Hand Gesture Recognition in python
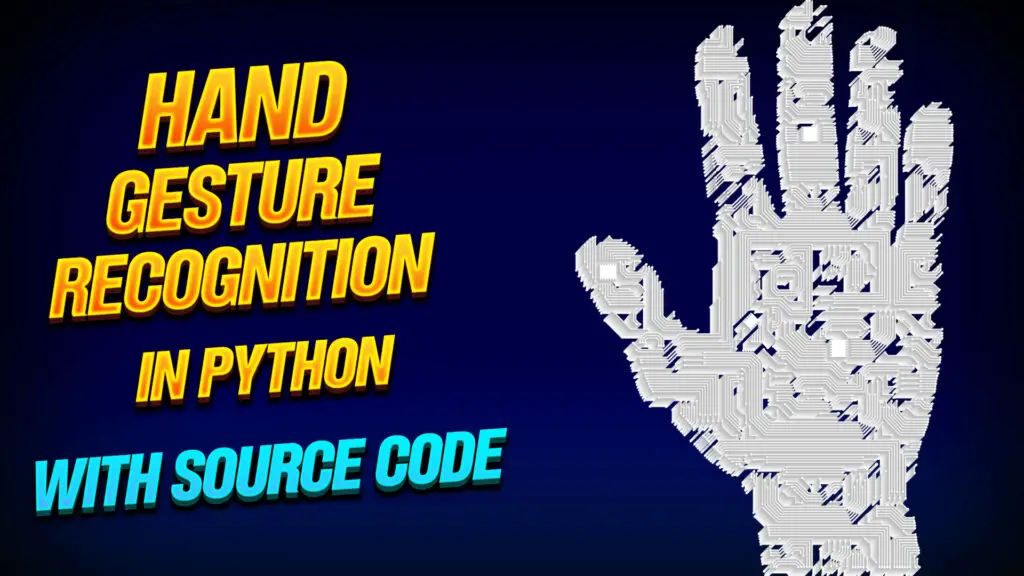
As a final year project, you can develop a gesture recognition interface that will help you enable direct communication between humans and machines. Traditional methods, such as keyboards and mice, can limit communication between humans and machines. So, this project used machine learning techniques and infrared information to detect and classify hand gestures. The system consists of several stages: hand detection, gesture segmentation, feature extraction, gesture classification using five pre-trained convolutional neural network models and vision transformer, and human-machine interface design. To build this system, you can use several machine learning algorithms like support vector machine, K nearest neighbor, decision tree, and neural networks to recognize gestures. This article also provides source code to make this system easy to use.
The Basics of Hand Gestures
Let’s learn some simple things about hand gestures first. We use our hands to show many things by moving them, making different shapes, and putting them in different places. Machines can understand what we want to do by looking at these gestures.
Applications of Hand Gesture Recognition
There are many different and amazing things you can do with hand gestures recognition system. You can make your computer or smart home things do what you want by just moving your hand. Hand gestures can also help people get better after being sick or hurt, and make cars more fun and easy to use.
Hand Gesture Recognition in python
To make Hand Gesture Recognition in python follow these steps.
