10 exciting C++ projects with source code
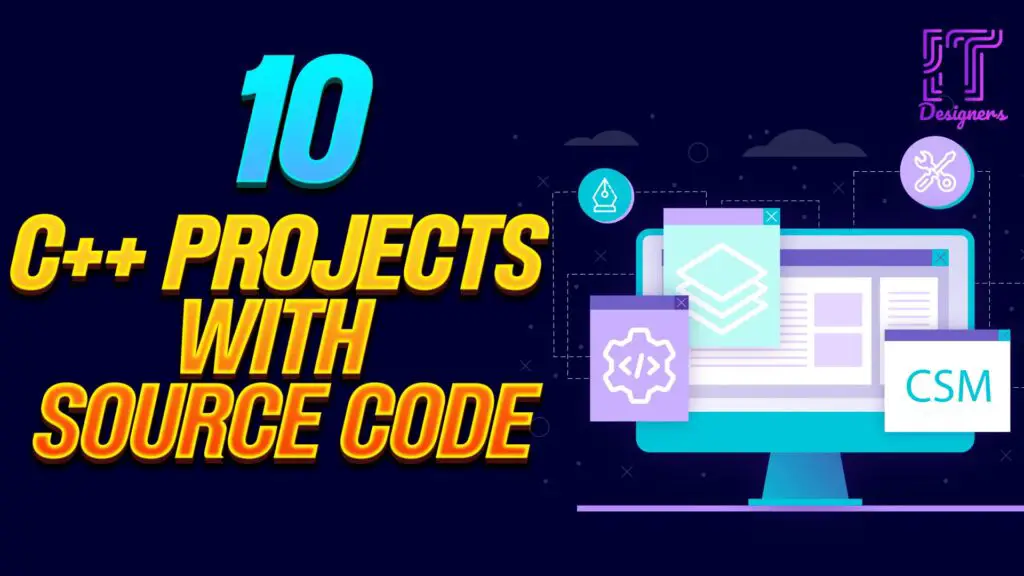
Are you looking for interesting C++ projects to work on? Look no further! This article will look at the top 10 C++ projects with source code. These projects are not only fun to work on, but they also offer excellent learning opportunities. Whether you’re a newbie or an experienced coder, there’s something here for you. Source Code is also available to help you in making these exciting projects.
1. Library management system
The Library Management System aims to improve the library’s organization and retrieval of books. This C++ software lets librarians maintain book records, track borrowing and returning operations, and process user registrations. Users may use a user-friendly interface to browse for books, view availability, and check their borrowing history. Administrators can also generate reports, manage fines, and keep the library running smoothly.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Class to represent a book
class Book {
public:
string title;
string author;
int id;
bool available;
Book(string t, string a, int i) : title(t), author(a), id(i), available(true) {}
};
// Class to represent a user
class User {
public:
string name;
int userId;
User(string n, int id) : name(n), userId(id) {}
};
// Class to manage the library
class Library {
private:
vector<Book> books;
vector<User> users;
public:
void addBook(string title, string author, int id) {
Book book(title, author, id);
books.push_back(book);
}
void addUser(string name, int userId) {
User user(name, userId);
users.push_back(user);
}
void displayBooks() {
cout << "Library Books:" << endl;
for (const Book& book : books) {
cout << "ID: " << book.id << "\tTitle: " << book.title << "\tAuthor: " << book.author;
if (book.available) {
cout << "\tStatus: Available" << endl;
} else {
cout << "\tStatus: Checked Out" << endl;
}
}
}
void displayUsers() {
cout << "Library Users:" << endl;
for (const User& user : users) {
cout << "ID: " << user.userId << "\tName: " << user.name << endl;
}
}
void borrowBook(int userId, int bookId) {
for (Book& book : books) {
if (book.id == bookId && book.available) {
book.available = false;
cout << "Book successfully borrowed by user ID " << userId << "." << endl;
return;
}
}
cout << "Book not available or invalid ID." << endl;
}
void returnBook(int bookId) {
for (Book& book : books) {
if (book.id == bookId && !book.available) {
book.available = true;
cout << "Book successfully returned." << endl;
return;
}
}
cout << "Invalid book ID or book already available." << endl;
}
};
int main() {
Library library;
// Adding some books and users for testing
library.addBook("The Catcher in the Rye", "J.D. Salinger", 1);
library.addBook("To Kill a Mockingbird", "Harper Lee", 2);
library.addBook("1984", "George Orwell", 3);
library.addUser("Alice", 101);
library.addUser("Bob", 102);
// Displaying the initial state of the library
library.displayBooks();
library.displayUsers();
// Simulating book borrowing and returning
library.borrowBook(101, 1);
library.borrowBook(102, 2);
library.returnBook(1);
// Displaying the updated state of the library
library.displayBooks();
library.displayUsers();
return 0;
}
2. Online Exam System
The Online Exam System provides a complete solution for administering exams digitally. Instead of traditional pen-and-paper tests, this system lets students take them on a computer or digital device. The goal is to make the test process more efficient for students and educators.
