image and entering the message you want to hide.
from PIL import Image
def encode_image(image_path, message):
img = Image.open(image_path)
# Convert the message to binary
binary_message = ''.join(format(ord(char), '08b') for char in message)
# Check if the image can hold the message
if len(binary_message) > img.width * img.height * 3:
raise ValueError("Message is too large for the image")
# Encode the message in the image
data_index = 0
for y in range(img.height):
for x in range(img.width):
pixel = list(img.getpixel((x, y)))
for i in range(3):
if data_index < len(binary_message):
pixel[i] = pixel[i] & ~1 | int(binary_message[data_index])
data_index += 1
img.putpixel((x, y), tuple(pixel))
# Save the modified image
img.save('encoded_image.png')
def decode_image(image_path):
img = Image.open(image_path)
binary_message = ''
for y in range(img.height):
for x in range(img.width):
pixel = img.getpixel((x, y))
for i in range(3):
binary_message += str(pixel[i] & 1)
# Convert the binary message to text
message = ''
for i in range(0, len(binary_message), 8):
byte = binary_message[i:i+8]
message += chr(int(byte, 2))
return message
# Example usage
message = "Hello, this is a secret message!"
encode_image('original_image.png', message)
decoded_message = decode_image('encoded_image.png')
print(decoded_message)
6. Cross-Site Request Forgery (CSRF) Exploitation
CSRF is a web security problem where attackers can perform actions on behalf of users without their permission. They exploit the trust between a website and a user’s browser to make harmful requests. Web developers and security professionals must understand how CSRF works and how attackers use it to improve online security. CSRF utilizes the fact that many websites rely on browser cookies to authenticate users. When a user is logged into a site, their browser automatically sends associated cookies to that site with every request. Ethical hackers help identify and address CSRF vulnerabilities by simulating attacks in controlled environments. They work with web developers to enhance security measures and protect against potential exploits.
7. Wi-Fi Password Cracking
Wi-Fi password cracking is about exploring wireless security. Ethical hackers aim to understand weaknesses in Wi-Fi networks. It simply involves deciphering the password that protects a Wi-Fi network. Password cracking is better than it sounds. It is done ethically for educational reasons. Creating a Wi-Fi password-cracking system helps find and fix vulnerabilities in wireless networks. Wi-Fi is widely used, making it a target for attackers. Understanding how passwords can be cracked is crucial for strengthening Wi-Fi security. Ethical hackers can use controlled environments to identify weaknesses and help network administrators enhance defenses for safer wireless communication.
8. Biometric Authentication Implementation
Biometric authentication is a project-based security measure that uses unique physical or behavioral characteristics to verify an individual’s identity. Biometric systems use unique physical characteristics like fingerprints, facial features, or voice patterns instead of traditional methods like passwords or PINs. This technology ensures that only authorized people can access sensitive information or secure areas. Biometric authentication includes using biometric sensors, securely storing templates, and creating accurate matching algorithms. Ethical hackers test the system by trying to bypass it using techniques like fake fingerprints or facial recognition using photos. Their insights help strengthen the system against potential attacks, ensuring that only genuine biometric data is accepted for authentication.
import face_recognition
import cv2
# Load a sample image and learn how to recognize it.
image_of_person = face_recognition.load_image_file("person.jpg")
person_face_encoding = face_recognition.face_encodings(image_of_person)[0]
# Create arrays of known face encodings and their corresponding names
known_face_encodings = [
person_face_encoding,
]
known_face_names = [
"Person",
]
# Initialize some variables
face_locations = []
face_encodings = []
face_names = []
process_this_frame = True
# Get a reference to webcam
video_capture = cv2.VideoCapture(0)
while True:
# Capture frame-by-frame
ret, frame = video_capture.read()
# Resize frame of video to 1/4 size for faster face recognition processing
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# Convert the image from BGR color (which OpenCV uses) to RGB color (which face_recognition uses)
rgb_small_frame = small_frame[:, :, ::-1]
# Only process every other frame of video to save time
if process_this_frame:
# Find all the faces and face encodings in the current frame of video
face_locations = face_recognition.face_locations(rgb_small_frame)
face_encodings = face_recognition.face_encodings(rgb_small_frame, face_locations)
face_names = []
for face_encoding in face_encodings:
# See if the face is a match for the known face(s)
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
# If a match was found in known_face_encodings, just use the first one.
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
face_names.append(name)
process_this_frame = not process_this_frame
# Display the results
for (top, right, bottom, left), name in zip(face_locations, face_names):
# Scale back up face locations since the frame we detected in was scaled to 1/4 size
top *= 4
right *= 4
bottom *= 4
left *= 4
# Draw a box around the face
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
# Draw a label with a name below the face
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 1.0, (255, 255, 255), 1)
# Display the resulting image
cv2.imshow('Video', frame)
# Hit 'q' on the keyboard to quit!
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release handle to the webcam
video_capture.release()
cv2.destroyAllWindows()
9. Threat Intelligence Platform Integration
This ethical hacking project is a powerful tool that collects, analyzes, and responds to cybersecurity threat data. Its primary purpose is to provide essential insights to strengthen defenses against evolving cyber threats. Threat intelligence involves gathering information about potential threats and attackers to understand their strategies and methods. Using a Threat Intelligence Platform makes the process more efficient by providing a central hub to collect and analyze data for proactive cybersecurity measures.
10. Dark Web Monitoring
The dark web is a secret part of the internet where illegal activities happen, and cyber threats are bought and sold. Dark web monitoring is a proactive cybersecurity approach aimed at tracking and analyzing activities in this hidden part. It means watching forums, marketplaces, and encrypted channels for bad actors and using the information to help organizations defend against new cyber threats.
All the projects mentioned are intended for educational and ethical hacking. Ensure that you have proper authorization before conducting any activities in a real-world environment.
Start by learning the basics of networking, programming, and cybersecurity. Familiarize yourself with tools like Wireshark, Nmap, and Kali Linux. Join online communities, attend workshops, and consider pursuing relevant certifications.
While technical skills are crucial, ethical hacking also involves understanding human behavior, social engineering, and legal aspects. A well-rounded ethical hacker combines technical expertise with a deep understanding of cybersecurity.
Ethical hacking helps organizations identify and rectify vulnerabilities before malicious actors can exploit them. By proactively securing systems, ethical hackers contribute to the overall resilience of organizations against cyber threats.
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
10 Advance Final Year Project Ideas with Source Code
-
Realtime Object Detection
-
AI Music Composer project with source code
-
E Commerce sales forecasting using machine learning
-
Stock market Price Prediction using machine learning
-
30 Final Year Project Ideas for IT Students
-
c++ Projects for beginners
-
Python Projects For Final Year Students With Source Code
-
20 Exiciting Cyber Security Final Year Projects
-
Top 10 Best JAVA Final Year Projects
-
C++ Projects with Source Code
-
Artificial Intelligence Projects For Final Year
-
10 Web Development Projects for beginners
-
How to Download image in HTML
-
Fake news detection using machine learning source code
-
How to Host HTML website for free?
-
Hate Speech Detection Using Machine Learning
-
Credit Card Fraud detection using machine learning
-
15 Exciting Blockchain Project Ideas with Source Code
-
Hand Gesture Recognition in python
-
10 advanced JavaScript project ideas for experts in 2024
-
Best Machine Learning Final Year Project
-
Best 21 Projects Using HTML, CSS, Javascript With Source Code
-
Data Science Projects with Source Code
-
Ethical Hacking Projects
-
Plant Disease Detection using Machine Learning
-
Artificial Intelligence Projects for the Final Year
-
20 Advance IOT Projects For Final Year in 2024
-
Top 7 Cybersecurity Final Year Projects in 2024
-
Python Projects For Beginners with Source Code
-
Phishing website detection using Machine Learning with Source Code
-
17 Easy Blockchain Projects For Beginners
-
Top 13 IOT Projects With Source Code
-
portfolio website using javascript
-
Best 13 IOT Project Ideas For Final Year Students
-
10 Exciting Next.jS Project Ideas
-
Fabric Defect Detection
-
Heart Disease Prediction Using Machine Learning
-
How to Change Color of Text in JavaScript
-
Why Creators Choose YouTube: Exploring the Four Key Reasons
-
10 Exciting C++ projects with source code in 2024
-
Wine Quality Prediction Using Machine Learning
-
Diabetes Prediction Using Machine Learning
-
10 Final Year Projects For Computer Science With Source Code
-
Where Do YouTubers Get Their Music?
-
Chronic Kidney Disease Prediction Using Machine Learning
-
10 TypeScript Projects With Source Code
-
Titanic Survival Prediction Using Machine Learning