Step 1: Install Required libraries
Install following libraries by typing these commands in terminal
pip install opencv-python
pip install mediapipe
Step 2: Import required libraries
Create a new python file in VS Code and start typing this code
import cv2
import mediapipe as mp
Step 3: Initialize MediaPipe Hands module
mp_drawing = mp.solutions.drawing_utils
mp_hands = mp.solutions.hands
Step 4: Set up webcam capture:
cap = cv2.VideoCapture(0)
Step 5: Create a loop for real-time processing
with mp_hands.Hands(
min_detection_confidence=0.5,
min_tracking_confidence=0.5) as hands:
Step 6: Inside the loop, read frames from the webcam
success, image = cap.read()
Step 7: Convert and flip the image:
image = cv2.cvtColor(cv2.flip(image, 1), cv2.COLOR_BGR2RGB)
Step 8: Process the image using MediaPipe Hands
results = hands.process(image)
Step 9: Draw hand landmarks on the image
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
mp_drawing.draw_landmarks(
image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
Step 10: Display the annotated image:
cv2.imshow('MediaPipe Hands', image)
Step 11: Break the loop if the 'Esc' key is pressed
if cv2.waitKey(5) & 0xFF == 27:
break
Step 12: Release the webcam and close the window when done
cap.release()
Output
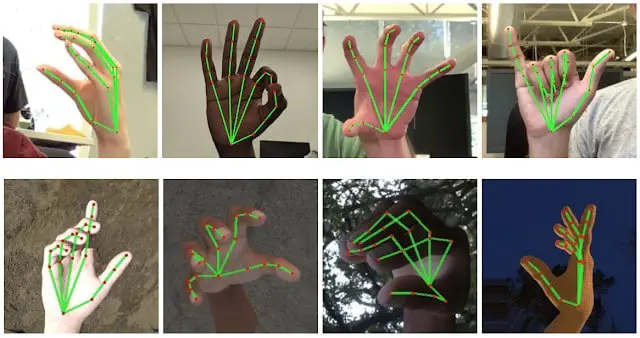
To expand your knowledge, check out these links:
You did it! You learned a lot about hand gesture recognition in Python. You learned some simple things and also how to make it work in real time. You know a lot about this cool technology that can do amazing things. When you work on your own things, don’t forget that you can do anything you want.
Absolutely! Hand gesture recognition is widely used in virtual reality to enhance user interaction and create immersive experiences.
While it may seem complex at first, with the right resources and dedication, beginners can grasp the fundamentals and gradually master the techniques.
Hand gesture recognition is valuable in healthcare for applications such as rehabilitation exercises, where patients can interact with virtual environments to aid in their recovery.
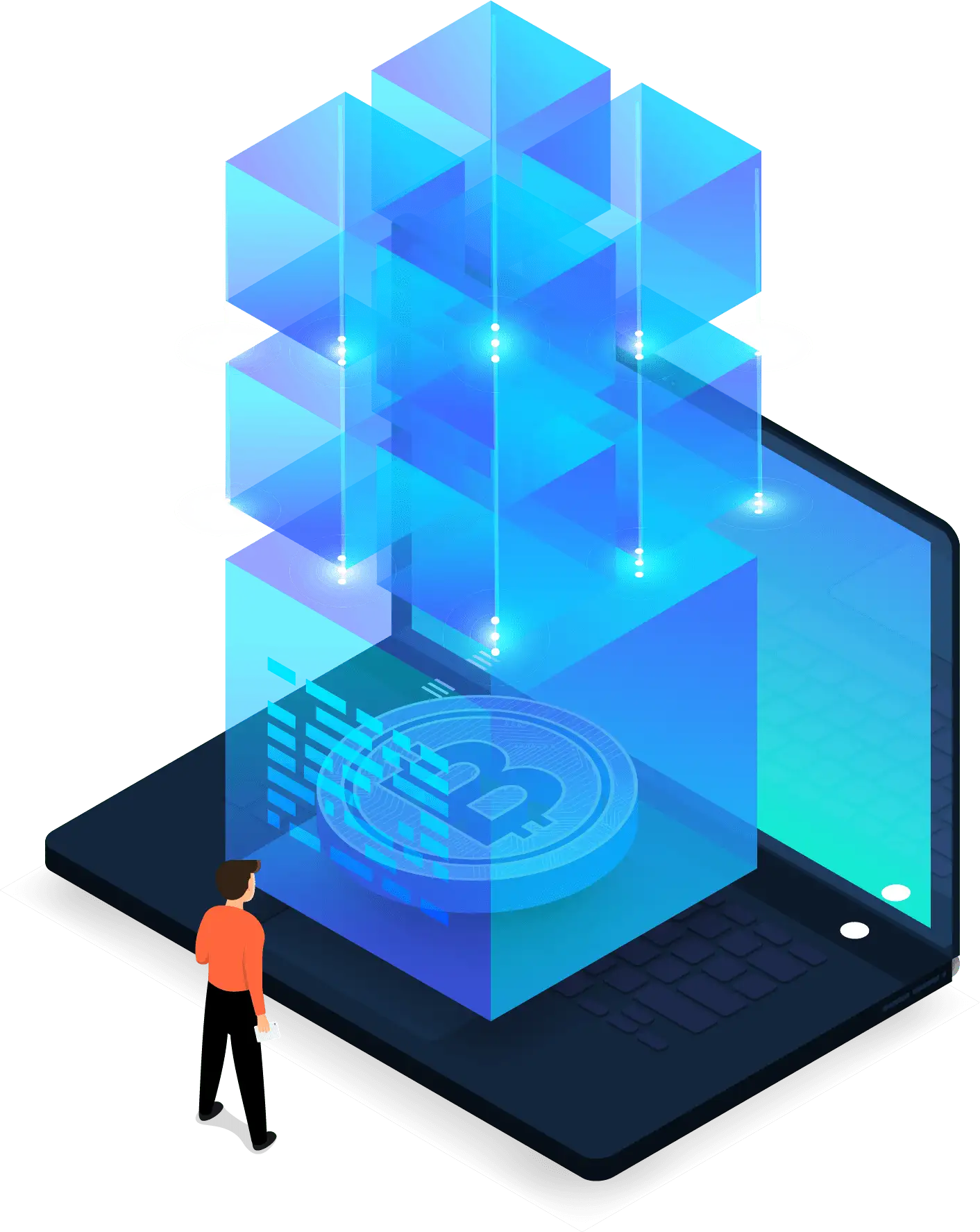
Blockchain Projects
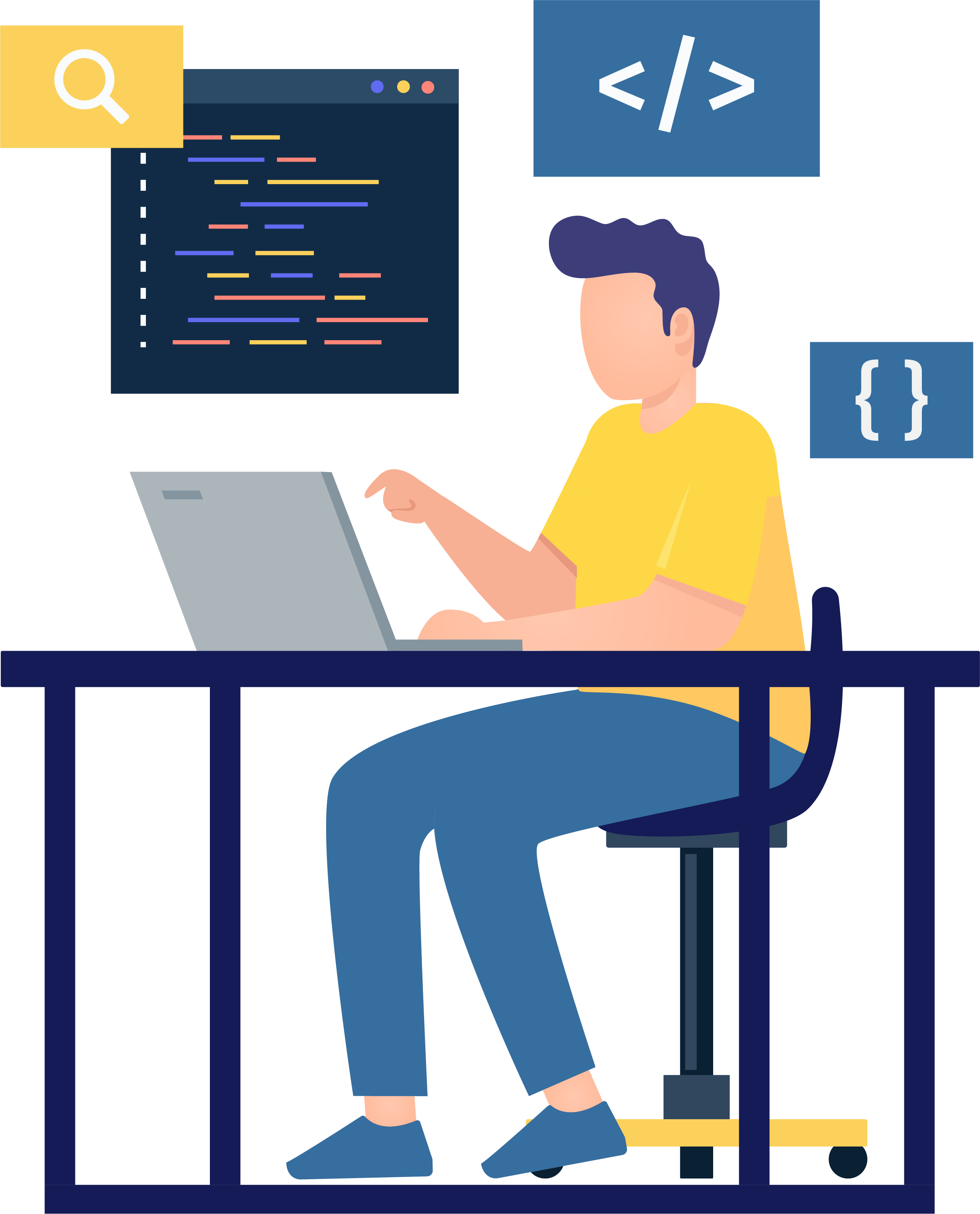
Python Projects
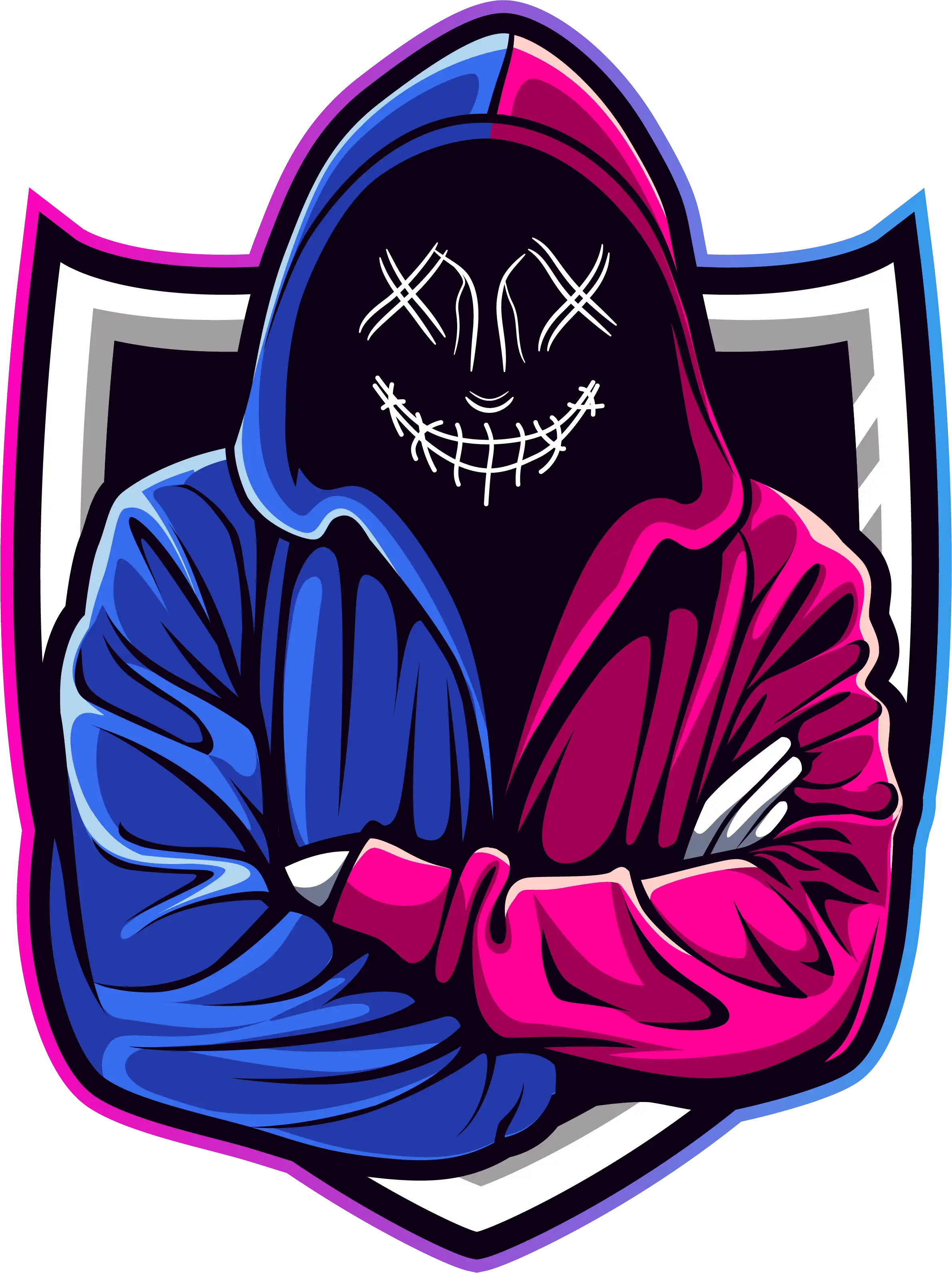
Cyber Security Projects
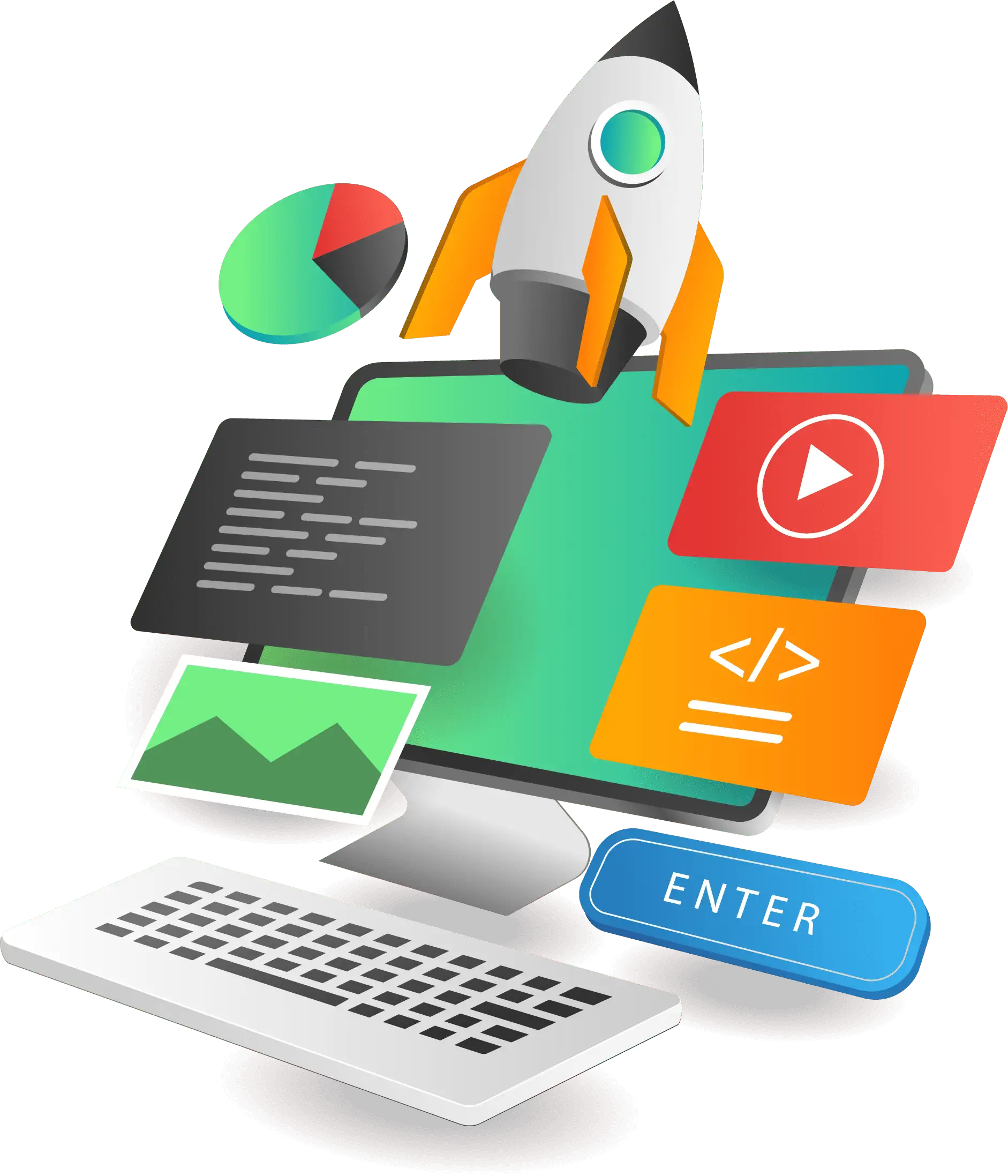
Web dev Projects
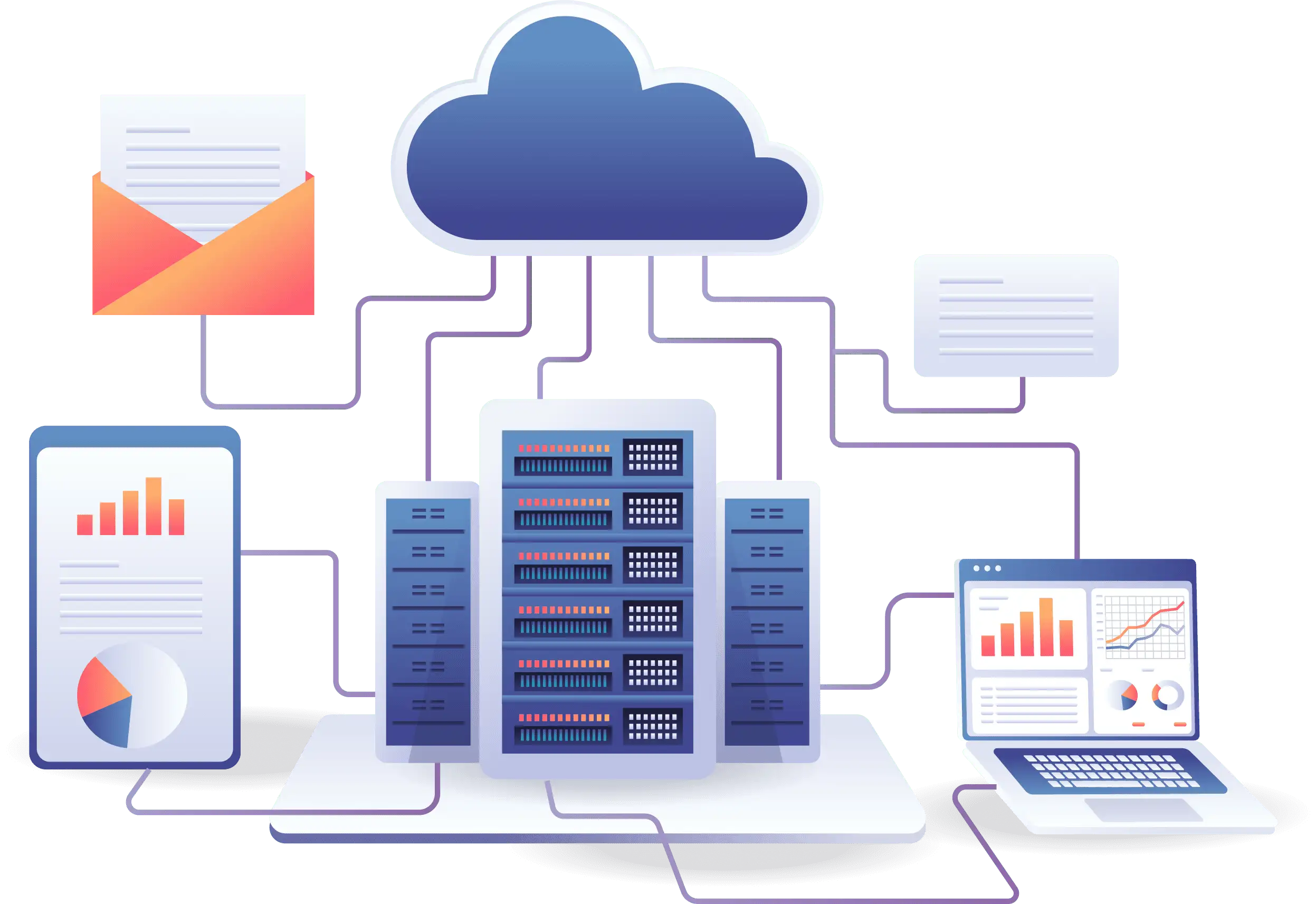
IOT Projects
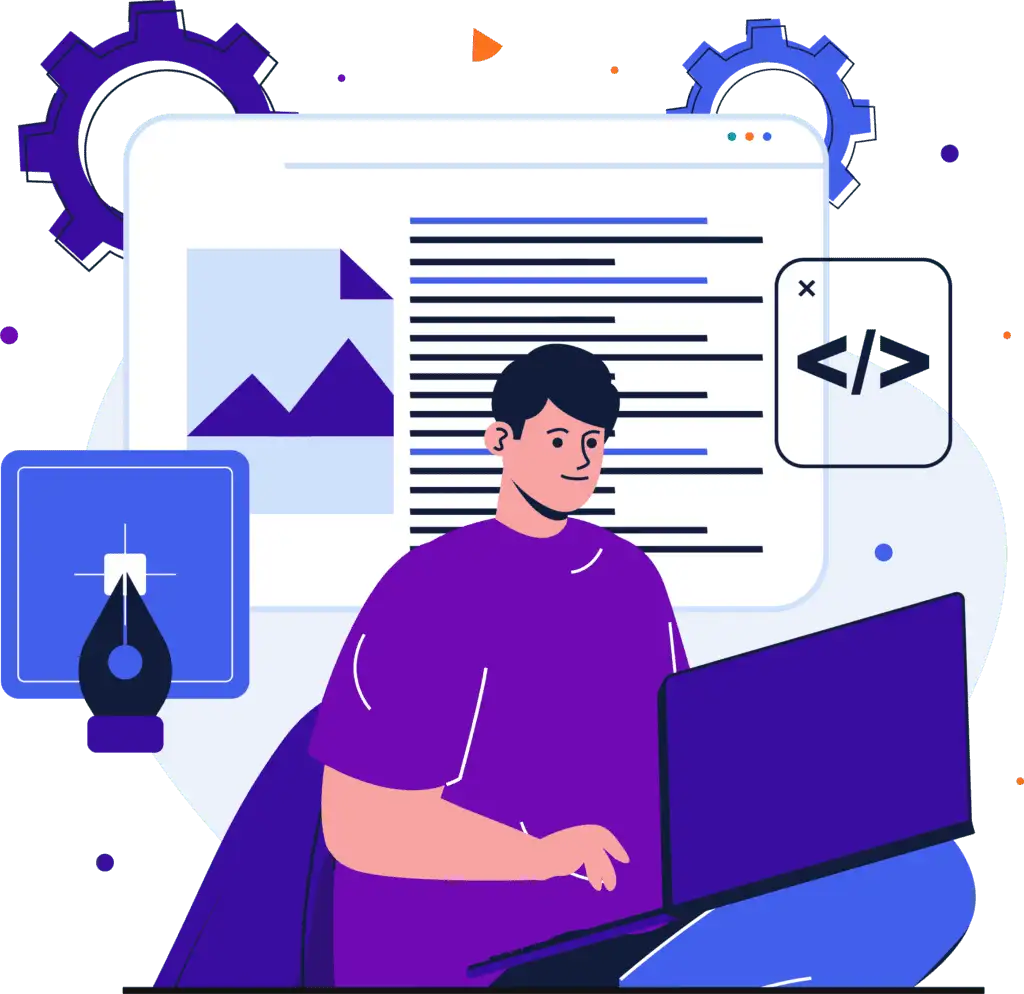
C++ Projects
-
Top 20 Machine Learning Project Ideas for Final Years with Code
-
10 Deep Learning Projects for Final Year in 2024
-
10 Advance Final Year Project Ideas with Source Code
-
Realtime Object Detection
-
AI Music Composer project with source code
-
E Commerce sales forecasting using machine learning