#include <iostream>
#include <iomanip>
#include <vector>
#include <ctime>
using namespace std;
class Question {
public:
string question;
vector<string> options;
int correctOption;
Question(string q, vector<string> opts, int correct) {
question = q;
options = opts;
correctOption = correct;
}
};
class Exam {
public:
vector<Question> questions;
int totalQuestions;
Exam() {
totalQuestions = 0;
}
void addQuestion(Question q) {
questions.push_back(q);
totalQuestions++;
}
void displayQuestion(int index) {
cout << "Q" << index + 1 << ": " << questions[index].question << endl;
for (size_t i = 0; i < questions[index].options.size(); i++) {
cout << " " << char('A' + i) << ". " << questions[index].options[i] << endl;
}
}
int conductExam() {
int score = 0;
for (int i = 0; i < totalQuestions; i++) {
displayQuestion(i);
char userAnswer;
cout << "Your answer (A/B/C/D): ";
cin >> userAnswer;
int userOption = toupper(userAnswer) - 'A';
if (userOption >= 0 && userOption < questions[i].options.size()) {
if (userOption == questions[i].correctOption) {
cout << "Correct!\n\n";
score++;
} else {
cout << "Incorrect! Correct answer: " << char('A' + questions[i].correctOption) << "\n\n";
}
} else {
cout << "Invalid option!\n\n";
i--;
}
}
return score;
}
};
int main() {
srand(static_cast<unsigned>(time(0)));
Exam exam;
// Sample questions
exam.addQuestion(Question("What is the capital of France?", {"Paris", "Berlin", "London", "Madrid"}, 0));
exam.addQuestion(Question("What is the largest mammal on Earth?", {"Elephant", "Blue Whale", "Giraffe", "Hippopotamus"}, 1));
exam.addQuestion(Question("Who wrote 'Romeo and Juliet'?", {"Charles Dickens", "William Shakespeare", "Jane Austen", "Leo Tolstoy"}, 1));
// Add more questions as needed
cout << "Welcome to the Online Exam System!\n";
cout << "You will be presented with " << exam.totalQuestions << " questions. Good luck!\n\n";
int userScore = exam.conductExam();
cout << "\nYour Exam is over. You scored " << userScore << " out of " << exam.totalQuestions << " questions.\n";
return 0;
}
3. Traffic Management System
The Traffic Management System attempts to improve traffic flow in urban areas. It incorporates features including traffic signal control, vehicle identification, and congestion management. The system continuously monitors traffic conditions and dynamically modifies signal timings. It helps to create a more efficient and ordered urban traffic environment by using algorithms to reduce congestion and prioritize emergency vehicles.
#include <iostream>
#include <thread>
#include <chrono>
#include <vector>
#include <mutex>
using namespace std;
enum TrafficLightColor {
RED,
GREEN,
YELLOW
};
class TrafficLight {
private:
TrafficLightColor color;
mutex mtx;
public:
TrafficLight() : color(RED) {}
void setLight(TrafficLightColor newColor) {
lock_guard<mutex> lock(mtx);
color = newColor;
}
TrafficLightColor getLight() {
lock_guard<mutex> lock(mtx);
return color;
}
};
class Car {
public:
void drive() {
cout << "Car is driving." << endl;
}
void stop() {
cout << "Car has stopped." << endl;
}
};
class TrafficManager {
private:
TrafficLight trafficLight;
vector<Car> cars;
public:
void addCar(const Car& car) {
cars.push_back(car);
}
void simulateTraffic() {
for (int i = 0; i < 10; ++i) {
this_thread::sleep_for(chrono::seconds(1));
TrafficLightColor currentColor = trafficLight.getLight();
if (currentColor == RED) {
cout << "Traffic Light: RED" << endl;
for (auto& car : cars) {
car.stop();
}
trafficLight.setLight(GREEN);
} else if (currentColor == GREEN) {
cout << "Traffic Light: GREEN" << endl;
for (auto& car : cars) {
car.drive();
}
trafficLight.setLight(YELLOW);
} else if (currentColor == YELLOW) {
cout << "Traffic Light: YELLOW" << endl;
// Yellow duration for caution
this_thread::sleep_for(chrono::seconds(1));
trafficLight.setLight(RED);
}
}
}
};
int main() {
Car car1, car2;
TrafficManager trafficManager;
trafficManager.addCar(car1);
trafficManager.addCar(car2);
thread trafficSimulation(&TrafficManager::simulateTraffic, &trafficManager);
trafficSimulation.join();
return 0;
}
4. Smart Home Automation System
A Smart Home Automation System is similar to having a personal assistant for your home. This technology allows you to operate various household equipment and appliances remotely. Think about being able to turn on the lights, adjust the thermostat, and even lock the doors from anywhere using your smartphone or computer. This system improves your home’s convenience, efficiency, and security.
#include <iostream>
#include <vector>
#include <string>
// Simulated Device Interface
class Device {
public:
virtual void turnOn() = 0;
virtual void turnOff() = 0;
virtual std::string getName() const = 0;
};
// Simulated Light Device
class Light : public Device {
public:
Light(const std::string& name) : name(name), status(false) {}
void turnOn() override {
status = true;
std::cout << name << " light turned ON.\n";
}
void turnOff() override {
status = false;
std::cout << name << " light turned OFF.\n";
}
std::string getName() const override {
return name;
}
private:
std::string name;
bool status;
};
// Simulated Thermostat Device
class Thermostat : public Device {
public:
Thermostat(const std::string& name) : name(name), temperature(22.0) {}
void turnOn() override {
std::cout << name << " thermostat turned ON.\n";
}
void turnOff() override {
std::cout << name << " thermostat turned OFF.\n";
}
void setTemperature(double temp) {
temperature = temp;
std::cout << name << " thermostat set to " << temperature << " degrees Celsius.\n";
}
std::string getName() const override {
return name;
}
private:
std::string name;
double temperature;
};
// Smart Home Controller
class SmartHome {
public:
void addDevice(Device* device) {
devices.push_back(device);
}
void operateDevices() {
for (const auto& device : devices) {
device->turnOn();
device->turnOff();
}
}
Device* findDeviceByName(const std::string& name) const {
for (const auto& device : devices) {
if (device->getName() == name) {
return device;
}
}
return nullptr;
}
private:
std::vector<Device*> devices;
};
int main() {
// Simulated Devices
Light livingRoomLight("Living Room");
Light kitchenLight("Kitchen");
Thermostat livingRoomThermostat("Living Room");
// Smart Home Initialization
SmartHome mySmartHome;
mySmartHome.addDevice(&livingRoomLight);
mySmartHome.addDevice(&kitchenLight);
mySmartHome.addDevice(&livingRoomThermostat);
// Operate Devices
mySmartHome.operateDevices();
// Find and Operate a Specific Device
Device* foundDevice = mySmartHome.findDeviceByName("Kitchen");
if (foundDevice) {
foundDevice->turnOn();
} else {
std::cout << "Device not found.\n";
}
return 0;
}
5. Employee Record System
An Employee Record System functions similarly to a digital filing cabinet, storing all critical employee information. Before Employee Record Systems, firms needed help to keep track of all of this information. They had to rely on paper files and manual processes, which could have been more efficient, were prone to errors, and took up a lot of space. Furthermore, it wasn’t easy to find information quickly when required. So, to make things easier and more structured, organizations started using employee record systems.