-
Stock market Price Prediction using machine learning
-
30 Final Year Project Ideas for IT Students
-
c++ Projects for beginners
-
Python Projects For Final Year Students With Source Code
-
20 Exiciting Cyber Security Final Year Projects
-
Top 10 Best JAVA Final Year Projects
-
C++ Projects with Source Code
-
Artificial Intelligence Projects For Final Year
-
10 Web Development Projects for beginners
-
How to Download image in HTML
-
Fake news detection using machine learning source code
-
How to Host HTML website for free?
-
Hate Speech Detection Using Machine Learning
-
Credit Card Fraud detection using machine learning
-
15 Exciting Blockchain Project Ideas with Source Code
-
Hand Gesture Recognition in python
-
10 advanced JavaScript project ideas for experts in 2024
-
Best Machine Learning Final Year Project
-
Best 21 Projects Using HTML, CSS, Javascript With Source Code
-
Data Science Projects with Source Code
-
Ethical Hacking Projects
-
Plant Disease Detection using Machine Learning
-
20 Advance IOT Projects For Final Year in 2024
-
Artificial Intelligence Projects for the Final Year
-
Python Projects For Beginners with Source Code
-
Top 7 Cybersecurity Final Year Projects in 2024
-
Phishing website detection using Machine Learning with Source Code
-
portfolio website using javascript
-
17 Easy Blockchain Projects For Beginners
-
Top 13 IOT Projects With Source Code
-
Best 13 IOT Project Ideas For Final Year Students
-
Fabric Defect Detection
-
10 Exciting Next.jS Project Ideas
-
Heart Disease Prediction Using Machine Learning
-
How to Change Color of Text in JavaScript
-
Why Creators Choose YouTube: Exploring the Four Key Reasons
-
10 Exciting C++ projects with source code in 2024
-
Wine Quality Prediction Using Machine Learning
-
10 Final Year Projects For Computer Science With Source Code
-
Diabetes Prediction Using Machine Learning
-
10 TypeScript Projects With Source Code
-
Maize Leaf Disease Detection
-
Car Price Prediction Using Machine Learning
-
Chronic Kidney Disease Prediction Using Machine Learning