#include <iostream>
#include <vector>
#include <algorithm>
class Employee {
public:
Employee(int id, const std::string& name, double salary)
: id(id), name(name), salary(salary) {}
int getId() const {
return id;
}
std::string getName() const {
return name;
}
double getSalary() const {
return salary;
}
private:
int id;
std::string name;
double salary;
};
class EmployeeRecordSystem {
public:
void addEmployee(int id, const std::string& name, double salary) {
employees.emplace_back(id, name, salary);
std::cout << "Employee added successfully!\n";
}
void displayEmployeeDetails() const {
if (employees.empty()) {
std::cout << "No employees in the record.\n";
} else {
std::cout << "Employee Details:\n";
for (const auto& employee : employees) {
std::cout << "ID: " << employee.getId()
<< "\tName: " << employee.getName()
<< "\tSalary: $" << employee.getSalary() << "\n";
}
}
}
void searchEmployeeById(int searchId) const {
auto it = std::find_if(employees.begin(), employees.end(),
[searchId](const Employee& e) { return e.getId() == searchId; });
if (it != employees.end()) {
std::cout << "Employee found!\n";
std::cout << "ID: " << it->getId()
<< "\tName: " << it->getName()
<< "\tSalary: $" << it->getSalary() << "\n";
} else {
std::cout << "Employee not found.\n";
}
}
private:
std::vector<Employee> employees;
};
int main() {
EmployeeRecordSystem recordSystem;
// Adding employees
recordSystem.addEmployee(1, "John Doe", 50000.0);
recordSystem.addEmployee(2, "Jane Doe", 60000.0);
// Displaying employee details
recordSystem.displayEmployeeDetails();
// Searching for an employee by ID
recordSystem.searchEmployeeById(1);
return 0;
}
6. Hotel Management System
Developing a Hotel Management System is a complete software solution that simplifies and automates various hotel and hospitality processes. This system is designed to make visitor reservations, room management, billing, and overall administration more efficient. The Hotel Management System improves efficiency, increases guest experiences, and allows for easy day-to-day hotel operations through technology.
#include <iostream>
#include <vector>
#include <string>
class Room {
public:
Room(int roomNumber, double price, bool isOccupied = false)
: roomNumber(roomNumber), price(price), isOccupied(isOccupied) {}
int getRoomNumber() const {
return roomNumber;
}
double getPrice() const {
return price;
}
bool getIsOccupied() const {
return isOccupied;
}
void occupyRoom() {
isOccupied = true;
}
void vacateRoom() {
isOccupied = false;
}
private:
int roomNumber;
double price;
bool isOccupied;
};
class Guest {
public:
Guest(const std::string& name, const std::string& phoneNumber)
: name(name), phoneNumber(phoneNumber) {}
std::string getName() const {
return name;
}
std::string getPhoneNumber() const {
return phoneNumber;
}
private:
std::string name;
std::string phoneNumber;
};
class Reservation {
public:
Reservation(const Guest& guest, const Room& room, int nights)
: guest(guest), room(room), nights(nights) {}
double getTotalCost() const {
return room.getPrice() * nights;
}
private:
Guest guest;
Room room;
int nights;
};
class HotelManagementSystem {
public:
void addRoom(const Room& room) {
rooms.push_back(room);
}
void displayAvailableRooms() const {
std::cout << "Available Rooms:\n";
for (const auto& room : rooms) {
if (!room.getIsOccupied()) {
std::cout << "Room " << room.getRoomNumber() << "\tPrice: $" << room.getPrice() << "\n";
}
}
}
Reservation bookRoom(const Guest& guest, int roomNumber, int nights) {
auto it = std::find_if(rooms.begin(), rooms.end(),
[roomNumber](const Room& r) { return r.getRoomNumber() == roomNumber && !r.getIsOccupied(); });
if (it != rooms.end()) {
it->occupyRoom();
std::cout << "Room " << it->getRoomNumber() << " booked successfully.\n";
return Reservation(guest, *it, nights);
} else {
throw std::runtime_error("Selected room is not available or doesn't exist.");
}
}
private:
std::vector<Room> rooms;
};
int main() {
HotelManagementSystem hotel;
// Adding rooms
hotel.addRoom(Room(101, 100.0));
hotel.addRoom(Room(102, 120.0));
hotel.addRoom(Room(103, 150.0));
// Displaying available rooms
hotel.displayAvailableRooms();
// Booking a room
Guest guest("John Doe", "123-456-7890");
try {
Reservation reservation = hotel.bookRoom(guest, 102, 3);
std::cout << "Total Cost: $" << reservation.getTotalCost() << "\n";
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << "\n";
}
return 0;
}
7. Supermarket Billing System
The Supermarket Billing System, which employs C++, simplifies the billing process in a supermarket. In the fast-paced retail environment of supermarkets, efficient and accurate invoicing is critical for both customers and shop management. This technology is designed to simplify the checkout process, improve accuracy, and increase overall customer happiness.Â
#include <iostream>
#include <iomanip>
#include <vector>
#include <string>
// Product class to represent items in the supermarket
class Product {
public:
Product(const std::string& name, double price) : name(name), price(price) {}
const std::string& getName() const {
return name;
}
double getPrice() const {
return price;
}
private:
std::string name;
double price;
};
// ShoppingCart class to handle customer's items and calculate the total amount
class ShoppingCart {
public:
void addItem(const Product& product, int quantity) {
items.push_back({ product, quantity });
}
void displayReceipt() const {
std::cout << "===== Receipt =====\n";
double totalAmount = 0.0;
for (const auto& item : items) {
const Product& product = item.first;
int quantity = item.second;
double itemTotal = product.getPrice() * quantity;
totalAmount += itemTotal;
std::cout << product.getName() << " x " << quantity << " = $" << std::fixed << std::setprecision(2) << itemTotal << "\n";
}
std::cout << "-------------------\n";
std::cout << "Total Amount: $" << std::fixed << std::setprecision(2) << totalAmount << "\n";
}
private:
std::vector<std::pair<Product, int>> items;
};
int main() {
// Sample products
Product apple("Apple", 1.0);
Product banana("Banana", 0.5);
Product milk("Milk", 2.0);
// Create a shopping cart
ShoppingCart cart;
// Customer adds items to the cart
cart.addItem(apple, 3);
cart.addItem(banana, 2);
cart.addItem(milk, 1);
// Display the receipt
cart.displayReceipt();
return 0;
}
8. Pharmacy Management System
Pharmacies often face issues with inventory management, prescription processing, and manual customer record keeping. These issues might result in errors, delays, and inefficiencies when serving patients. The Pharmacy Management System tackles these difficulties by automating and centralizing tasks, automating the entire process from medicine supply to customer checkout, enhancing service quality, and lowering the chance of error.
#include <iostream>
#include <vector>
#include <iomanip>
#include <algorithm>
class Medicine {
public:
Medicine(const std::string& name, double price, int quantity)
: name(name), price(price), quantity(quantity) {}
const std::string& getName() const {
return name;
}
double getPrice() const {
return price;
}
int getQuantity() const {
return quantity;
}
void updateQuantity(int delta) {
quantity += delta;
}
private:
std::string name;
double price;
int quantity;
};
class Pharmacy {
public:
void addMedicine(const Medicine& medicine) {
medicines.push_back(medicine);
}
void displayMedicines() const {
std::cout << "===== Medicines in Stock =====\n";
for (const auto& medicine : medicines) {
std::cout << medicine.getName() << " | Price: $" << std::fixed << std::setprecision(2) << medicine.getPrice()
<< " | Quantity: " << medicine.getQuantity() << "\n";
}
}
bool sellMedicine(const std::string& medicineName, int quantity) {
auto it = std::find_if(medicines.begin(), medicines.end(),
[medicineName](const Medicine& m) { return m.getName() == medicineName; });
if (it != medicines.end() && it->getQuantity() >= quantity) {
it->updateQuantity(-quantity);
return true;
}
return false;
}
private:
std::vector<Medicine> medicines;
};
int main() {
// Sample Medicines
Medicine paracetamol("Paracetamol", 5.0, 100);
Medicine aspirin("Aspirin", 3.5, 50);
Medicine ibuprofen("Ibuprofen", 7.0, 75);
// Create Pharmacy
Pharmacy pharmacy;
pharmacy.addMedicine(paracetamol);
pharmacy.addMedicine(aspirin);
pharmacy.addMedicine(ibuprofen);
// Display Medicines
pharmacy.displayMedicines();
// Sell Medicines
std::string medicineToSell;
int quantityToSell;
std::cout << "\nEnter medicine name to sell: ";
std::cin >> medicineToSell;
std::cout << "Enter quantity to sell: ";
std::cin >> quantityToSell;
if (pharmacy.sellMedicine(medicineToSell, quantityToSell)) {
std::cout << "Sale Successful!\n";
} else {
std::cout << "Sale Failed. Insufficient quantity or medicine not found.\n";
}
// Display Updated Medicines
pharmacy.displayMedicines();
return 0;
}
9. Cafeteria Order Management System
Create a cafeteria order management. This System is a convenient and efficient solution for ordering and managing food in a cafeteria.
#include <iostream>
#include <vector>
#include <iomanip>
#include <algorithm>
class MenuItem {
public:
MenuItem(const std::string& name, double price) : name(name), price(price) {}
const std::string& getName() const {
return name;
}
double getPrice() const {
return price;
}
private:
std::string name;
double price;
};
class Order {
public:
void addItem(const MenuItem& item, int quantity) {
items.push_back({ item, quantity });
}
double calculateTotalCost() const {
double totalCost = 0.0;
for (const auto& item : items) {
totalCost += item.first.getPrice() * item.second;
}
return totalCost;
}
void displayOrder() const {
std::cout << "===== Order Details =====\n";
for (const auto& item : items) {
std::cout << item.first.getName() << " x " << item.second << " = $"
<< std::fixed << std::setprecision(2) << item.first.getPrice() * item.second << "\n";
}
std::cout << "--------------------------\n";
std::cout << "Total Cost: $" << std::fixed << std::setprecision(2) << calculateTotalCost() << "\n";
}
private:
std::vector<std::pair<MenuItem, int>> items;
};
class Cafeteria {
public:
void addMenuItem(const MenuItem& item) {
menu.push_back(item);
}
void displayMenu() const {
std::cout << "===== Menu =====\n";
for (const auto& item : menu) {
std::cout << item.getName() << " | Price: $" << std::fixed << std::setprecision(2) << item.getPrice() << "\n";
}
}
bool placeOrder(Order& order) {
std::string itemName;
int quantity;
std::cout << "Enter item name to add to the order (type 'done' to finish): ";
std::cin >> itemName;
while (itemName != "done") {
auto it = std::find_if(menu.begin(), menu.end(),
[itemName](const MenuItem& m) { return m.getName() == itemName; });
if (it != menu.end()) {
std::cout << "Enter quantity: ";
std::cin >> quantity;
order.addItem(*it, quantity);
} else {
std::cout << "Item not found in the menu.\n";
}
std::cout << "Enter another item name or 'done' to finish: ";
std::cin >> itemName;
}
return !order.calculateTotalCost(); // Returns false if the order is empty
}
private:
std::vector<MenuItem> menu;
};
int main() {
// Sample Menu Items
MenuItem coffee("Coffee", 2.5);
MenuItem sandwich("Sandwich", 5.0);
MenuItem salad("Salad", 3.75);
// Create Cafeteria
Cafeteria cafeteria;
cafeteria.addMenuItem(coffee);
cafeteria.addMenuItem(sandwich);
cafeteria.addMenuItem(salad);
// Display Menu
cafeteria.displayMenu();
// Place Order
Order customerOrder;
if (!cafeteria.placeOrder(customerOrder)) {
std::cout << "Order is empty. Exiting.\n";
return 0;
}
// Display Order
customerOrder.displayOrder();
return 0;
}
10. Hostel Accommodation System
The Hostel Accommodation System is a digital organizer for managing living rooms at schools and universities. It keeps track of who stays where for how long and provides a comfortable and secure student environment. The System is a comprehensive system that simplifies and manages the processes involved in hostel room distribution and management.
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
class Student {
public:
Student(const std::string& name, int rollNumber)
: name(name), rollNumber(rollNumber), allocatedRoomNumber(-1) {}
const std::string& getName() const {
return name;
}
int getRollNumber() const {
return rollNumber;
}
int getAllocatedRoomNumber() const {
return allocatedRoomNumber;
}
void allocateRoom(int roomNumber) {
allocatedRoomNumber = roomNumber;
}
private:
std::string name;
int rollNumber;
int allocatedRoomNumber;
};
class Hostel {
public:
Hostel(int totalRooms) : totalRooms(totalRooms), availableRooms(totalRooms) {
for (int i = 1; i <= totalRooms; ++i) {
availableRooms.push_back(i);
}
}
void displayAvailableRooms() const {
std::cout << "===== Available Rooms =====\n";
for (int room : availableRooms) {
std::cout << "Room " << room << "\n";
}
}
bool allocateRoom(Student& student) {
if (availableRooms.empty()) {
std::cout << "No available rooms.\n";
return false;
}
int roomNumber = availableRooms.back();
availableRooms.pop_back();
student.allocateRoom(roomNumber);
allocatedStudents.push_back(student);
std::cout << "Room allocated successfully. Student " << student.getName() << " assigned to Room " << roomNumber << "\n";
return true;
}
void displayAllocatedRooms() const {
std::cout << "===== Allocated Rooms =====\n";
for (const auto& student : allocatedStudents) {
std::cout << "Student " << student.getName() << " | Roll Number: " << student.getRollNumber()
<< " | Room: " << student.getAllocatedRoomNumber() << "\n";
}
}
bool deallocateRoom(int rollNumber) {
auto it = std::find_if(allocatedStudents.begin(), allocatedStudents.end(),
[rollNumber](const Student& student) { return student.getRollNumber() == rollNumber; });
if (it != allocatedStudents.end()) {
int roomNumber = it->getAllocatedRoomNumber();
availableRooms.push_back(roomNumber);
allocatedStudents.erase(it);
std::cout << "Room deallocated successfully. Student " << it->getName() << " removed from Room " << roomNumber << "\n";
return true;
}
std::cout << "Student not found or not allocated a room.\n";
return false;
}
private:
int totalRooms;
std::vector<int> availableRooms;
std::vector<Student> allocatedStudents;
};
int main() {
// Create Hostel with 10 rooms
Hostel hostel(10);
// Add students
Student student1("John Doe", 101);
Student student2("Jane Smith", 102);
// Allocate rooms
hostel.allocateRoom(student1);
hostel.allocateRoom(student2);
// Display allocated rooms
hostel.displayAllocatedRooms();
// Deallocate a room
hostel.deallocateRoom(student1.getRollNumber());
// Display updated allocated rooms
hostel.displayAllocatedRooms();
return 0;
}
Do not get disheartened! Programming is all about problem-solving. If you run into challenges, divide the problem into smaller jobs, review resources, and seek assistance from peers or online forums. With perseverance and determination, you are going to overcome any challenges.
Absolutely! Feel free to customize these projects according to your needs. Experiment with new features, improve existing functionalities, and make them your own.
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
10 Advance Final Year Project Ideas with Source Code
-
Realtime Object Detection
-
E Commerce sales forecasting using machine learning
-
AI Music Composer project with source code
-
Stock market Price Prediction using machine learning
-
30 Final Year Project Ideas for IT Students
-
c++ Projects for beginners
-
Python Projects For Final Year Students With Source Code
-
20 Exiciting Cyber Security Final Year Projects
-
Top 10 Best JAVA Final Year Projects
-
C++ Projects with Source Code
-
Artificial Intelligence Projects For Final Year
-
How to Host HTML website for free?
-
How to Download image in HTML
-
Hate Speech Detection Using Machine Learning
-
10 Web Development Projects for beginners
-
Fake news detection using machine learning source code
-
Credit Card Fraud detection using machine learning
-
Best Machine Learning Final Year Project
-
15 Exciting Blockchain Project Ideas with Source Code
-
10 advanced JavaScript project ideas for experts in 2024
-
Best 21 Projects Using HTML, CSS, Javascript With Source Code
-
Hand Gesture Recognition in python
-
Data Science Projects with Source Code
-
Ethical Hacking Projects
-
20 Advance IOT Projects For Final Year in 2024
-
Python Projects For Beginners with Source Code
-
Top 7 Cybersecurity Final Year Projects in 2024
-
Phishing website detection using Machine Learning with Source Code
-
Artificial Intelligence Projects for the Final Year
-
17 Easy Blockchain Projects For Beginners
-
Plant Disease Detection using Machine Learning
-
portfolio website using javascript
-
Top 13 IOT Projects With Source Code
-
Fabric Defect Detection
-
Heart Disease Prediction Using Machine Learning
-
Best 13 IOT Project Ideas For Final Year Students
-
10 Exciting Next.jS Project Ideas
-
How to Change Color of Text in JavaScript
-
10 Exciting C++ projects with source code in 2024
-
Wine Quality Prediction Using Machine Learning
-
Diabetes Prediction Using Machine Learning
-
Maize Leaf Disease Detection
-
Why Creators Choose YouTube: Exploring the Four Key Reasons
-
Chronic Kidney Disease Prediction Using Machine Learning
-
10 Final Year Projects For Computer Science With Source Code
-
Titanic Survival Prediction Using Machine Learning
-
Car Price Prediction Using Machine Learning